Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial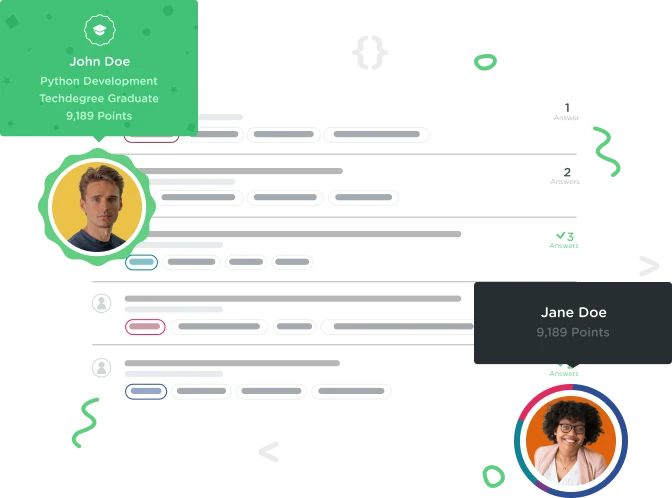

Nhat Anh Dao
8,370 PointsStill don't understand what 'return' is used for ?
Somebody please tell me what is the function of key word 'return' in this method
public boolean applyGuess(char letter){ boolean isHit = mAnswer.indexOf(letter) >=0; if (isHit){ mHits += letter; } else { mMisses += letter; } return isHit; }
I remove the line 'return isHit' and try to run the program, it come out just fine, so, what's the problem if i just cut that line off ?? Will it cause some problems in the future when i try to make more complicated program ? Someone please let me know, thanks you !!
4 Answers
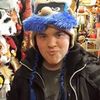
Codin - Codesmite
8,600 PointsI am going to use a different more simplified example to explain return.
Return unlike print, only returns the value, it does not print it to screen, so in some cases you may not see any difference when you remove return.
Here is an example:
public static int add(int a, int b) {
return a + b; // go back to method that called this method (main)
}
public static void main(String[] args) {
int sum = add(10,4); // the return result (14) is stored in sum
}
The above function "add()" will return the value of a + b. In the example above we are declaring the integer sum as the result of the function add(10,4); which would result in 14. If we were to remove "return" from the function so it just had a + b like in the following example.
public static int add(int a, int b) {
a + b; // a + b still equals (14).
}
public static void main(String[] args) {
int sum = add(10,4); // the return result will be NULL because no value was returned from the add function.
}
The function no longer outputs a value, it adds a + b together as normal, but it returns no value at the end of the function.
For example:
public static int add(int a, int b) {
a + b; // a + b still equals (14)
return 11;
}
public static void main(String[] args) {
int sum = add(10,4); // the return result will be (11) as we returned (11) from the function.
}
In the above example "sum" will equal 11.
another example:
public static int add(int a, int b) {
if (a + b > 10) {
return "Sum is more than 10";
} else {
return "Sum is not more than 10";
}
}
public static void main(String[] args) {
String sum = add(10,4); // the return result will be "Sum is more than 10".
}

Sang Tran
6,918 Pointspublic boolean applyGuess(char letter){ boolean isHit = mAnswer.indexOf(letter) >=0; if (isHit){ mHits += letter; } else { mMisses += letter; } return isHit; }
Hello! this is my first time helping someone on this, but to answer your question on the return's purpose in a method. Whenever you create a method and include a type Example. int,String,boolean, double, etc. you must return what you wrote back to your main code so you can use it.
Its like you're creating a promise/pact with your method that whenever you mention a specific type when you create the method you must return it. Unless the method has a void in it then the method just performs an action.
When you call applyGuess() in your main code it will either be true or false, but your main code wont know what it is since you are not returning it.
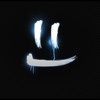
Grigorij Schleifer
10,365 PointsHi Nhat,
You need a method that proofs whether the guess of the User is right or not (or in other words true or false).
This method is applyGuess() and it takes a char "letter" as parameter. This char is the guess of our user. The applyGuess method has a return type. So if you call applyGuess() a value will be returned. This value is a boolean .... true or false. If true, then mAnswer contains the guessed character. If false, the guessed character is not inside the mAnswer.
This line is "creating" the value of the boolean mHit. It can store true or false.
boolean isHit = mAnswer.indexOf(letter) >=0;
If the guessed character (our "letter") is inside of the mAnswer, the indexOf() method returns 1 and 1 is more then 0 ... the expression is true ... and the value stored in isHit is true. If not the value of the mHit is false.
Does it make sense?
Grigorij

Nhat Anh Dao
8,370 Pointsthanks guys, it makes sense to me now