Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial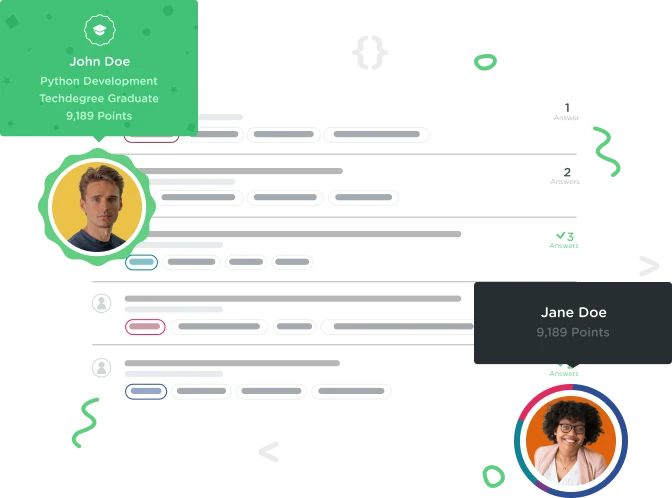

Matt Conway
1,572 PointsStill confused about while, loops
Trying to figure out how to do it using right order and codes ...
Challenge Task 2 of 2
Now that we have the while loop set up, it's time to compute the sum! Using the value of counter as an index value, retrieve each value from the array and add it to the value of sum.
For example: sum = sum + newValue. Or you could use the compound addition operator sum += newValue where newValue is the value retrieved from the array.
/Users/mattconway/Desktop/Screen Shot 2016-01-13 at 6.50.22 PM.png
let numbers = [2,8,1,16,4,3,9]
var sum = 0
var counter = 0
// Enter your code below
while counter < numbers.count { counter++ }
var newValue = sum += newValue
while index < newValue.count {
print(newValue[index])
index++
}
2 Answers
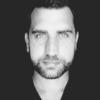
Jhoan Arango
14,575 PointsHello :
A while loop basically means "While this condition is true, then continue until is false".
In this challenge we have an array to iterate through.
let numbers = [2,8,1,16,4,3,9]
So now we have to create a condition to be able to use this while loop.
let numbers = [2,8,1,16,4,3,9]
var sum = 0 // We will use this to add all the numbers inside the array
var counter = 0 // We will use this for the condition, and as the index for the array
Now let's create that loop...
let numbers = [2,8,1,16,4,3,9]
var sum = 0
var counter = 0
// Loop
while counter < numbers.count {
counter++
}
/*
This is saying "While counter is less then the
amount of items in the array then continue,
and add one more to counter"
*/
Now we finish the loop by retrieving an item from the array, and adding it to the sum variable.
let numbers = [2,8,1,16,4,3,9]
var sum = 0
var counter = 0
// Loop
while counter < numbers.count {
sum += numbers[counter]
counter++
}
So basically what we are doing is grabbing one item from the array as the loop goes around the first time, and adding it to the sum variable, then we update the counter by adding to it. So now sum is 1, and this will help us with the next item in the array by using counter as the index. Once the condition becomes false, which it will after we have no more items in the array, the loop will stop.
Hope this helps you understand while loops a bit more.

Reagen Rahardjo
3,770 PointsHi,
I tried to run the program on XCODE but i got this message back Untitled Page[30758] <Error>: CGContextSaveGState: invalid context 0x0. If you want to see the backtrace, please set CG_CONTEXT_SHOW_BACKTRACE environmental variable.
is it an error?