Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial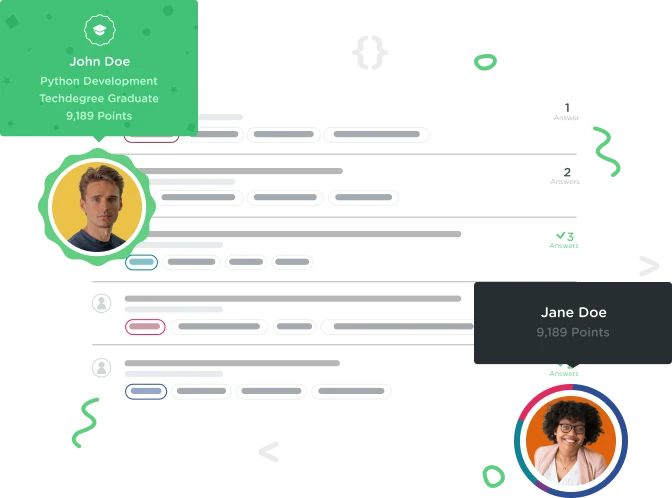

Gordon Jiroux
8,728 PointsStopping bad habits before they start
There's something troubling me. In the php lessons there is a lot of foreach usage in-lieu of "for" loops
One thing that that really troubles me is iterating through the entire array to find the last 4 items based on a conditional that checks for position. this is inefficient and can lead to bad habits going forward.
why not introduce the "for" keyword early on (as many members may be developing sites as they go) . introducing something like the code would be better, no?
(granted, I realize the video was dealing with shirts, but I copied the flavors array)
<?php
$flavors = array(
"Jalapeno So Spicy",
"Avocado Chocolate",
"Peppermint",
"Vanilla",
"Cake Batter",
"Cookie Dough"
);
for($i = count($flavors) - 4; $i < count($flavors); $i++)
{
echo $flavors[$i] . "<br>";
}
?>
7 Answers

Gordon Jiroux
8,728 Points... or one step better (I wanted to show that count() could be used "inline" above)
<?php
$flavors = array(
"Jalapeno So Spicy",
"Avocado Chocolate",
"Peppermint",
"Vanilla",
"Cake Batter",
"Cookie Dough"
);
$count_flavors = count($flavors);
for($i = $count_flavors - 4; $i < $count_flavors; $i++)
{
echo $flavors[$i] . "<br>";
}
?>

James Barnett
39,199 PointsI wonder what Randy Hoyt the PHP teacher's thoughts on this.

Gordon Jiroux
8,728 Points(Please keep in mind, I didn't want to sound like a "Tool" when typing any part of this thread. if I do, just know that it was not my intent)
I only bring this up because as a Business Owner, and Lead Developer, it troubles me when my "newer" developers (not necessarily just Jr. devs, but new to my company) write inefficient code (when it can be helped), that I have to eat the time and money for them (or me) to go back and fix because I :
1.) View inefficient code as being substandard.
2.) Refuse to release substandard code to a paying customer, and refuse to charge more without a scope change, so I eat the hours/money.
3.) Refuse to host inefficient code on my boxes (I'll only host sites we develop, redevelop, or review and deem acceptable, and maintain ourselves)
I understand development a perpetual learning process, (If I'm not learning something new every day, I'm not doing something right) and I'll spend time with them "mentoring", but I prefer when devs don't have bad habits to begin with :) We also have a peer review policy for every developer (including me), so the devs can learn from each other. It also helps to limit poor design, architecture and development decisions.
I started developing when memory and processor speed was at a premium (to me it still is and always will be)... For they people who taught me, the answer was never "let's just get a faster/better server/computer...". it was make the code as lean and efficient as possible (while staying in scope and budget).
I haven't gotten any sections of Treehouse that would that deal with it yet; but I would hope to see the differences between value and reference types (as applicable) being discussed and explored...
Another thing I hope to see being taught is; using the smallest "datatype" needed to hold a column's "maximum" value and an understanding of how, when and what goes into making decisions like using char(2) to hold a U.S. postal code, or an ISO 3166-1-alpha-2 "country" code instead of varchar(2)... I know the savings may seem trivial to some with that example, but it is just a small example of a much bigger picture... I know developers that will use varchar/nvarchar(255) to hold 2 non-variable length characters without any regard for extra storage bytes per column-per row, or the possible performance impact (varies by database and circumstance).

Randy Hoyt
Treehouse Guest TeacherHey Gordon,
A for
loop works great for the flavors example, but it won't work with the shirts array. I'd love to use one for the Recent Shirts section on the home page, but it's just not possible the way the array is structured. I spent a lot of time thinking about the best way to structure the array of shirts so that the home page, the shirts listing page, and the shirt details would work together as efficiently as possible overall.
I understand what you are saying about stopping bad habits before they start, but the other side of the equation is that the best way to do something is often not the simplest way. Imagine a PHP course that began like this:
PHP is a fun and easy programming language. The first step is to create a cluster of web servers behind a load balancer. Before we write our first line of PHP code, we also want to be sure to integrate our site with a CDN using this great command line tool.
Efficiency and simplicity are often at odds.
Also, maintainability and efficiency are often at odds. For example, minified JavaScript downloads faster but is impossible to edit. Build scripts, caching, and other advanced techniques can help reconcile those, but that's not something you can learn when you first start.
As teachers, we have to think very carefully about the right order to introduce concepts. I spent a lot of time refining the structure for the array of shirts. The only thing I wish were more efficient in the project is this one thing you've pointed out, that the list of Recent Shirts didn't have to loop through all eight shirts. But I feel this is acceptable, and I am happy with the overall result.
Also, I have found that a for loop is a bit difficult for students new to programming to grasp. I introduce the concept in a future set of PHP videos, but I do it as a while loop:
$i = 0;
while ($i < 4) {
$i += 1;
// do this four times
}
It's the same concept, but in my experience it's easier to learn when each piece is broken out separately like this.
Does that help?

Gordon Jiroux
8,728 PointsRandy Hoyt, I'm with you on the balancing act; and Ideally the shirt data would be coming from a DB anyway.
I guess my point was that i would've liked to see you call out that iterating through every item in an array isn't always the best course of action... 8 items isn't a big deal... I was speaking to the possibility of much larger arrays.
With respect to "for" being more difficult to grasp; I usually teach "foreach" in tandem with "for" (after teaching the parts: variables, "if" conditionals and "incrementing a value") which by that stage in the php series the members have seen and used.
for("variable"; "(more-or-less) an if condition that allows loop to continue"; "increment the variables value")
{
}

Gordon Jiroux
8,728 PointsGreat job with the series... in-fact all of series (that I have seen)... Kudos to the entire Treehouse Staff... Treehouse is "Top Notch", It's informative, engaging, and makes learning the material easy... I've seen others sites like this that made me want to pluck out my eyes , just out of pure boredom... I'm really enjoying Treehouse... Even though I've been working with these languages, platforms, etc for many years, I'm picking up new stuff here... GREAT JOB!!!, Treehouse gets a +1 from me... I like it so much, I've got my whole family signed up (Wife and 2 kids), and 3 others so far.... My brother should be signing up right now as well.

Randy Hoyt
Treehouse Guest TeacherHey Gordon Jiroux, I like the idea of mentioning that iterating through the whole array is not always the best course of action. Thanks! I'll go back and add that in. At the very end of the second project, after we've refactored the main products array so that we could use a for
/ while
loop, for the "extra credit" activity, I'll ask students to refactor that function using a while
loop and array_pop
or something.
In the third project, which I'll start writing soon, I'll be moving the products into a database. :~)