Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial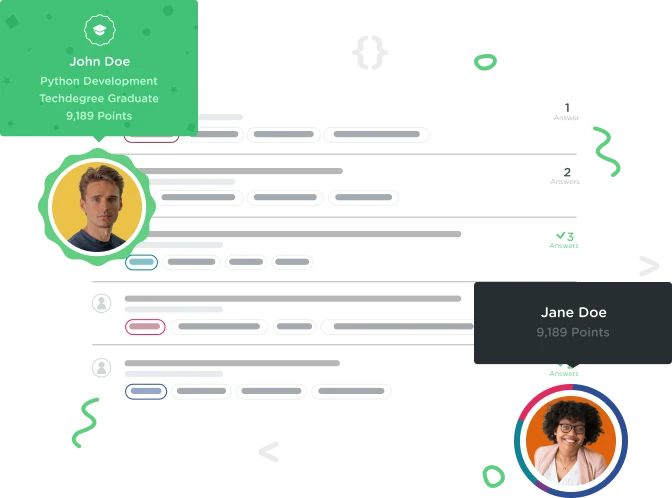

Delicia Wharton
UX Design Techdegree Student 11,098 PointsStill can't get adjacent buttons to work (btn + btn)
**TLDR Guil always makes things seem complicated for me versus Nick Petit and I always struggle with Guil's CSS lessons. Please help me! My buttons never displayed online at the media query (previous video) and in this video, I can't add padding or whatever properly for adjacent button spacing if they were never inline in the first place. I am sure I don't have typos but maybe I cannot see them.
(1 of 2) CSS
/* Attribute Selectors ------------- */
/* KEEP IT SIMPLE */
/*
[class] {
color: red;
}
^^ every attribute that is "class" is selected when brackets are used like this
*/
/* div[id="container"] targets all div elements that have their id as container
div[id="container"] {
max-width: 500px;
margin: auto;
}
*/
/* HOWEVER, BELOW IS THE BEST WAY (SELECTING CLASSES INSTEAD OF ELEMENTS) UNLESS IT IS THE INPUT ELEMENT (BECAUSE THE INPUT ELEMENT HAS SEVERAL ATTRIBUTES WHICH SPECIFY IT vv */
.form-contact {
padding: 24px;
background: #F4F7F8;
}
#container {
max-width: 500px;
margin: auto;
}
/* INPUT + placeholder attribute in general will have yellow background */
input[placeholder] {
background: #fdfee6;
}
/* there is more than one way, see below (same specificity because the same placeholder input attribute, also has the type=email attribute)
input[type="email"] {
background: #fdfee6;
}
*/
/* INPUT + all types that are text will be black background */
input[type="text"] {
background: #000;
}
/* display pointer cursor when user hovers over RADIO BUTTONS (action buttons)
input[type="button"],
input[type="reset"],
input[type="submit"] {
cursor: pointer;
}
^^ this is the long way, there is a shorter way (DRY CSS METHOD) directly below, it replaces a list of input types with a class they all share to make the code shorter
.btn {
cursor: pointer;
}
*/
/* the blow target all links that open in a new window or tab such as target="_blank" from HTML */
a[target="_blank"] {
color: #39add1;
text-decoration: none;
border-bottom: 1px dotted;
}
/* --------------------- DRY CLASSES --------------------- */
.br {
border-radius: .5em;
}
.avatar {
display: block;
margin: 0 auto 2em;
}
.rounded {
border-radius: 50%;
}
/* ^^ centered and rounded avatar element above the sign up section */
.btn {
cursor: pointer;
font-size: .875em;
font-weight: 400;
color: #fff;
padding-left: 20px;
padding-right: 20px;
text-transform: uppercase;
}
.btn:hover {
opacity: .75;
}
.default {
background-color: #52bab3;
}
/* ^^ in reference to <input class="btn default"> from HTML doc */
.error {
background-color: #ff784f;
/* ^^ for the reset button */
@media (min-width: 769px) {
.inln {
width: auto;
display: inline-block;
}
.btn + .btn {
margin-left: 20px;
}
/* ^^ whenever buttons are next to eachother */
}
/* ^^ in reference to
<input class="btn inln default" type="submit" value="Submit">
<input class="btn inln error" type="reset" value="Reset">
from HTML */
/* --------------------- COMBINATORS --------------------- */
/* Children are directly nested within their parent elements by 1 indentation / 1 specificity level */
form > a {
font-size: .7em;
}
h1 ~ label {
background: tomato;
color: white;
padding: 5px;
}
/* ^^ targeting all sibling label element that follow an h1 element */
