Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial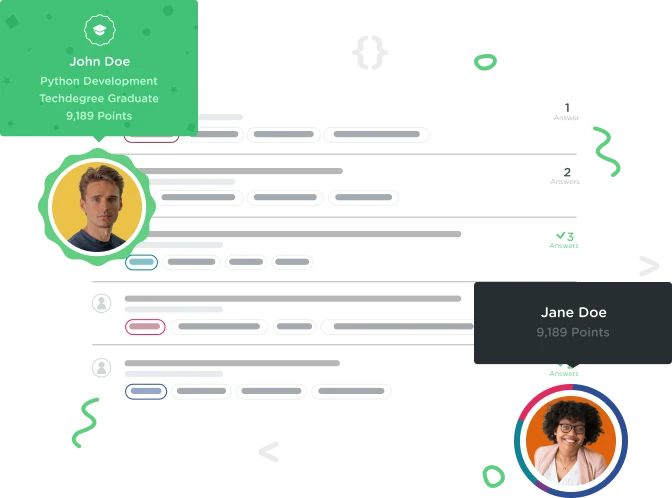
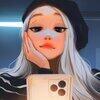
iclick
10,945 PointsStill having problem with the code
Not sure what is wrong with the code. It keeps giving me error with the @override
10 Answers
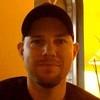
Jeremy Hill
29,567 PointsWell I am glad you finally got it :) I just want to leave you with this final note. I am going to paste a tid bit of code and notice the alignment of the curly braces; it is done this way for programmers to see if they have included them all. If they were scattered around it would make for a long day trying to figure out which one was extra or missing, with that said here is the code:
public class SomeClass{
public void someMethod{
// some code
}
public void someOtherMethod{
// some code
}
}
Notice how the closing braces stay in alignment with their respective methods. This is done to prevent confusion. And any time you get the error: "reached end of file while parsing" this usually means that you have an extra brace or a missing brace- which in this case it was missing. Just a little FYI for ya ;) Happy coding!
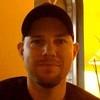
Jeremy Hill
29,567 PointsAnd some times when I know that I will have many methods and if statements I will put comments next to my closing braces so I know what they go to.
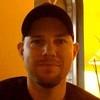
Jeremy Hill
29,567 PointsMake sure that your "Override" is capitalized in your @Override. It is asking you to return the String in the Overridden toString method so it needs to look like this:
@Override
public String toString(){
return "BlogPost: " + this.getTitle() +" by " + this.getAuthor();
}
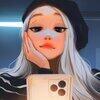
iclick
10,945 PointsOh so sorry. I thought i did post the code. :(
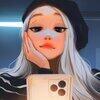
iclick
10,945 PointsHi Jeremy , I did capitalized it but still having compiler error.
./com/example/BlogPost.java:42: error: reached end of file while parsing } ^ 1 error
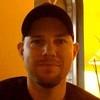
Jeremy Hill
29,567 PointsSounds like you are missing a curly brace somewhere.
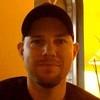
Jeremy Hill
29,567 PointsCheck to make sure that your class has a closing curly brace, as well as all of your methods.
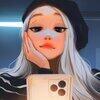
iclick
10,945 PointsJeremy I can't find which one which. That last curly brace pointing that error. :(
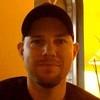
Jeremy Hill
29,567 PointsPaste your formatted code and I will look at it.
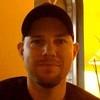
Jeremy Hill
29,567 PointsIt will also throw an error if you have an extra brace- you might need to delete one. If you paste your code I should be able to spot it.
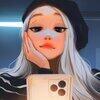
iclick
10,945 Points@Override
public String toString() {
return "BlogPost: " + this.getTitle() +" by " + this.getAuthor();
}
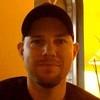
Jeremy Hill
29,567 PointsGo ahead and paste the entire code if you don't mind.
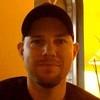
Jeremy Hill
29,567 PointsRight after you type the first three accent marks type the word java; this should format it for the java language.
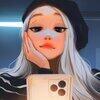
iclick
10,945 Pointspackage com.example;
import java.util.Date;
public class BlogPost { private String mAuthor; private String mTitle; private String mBody; private String mCategory; private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
@Override public String toString(){ return "BlogPost: " + this.getTitle() +" by " + this.getAuthor(); }
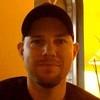
Jeremy Hill
29,567 PointsOkay add one more curly brace at the very bottom; your closing class brace seems to be missing. If this doesn't work then click in the workspace then click ctrl+a to select all of your code then ctrl+v inside your accent marks. This will paste your entire code inside the accent marks and format all of it.
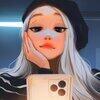
iclick
10,945 PointsJeremy you Rock! It's Working :) Oh My ... You have no idea how frustrated i am. Geeeezzzz
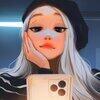
iclick
10,945 PointsAwesome Jeremy! Thanks a bunch. You will hear from me a lot and please don't be annoyed :) You're the best !!!
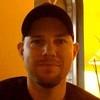
Jeremy Hill
29,567 PointsNot a problem, I enjoy helping out when I can :)
Florian Tönjes
Full Stack JavaScript Techdegree Graduate 50,856 PointsFlorian Tönjes
Full Stack JavaScript Techdegree Graduate 50,856 PointsIrene,
post your code so we can have a look at it.
Regards, Florian