Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial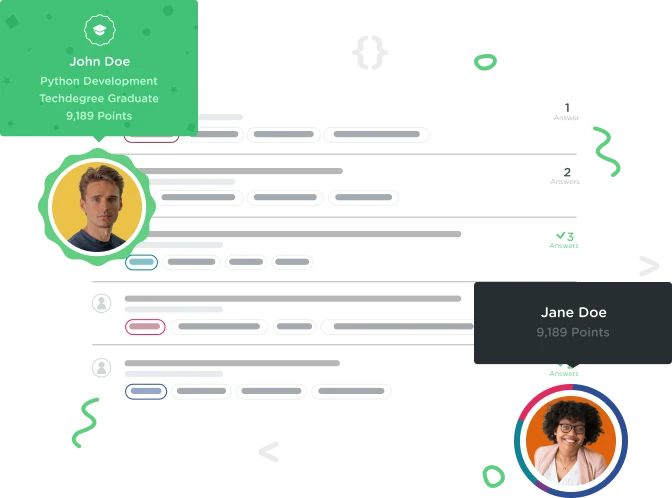
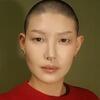
Begana Choi
Courses Plus Student 13,126 PointsStill confused with self and self.value
I'm still confused with self and self.value concept.
I can understand that self.value is just an attribute of self
and self and Numstring itself.
but why don't we use self.value for add method and other methods for add?
class Numstring:
def __init__(self, value):
self.value = str(value)
def __str__(self):
return self.value
def __int__(self):
return int(self.value)
def __float__(self):
return float(self.value)
#from here, why don't we use self.value ??
def __add__(self, other):
if '.' in self.value:
return float(self) + other
return int(self) + other
def __radd__(self, other):
return self + other
def __iadd__(self, other):
self.value = self + other
return self.value
2 Answers
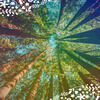
majesticcat123
3,020 PointsBy this point in the code, we have already defined the __float__()
method, so we can just convert the whole object (self
) to a float. It's handier to have separate values for str
versions and int
/float
versions rather than keep converting self.value
. self
is the whole object, and self.value
is the value of self
as a str
datatype. self.value
is an attribute.
Also, sorry, I didn't quite read the code when I answered earlier, I should have said that we can't use self.value
because it's a str
but we can't use self
because it's a Numstring
. We have to convert them.
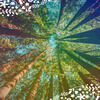
majesticcat123
3,020 PointsIf you look higher up in the code, you see that self.value
is actually an str
datatype.
def __init__(self, value):
self.value = str(value)
If we tried to use self.value
for the arithmetic methods (__add__
, __mul__
, __radd__
etc.), we would get an error, because it would be adding an str
to an int
or float
. So, we convert self.value
to a number datatype (int
or float
) so it is a valid datatype to do maths with. I hope this helps!
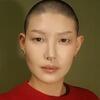
Begana Choi
Courses Plus Student 13,126 Pointsbut then we need to convert them like float(self.value)
not flaot(self)
I'm asking difference between self and self.value :)
Begana Choi
Courses Plus Student 13,126 PointsBegana Choi
Courses Plus Student 13,126 Pointsnow it's very clear!! thank you so much!!!
Alexis Othonos
3,171 PointsAlexis Othonos
3,171 PointsAlso you need to be careful when assigning values to self. So for example in the
__iadd__
method, you will notice he usesself.value
instead ofself
for assignment.If he used
self
for assignment, the object would no longer beNumstring
class type, but would be int or float instead!However, if you try it though, it will still be replaced because there are still a few more errors in the
__iadd__
method:self.value
or else the print function will break after you try something like+=
. Alternatively, change the__str__
method to always return value after casting into string (return str(self.value)
)__iadd__
method should return the object, not the object value.The correct implementation should be more like this: