Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial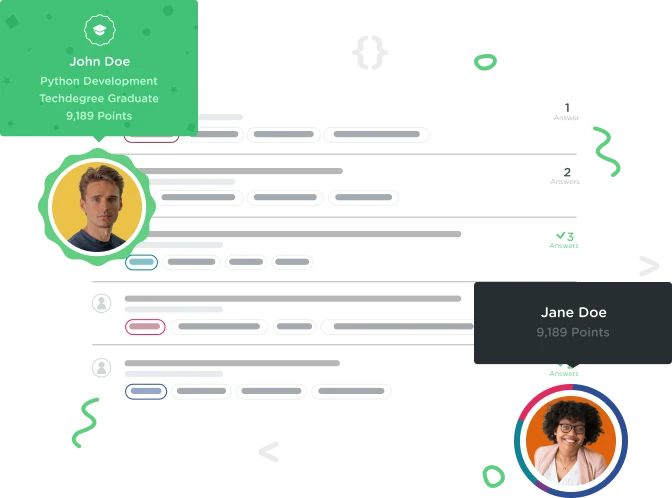

Gary Gibson
5,011 PointsStill not clear on when to add arguments in parentheses.
I thought I understood when to add arguments to a function, but I don't. For example, this works:
def devowel():
word = input("Give me a word to devowel. ")
vowels = 'aeiou'
result = ''
for i in word:
if i not in vowels:
result += i
print(result)
devowel()
But this throws an error:
def devowel(word):
word = input("Give me a word to devowel. ")
vowels = 'aeiou'
result = ''
for i in word:
if i not in vowels:
result += i
print(result)
devowel(word)
This works too, though:
def devowel(word):
word = input("Give me a word to devowel. ")
vowels = 'aeiou'
result = ''
for i in word:
if i not in vowels:
result += i
print(result)
devowel(abomination)
So when the two arguments look different, it's fine.
What's going one?
2 Answers
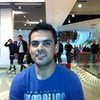
Andreas cormack
Python Web Development Techdegree Graduate 33,011 PointsHi Gary
I ran both your examples and they both throw errors, reason being the argument you are passing is not a string. Hence the error you get is word is not defined or abomination is not defined.
def devowel(word):
word = input("Give me a word to devowel. ")
vowels = 'aeiou'
result = ''
for i in word:
if i not in vowels:
result += i
print(result)
devowel("abomination") # surround either word or abomination within quotes as you need to pass a string.
In terms of passing arguments, You pass arguments to a function to prevent repeating yourself. Imagine if i wanted to add 2 numbers, I would not say num1= 5+5 and then 20 lines down the code say again num3 = 25 +32. That's where functions come in. I could create a function named add and call it every time i need to add two numbers.
see below
def add(a,b):
return a + b
#So when ever i need to add two numbers i call num1=add(5,5) or num3 = add(25,32).
For this example i would not pass arguments as the input function will give you unique inputs from the user every time devowel function is called.
You first example is the best way to write the devowel function
def devowel():
word = input("Give me a word to devowel. ")
vowels = 'aeiou'
result = ''
for i in word:
if i not in vowels:
result += i
print(result)
devowel()
hope this helps

Gary Gibson
5,011 PointsThank you, Andreas. Good illustration.
Gary Gibson
5,011 PointsGary Gibson
5,011 PointsEdit: Got it.
Andreas cormack
Python Web Development Techdegree Graduate 33,011 PointsAndreas cormack
Python Web Development Techdegree Graduate 33,011 Pointswhat you have written is fine. if you still require the user input then see below.