Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial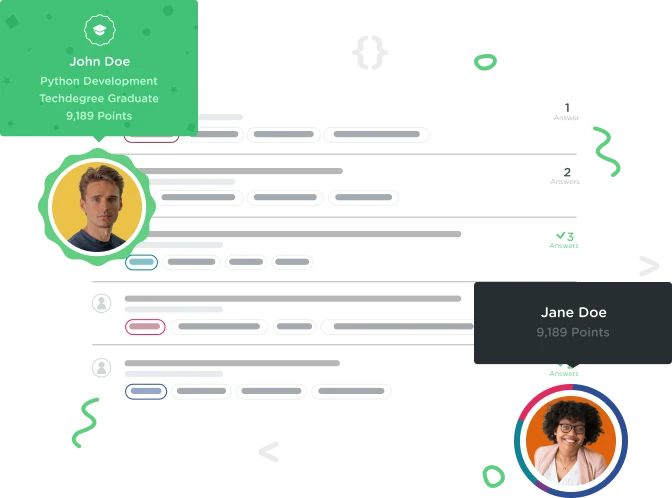

justin z
11,042 PointsStep 2 of "Parcel All the Things"
I get the following error when I run my code.: ./TimeTravelActivity.java:15: error: incompatible types: Parcelable cannot be converted to EnergySource EnergySource source = intent.getParcelableExtra("EXTRA_ENERGY"); ^ For some reason it is not returning a EnergySource object even though as you can see in my code, I passed in the EnergySource variable with my intent. Where did i go wrong?
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
public class MainActivity extends Activity {
public Button goButton;
public EditText yearField;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
goButton = (Button)findViewById(R.id.goButton);
yearField = (EditText)findViewById(R.id.yearField);
final EnergySource source = new EnergySource();
goButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// Add your code in here!
Intent intent = new Intent(MainActivity.this, TimeTravelActivity.class);
intent.putExtra("EXTRA_YEAR", yearField.getText().toString());
intent.putExtra("EXTRA_ENERGY",source);
startActivity(intent);
}
});
}
}
import android.os.Bundle;
import android.view.View;
public class TimeTravelActivity extends Activity {
public String targetYear;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_time_travel);
Intent intent = getIntent();
targetYear = intent.getStringExtra("EXTRA_YEAR");
EnergySource source = intent.getParcelableExtra("EXTRA_ENERGY");
}
}
1 Answer
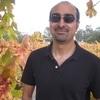
Kourosh Raeen
23,733 PointsHi Justin - You need to cast it to EnergySource .
EnergySource source = (EnergySource) intent.getParcelableExtra("EXTRA_ENERGY");
justin z
11,042 Pointsjustin z
11,042 PointsThank you Kourosh! I can't believe I missed this!
Kourosh Raeen
23,733 PointsKourosh Raeen
23,733 PointsIt happens to us all
. Happy coding!
Jeff Vig
16,345 PointsJeff Vig
16,345 PointsI don't understand why the cast is required. In the video, putting the parcelable data into Song worked without a cast. And when I put a cast on it, it says it is redundant.
Can you explain?