Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial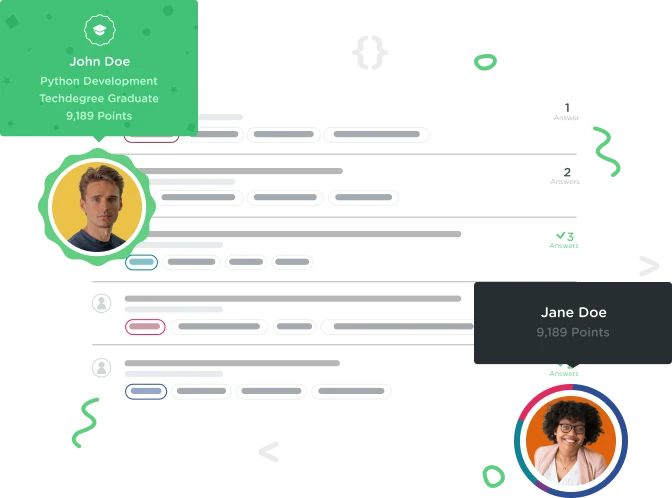
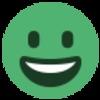
Jack Cummins
17,417 Points@stevehunter Please help me with this.
Steve Hunter please help me
In the normalizeDiscountCode verify that only letters or the $ character are used. If any other character is used, throw a IllegalArgumentException with the message Invalid discount code.
public class Order {
private String itemName;
private int priceInCents;
private String discountCode;
public void applyDiscountCode(String discountCode) {
this.discountCode = normalizeDiscountCode(discountCode);
}
private String normalizeDiscountCode(String letter){
this.discountCode = letter;
return this.discountCode.toUpperCase();
}
public Order(String itemName, int priceInCents) {
this.itemName = itemName;
this.priceInCents = priceInCents;
}
public String getItemName() {
return itemName;
}
public int getPriceInCents() {
return priceInCents;
}
public String getDiscountCode() {
return discountCode;
}
}
2 Answers
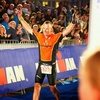
Steve Hunter
57,712 PointsOnly just saw this; sorry, Jack Cummins.
You've got an answer, so you're good to go! However, there's a slightly more elegant way of testing if a char
is a letter. The Character
class has a class helper method called isLetter()
which returns true or false depending whether the passed-in char
is a letter. To use a class method, chain it to the class itself using dot notation.
So, you end up with something like:
private String normalizeDiscountCode(String discountCode){
for(char letter : discountCode.toCharArray()){
if(!Character.isLetter(letter) && letter != '$'){
throw new IllegalArgumentException("Invalid discount code");
}
}
return discountCode.toUpperCase();
}
There's an issue with the other solution as it requires the discountCode
to be made up of upper case letters. Testing (c >= 'A' && c <= 'Z')
doesn't help with lowercase letters. You could do this:
(Character.toUpperCase(c) >= 'A' && Character.toUpperCase(c) <= 'Z')
But that's getting ugly - I'd recommend the use of the .isLetter()
method for its better handling of cases, and overall clarity.
I hope that makes sense.
Steve.

ianuweocrs
6,958 PointsHi ! In the normalizeDiscountCode, you have to check whether it countains something else than a letter or '$'. You can simply do it by iterating over all the characters of the String given in parameter, like this :
for (char c: discountCode.toCharArray())
if ( !((c >= 'A' && c <= 'Z') || c == '$') )
throw new IllegalArgumentException("Invalid discount code");
I let you implement the rest of the code
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsYou could send the
discountCode
to upper case prior to the testing to alleviate this issue. But the code still lacks the clarity of using the helper method:Jack Cummins
17,417 PointsJack Cummins
17,417 PointsSteve Hunter Thank You for Helping Me! By the way, who's that person on your profile picture??
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsThe person on my profile picture, amazingly, is me!
Jack Cummins
17,417 PointsJack Cummins
17,417 PointsSo what did you do?!
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsIt's a triathlon called an Ironman. The photo is of me crossing the finish line in Kalmar, Sweden in August 2014. An Ironman is a long distance triathlon consisting of a 2.4 mile sea swim, a 112 mile bike ride and then a 26.2 mile run, all done consecutively with no breaks. Tough day out - 140.6 miles across the three disciplines. I highly recommend trying one; such a great feeling when you finish!
Jack Cummins
17,417 PointsJack Cummins
17,417 PointsAwesome!
Jack Cummins
17,417 PointsJack Cummins
17,417 PointsSteve Hunter
57,712 PointsSteve Hunter
57,712 PointsHi!
Jack Cummins
17,417 PointsJack Cummins
17,417 PointsSteve Hunter
57,712 PointsSteve Hunter
57,712 PointsWe've got Twitter or Facebook for this!
Jack Cummins
17,417 PointsJack Cummins
17,417 Points