Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial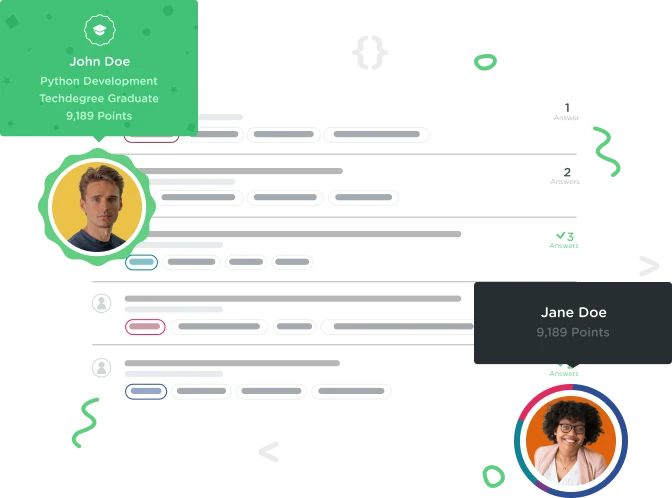
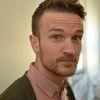
Simon Sporrong
35,097 PointsStill getting "Uncaught TypeError: Cannot read property 'setStates' of undefined"-error.
Even though I've been following along and trying to copy Guils' code I'm still getting the error written above. The console says:
Uncaught TypeError: Cannot read property 'setStates' of undefined at incrementScore (<anonymous>:86:12)
at app.js:57
const players = [
{
name: "Guil",
score: 50,
id: 1
},
{
name: "Treasure",
score: 85,
id: 2
},
{
name: "Ashley",
score: 95,
id: 3
},
{
name: "James",
score: 80,
id: 4
},
{
name: "Simme",
score: 99,
id: 5
}
]
const Header = (props) => {
return (
<header>
<h1>{ props.title }</h1>
<span className="stats">Players: {props.totalPlayers }</span>
</header>
);
}
const Player = (props) => {
return (
<div className="player">
<span className="player-name">
{props.name}
</span>
<Counter score={props.score}/>
</div>
);
}
class Counter extends React.Component {
state = {
score: 0
};
incrementScore = () => {
this.setState( prevState => {
return {
score: prevState.score + 1
};
});
}
decrementScore = () => {
this.setState( prevState => {
return {
score: prevState.score - 1
};
});
}
render() {
return (
<div className="counter">
<button className="counter-action decrement" onClick={this.decrementScore}> - </button>
<span className="counter-score">{ this.state.score }</span>
<button className="counter-action increment" onClick={this.incrementScore}> + </button>
</div>
);
}
}
const App = (props) => {
return (
<div className="scoreboard">
<Header
title="Scoreboard"
totalPlayers={ props.initialPlayers.length } />
{/*players list */}
{props.initialPlayers.map(player =>
<Player
name={player.name}
key={player.id.toString()}/>
)}
</div>
);
}
ReactDOM.render(
<App initialPlayers={players}/>,
document.getElementById('root')
);
Can someone help me please? What am I droing wrong? =)
2 Answers
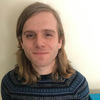
sradms0
Treehouse Project ReviewerYour code seems fine. The error you mention is referring to a 'setStates' function, however, that exact function doesn't show in your code. You are using 'setState' (without the 's' at the end), which is the right function. I just ran this, as well, and no issues. Double check this.
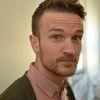
Simon Sporrong
35,097 PointsYes, there was nothing wrong. Turns out i just had a poor internet connection and React didn't load properly (?), or some such. Thanks for taking the time anyway =)
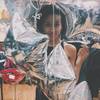
Akosua Kernizan
19,994 PointsIs the error happening when you increment/decrement? If yes then you have to update the code in the onClick listener.
onClick={this.decrementScore}
onClick={this.incrementScore}
becomes
onClick={() => this.decrementScore()}
onClick={() => this.incrementScore()}
The anonymous function binds the state to the functions.
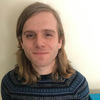
sradms0
Treehouse Project ReviewerYou shouldn't have have to add callbacks in these event handlers in this case. I believe the binding has already been taken care of within the definitions of 'incrementScore' and 'decrementScore' functions, so all that is needed is to just pass those as references.
KRIS NIKOLAISEN
54,971 PointsKRIS NIKOLAISEN
54,971 PointsWhich video/course is this?