Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial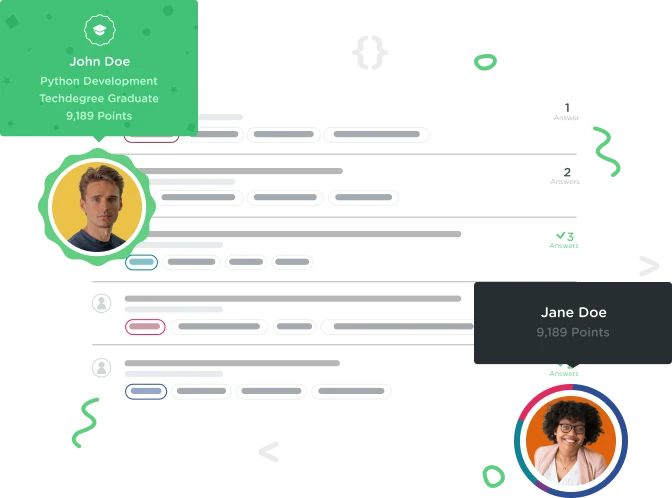

VICTORIA DENNIS
Full Stack JavaScript Techdegree Student 3,679 Pointsstatus === null ; why doesn't it stop the function when I don't submit anything?
const tMinus = 10;
let to = 'Liftoff! 🚀';
for ( let i = tMinus; i >= 1; i-- ) {
let status = prompt(`T-Minus ${i}... Continue? (Y/N)`);
if ( status === null || status.toLowerCase() === 'n' ) {
to = 'Abort launch!';
break;
}
}
alert(to);
In the if ( status === null || status.toLowerCase() === 'n' )
line, the countdown continues even if no letter is input, and also if pure jibberish is input. I suppose there needs to be an if loop for the jibberish part. But why does the loop still run if nothing is put in? Perhaps, I need a better definition for null. I thought that meant nothing is put in or 0. The only time it aborts is with an n
or I hit escape or cancel.
4 Answers
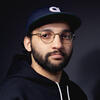
Thomas Davidson
7,243 PointsI'm not 100% certain but my understanding is as follows...
- null is an empty or non-existent value.
- null must be assigned.
By not declaring a value to a variable, JavaScript automatically assigns the value to undefined
.
JavaScript will never automatically assign the value to null
. That must be done by you in your code.
However, when you assign null
to a variable, you are declaring that this value is explicitly empty.
So leaving the prompt blank does not result in null
being the value, because you haven't explicitly stated it to be null
.
It instead becomes either undefined
or possibly just an empty string?
Also..
null === undefined // false
null == undefined // true
null === null // true
"" == null // false
"" == undefined // false

J A
Full Stack JavaScript Techdegree Student 4,646 PointsGood question. I was wondering what the purpose of null
here is as well. Here's what I found out:
If you hit Enter or click OK on the prompt dialog box, the returned value will always be a string. Even when you don't enter anything, the value would be an empty string ""
, which is not the same as null
and that's why the countdown continues. You can adjust the if
condition to include a check for an empty string, if you want that to Abort the Launch as well.
The prompt()
function returns null
when a user clicks Cancel on the dialog box (instead of OK). If you cancel the prompt when asked, the status
variable should be null
at that point and it will Abort the launch.
I learned this from the MDN documentation for the prompt()
function.
https://developer.mozilla.org/en-US/docs/Web/API/Window/prompt

Roxanne Reyes
9,006 PointsI think break tells the program to stop.

babyoscar
12,930 PointsAn empty prompt response actually returns ""
(an empty string), so if you change null
to ""
in your if
statement, it should work. Hope this helped!
Ha Yeong Jeong
4,940 PointsHa Yeong Jeong
4,940 Pointsconst tMinus = 3; let message = 'Liftoff! 🚀';
for ( let i = tMinus; i >= 1; i-- ) { let status = prompt(
T-Minus ${i}... Continue? (Y/N)
); if ( status === null || status.toLowerCase() !== 'y' ) { message = 'Abort launch!'; break; } }alert(message);
Try the above code. It seems that "null" is activated when you press the "Cancel" button.