Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial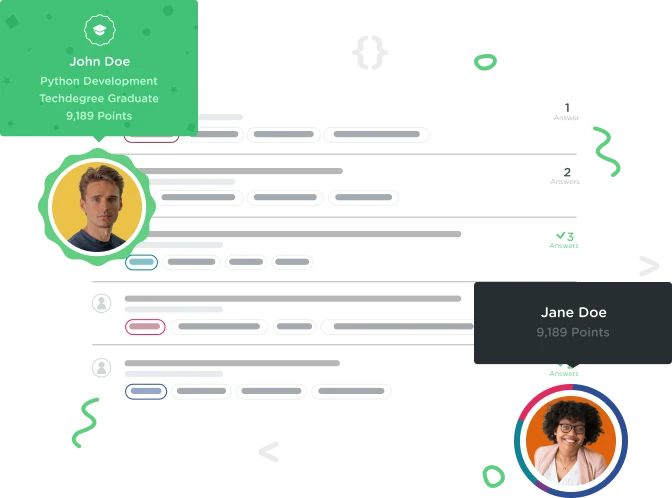
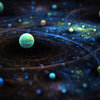
Joseph Martinez
5,482 PointsStill receiving errors when testing, after adding validations
My create_spec.rb file :
require 'spec_helper'
describe "Creating todo lists" do
it "redirects to the todo list index page on success" do
visit "/todo_lists"
click_link "New Todo list"
expect(page).to have_content("New todo_list")
fill_in "Title", with: "My todo list"
fill_in "Description", with: "This is what I'm doing today."
click_button "Create Todo list"
expect(page).to have_content ("My todo list")
end
it "displays an error when the todo list has no title" do
expect (TodoList.count).to eq(0)
visit "/todo_lists"
click_link "New Todo list"
expect(page).to have_content("New todo_list")
fill_in "Title", with:""
fill_in "Description", with: "This is what I'm doing today."
click_button "Create Todo list"
expect(page).to have_content("error")
expect (TodoList.count).to eq(0)
visit "/todo_lists"
expect(page).to_not have_content("This is what I'm doing today")
end
it "displays an error when the todo list has a title less than three characters" do
expect (TodoList.count).to eq(0)
visit "/todo_lists"
click_link "New Todo list"
expect(page).to have_content("New todo_list")
fill_in "Title", with:"Hi"
fill_in "Description", with: "This is what I'm doing today."
click_button "Create Todo list"
expect(page).to have_content("error")
expect (TodoList.count).to eq(0)
visit "/todo_lists"
expect(page).to_not have_content("This is what I'm doing today")
end
it "displays an error when the todo list has no description" do
expect (TodoList.count).to eq(0)
visit "/todo_lists"
click_link "New Todo list"
expect(page).to have_content("New todo_list")
fill_in "Title", with:"HiHiHi"
fill_in "Description", with: ""
click_button "Create Todo list"
expect(page).to have_content("error")
expect (TodoList.count).to eq(0)
visit "/todo_lists"
expect(page).to_not have_content("HiHiHi")
end
it "displays an error when the todo list has a description shorter than 6 characters" do
expect (TodoList.count).to eq(0)
visit "/todo_lists"
click_link "New Todo list"
expect(page).to have_content("New todo_list")
fill_in "Title", with:"HiHiHi"
fill_in "Description", with: "Bone"
click_button "Create Todo list"
expect(page).to have_content("error")
expect (TodoList.count).to eq(0)
visit "/todo_lists"
expect(page).to_not have_content("HiHiHi")
end
end
my todo_list.rb file:
class TodoList < ActiveRecord::Base
validates :title, presence: true
validates :title, length: { minimum: 3 }
validates :description, presence: true
validates :description, length: {minimum: 6}
end
when I open the local host and manually perform the tests the expected errors pop up. However, when I run the rspec test I am getting 5 examples, 4 failures... which leads me to believe that the problem is the way I have written the create_spec.rb file I just can't seem to find where I have made the mistake
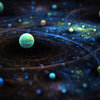
Joseph Martinez
5,482 Pointshere are the failure messages when running rspec using the tests built in the lesson:
1)creating todo lists displays an error when the todo list has a description shorter than 6 characters
Failure/Error: expect <TodoList.count>.to eq<0>
NoMethodError:
undefined method 'to' for 0:Fixnum
# ./spec/features/todo_lists/create_spec.rb:74:in 'block <2 levels> in <top<required>>'
The remaining error messages are exactly the same, except they cite the other lines in the create_spec.rb code in which the following appears :
expect (TodoList.count).to eq(0)
To clarify: In actual practice the ruby app does throw the appropriate error messages when inadequate user input is recieved. However, the tests to ensure that these error messages are working is failing, and they should be passing. The line of code that seems to be the problem is:
expect(TodoList.count).to eq(0)
which checks to make sure that no Todo List was made if inadequate input was given. However I could be mistaken about exactly the problem is
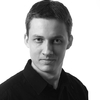
Maciej Czuchnowski
36,441 PointsDid you put space after the expect
keyword here, as quoted?
expect (TodoList.count).to eq(0)
If so, remove it and see what errors pop up, if any. That's something to start with.
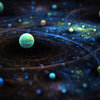
Joseph Martinez
5,482 PointsAh yes, that was the problem, I knew it was probably syntax error but I just couldn't figure out what. Thanks a lot for your help.
Maciej Czuchnowski
36,441 PointsMaciej Czuchnowski
36,441 PointsYou'd have to give us the failure messages, they will tell us everything.