(2 of 2) HTML
<!DOCTYPE html>
<html>
<head>
<!-- This attribute selector targets any element that has a class attribute:
[class] {
border: solid 1px #ccc;
}
To target an input element with a type value of submit, we write:
input[type="submit"] {
background-color: green;
}
This targets a elements with a target value of _blank:
a[target="_blank"] {
color: tomato;
} -->
<title>Selectors</title>
<meta name="viewport" content="width=device-width, initial-scale=1">
<link href='http://fonts.googleapis.com/css?family=Nunito:400,300' rel='stylesheet' type='text/css'>
<link rel="stylesheet" href="css/base-style.css">
<link rel="stylesheet" href="css/selectors.css">
</head>
<body>
<div id="container">
<form class="form-contact br">
<h1>Contact</h1>
<label for="name">Name:</label>
<input type="text" id="name">
<label for="email">Email:</label>
<input type="email" id="email" placeholder="email@website.com">
<label for="msg">Message:</label>
<textarea id="msg" rows="7"></textarea>
<input class="btn inln default" type="submit" value="Submit">
<input class="btn inln error" type="reset" value="Reset">
</form>
<hr>
<img class="avatar rounded" src="img/avatar.png" alt="Mountains">
<form class="form-login">
<label for="username">Username:</label>
<input type="text" id="username">
<label for="password">Password:</label>
<input type="password" id="password">
<input class="btn default" type="submit" value="Login">
<a href="#" target="_blank">Forgot your password?</a>
</form>
</div>
</body>
</html>
**TLDR Guil kind of makes things complicated for me versus Nick Petit and I always struggle with his CSS lessons. I cannot get the buttons to display inline (whether I use .7em or not for the Please help me!
Mod Edit: Fixed code formatting. See Steven Parker 's comment below on how to do this.
2 Answers
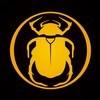
rydavim
18,813 PointsIt looks like your issue might just be a typo. If I copy-paste your code, it's on line 56 for me. You've got a style with no closing bracket.
.error {
background-color: #ff784f;
} /* I added this, and your buttons displayed inline for me. */
Let me know if that doesn't resolve it, or if you have follow up questions. Happy coding!

Delicia Wharton
UX Design Techdegree Student 11,098 PointsThank you I’ll try that ☺️

Delicia Wharton
UX Design Techdegree Student 11,098 PointsI really appreciate the help, I'm not sure why I've made so many typos. I'm a bit embarrassed.
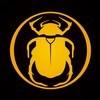
rydavim
18,813 PointsDon't feel embarrassed! Typos are totally normal and everyone makes them. Even experienced programmers are bound to have errors sometimes.
With practice you'll get used to finding them yourself based on the errors you get, but even then it's often much easier for someone else to find simple mistakes like that because they didn't write it. It's the same as having someone proofread a paper - they notice mistakes because they don't have an expectation of what it should be.
So don't get discouraged, and keep on coding! 😃
Steven Parker
229,732 PointsSteven Parker
229,732 PointsWhen posting code, use Markdown formatting to preserve the code's appearance and prevent certain characters from being consumed. An even better way to share code and make your issue easy to replicate is to make a snapshot of your workspace and post the link to it here.