Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial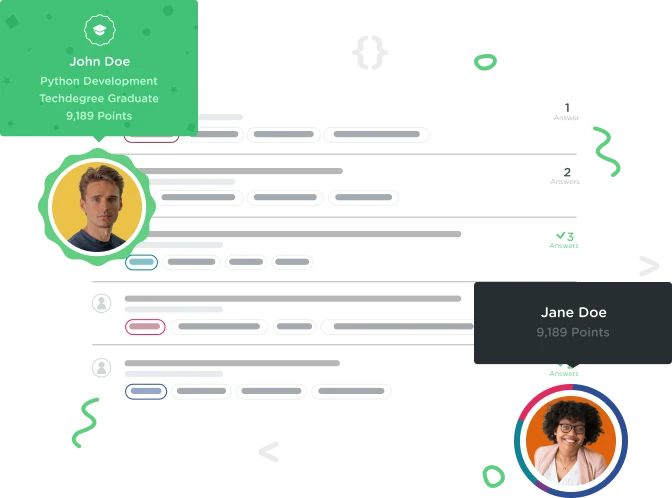

eestsaid
1,311 PointsStill stuck on disemvowel
... still stuck on disemvowel. After Stephen's assistance earlier it looked back through earlier exercises and think the for loop is more appropriate. The error that gets thrown up says that a string is being returned but it is not removing the vowels. Any comments without giving all away would be greatly appreciated.
Thanks
def disemvowel(word):
letters = word.upper()
vowels = ["A", "E", "I", "O", "U"]
for letter in letters:
if letter == vowels:
letters.remove(vowels)
return word
4 Answers
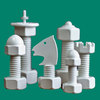
Steven Parker
231,533 PointsGlad I could help — Happy coding!
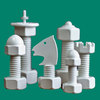
Steven Parker
231,533 PointsYour code is working on "letters" but returning "word" (the unchanged original argument).
Also, it seems to be trying to call a "remove" method on a string (string's don't have that). Perhaps you were thinking of "replace"? Or maybe you intended to convert the word into a list and then back later?
Either "remove" (for lists) or "replace" (for strings) can only work with one item at a time.
And be careful not to convert the case of letters that are not being removed!

eestsaid
1,311 PointsThanks Steven. More shuffling and getting an error which suggests getting closer.
def disemvowel(word):
word = list(word)
vowels = ["a", "e", "i", "o", "u", "A", "E", "I", "O", "U"]
for letter in vowels:
if letter in word:
word.remove(letter)
word = ''.join(letter)
return(word)
It seems to be throwing back the last letter in the vowels list...
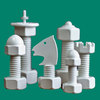
Steven Parker
231,533 PointsFrom this point, I see three issues:
- there could be more than one of each letter, you might need "while" instead of "if"
- it's not "letter" you want to join, but "word"
- don't rejoin the word until after the loop(s) are done

eestsaid
1,311 PointsThanks Steven - some progress but still not getting the vowels to remove. I'm assuming I'm not understanding how the while loop works over the vowels list. Any comments?
def disemvowel(word):
word = list(word)
vowels = ["a", "e", "i", "o", "u", "A", "E", "I", "O", "U"]
while vowels in word:
if vowels == word:
word.remove(vowels)
word = ''.join(word)
return(word)
Thanks as always
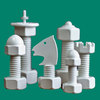
Steven Parker
231,533 PointsMy previous hints were based on leaving everything else (like the "for" loop) as it was.

eestsaid
1,311 PointsThat worked Steven - thanks so much for the patience and direction ... learned a bunch.
def disemvowel(word):
word = list(word)
vowels = ["a", "e", "i", "o", "u", "A", "E", "I", "O", "U"]
for letter in vowels:
while letter in word:
word.remove(letter)
word = ''.join(word)
return(word)