Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial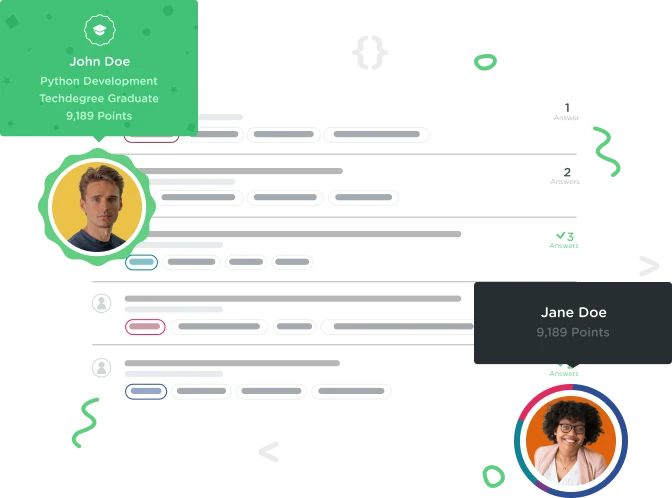
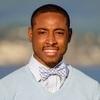
Jonathan Etienne
24,572 PointsStoring and retrieving user uploaded images in parse
I have successfully been able to store user entered information into parse, but I haven't figure out how to do store a user uploaded image that would be associated with the current user in parse, and to retrieve a user image at a later time.
To clarify this, below is the code I have used for my profile creation activity, an activity that is prompted after the user has signing using social media and responds to a series of questions like age, preferred name, and where those information are later updated in the parse database and added to the current user.
Also in the activity, the user is already able to upload a picture from their device, my issue now resolves around taking that uploaded picture and storing it on parse, and associating the image with the current user who has signing using their social media account - the social media integration is done through parse.
I have looked into the following guide, but is still greatly confused. https://www.parse.com/docs/android_guide#files
Any assistance would be greatly appreciated. Thanks in advance.
public class ProfileCreation extends Activity {
private static final int RESULT_LOAD_IMAGE = 1;
FrameLayout layout;
Button save;
protected EditText mName;
protected EditText mAge;
protected EditText mHeadline;
protected Button mConfirm;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_profile_creation);
Parse.initialize(this, "ID", "ID");
mName = (EditText)findViewById(R.id.etxtname);
mAge = (EditText)findViewById(R.id.etxtage);
mHeadline = (EditText)findViewById(R.id.etxtheadline);
mConfirm = (Button)findViewById(R.id.btnConfirm);
mConfirm.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String name = mName.getText().toString();
String age = mAge.getText().toString();
String headline = mHeadline.getText().toString();
age = age.trim();
name = name.trim();
headline = headline.trim();
if (age.isEmpty() || name.isEmpty() || headline.isEmpty()) {
AlertDialog.Builder builder = new AlertDialog.Builder(ProfileCreation.this);
builder.setMessage(R.string.signup_error_message)
.setTitle(R.string.signup_error_title)
.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
}
else {
// create the new user!
setProgressBarIndeterminateVisibility(true);
ParseUser currentUser = ParseUser.getCurrentUser();
currentUser.put("name", name);
currentUser.put("age", age);
currentUser.put("headline", headline);
currentUser.saveInBackground(new SaveCallback() {
@Override
public void done(ParseException e) {
setProgressBarIndeterminateVisibility(false);
if (e == null) {
// Success!
Intent intent = new Intent(ProfileCreation.this, MoodActivity.class);
intent.addFlags(Intent.FLAG_ACTIVITY_NEW_TASK);
intent.addFlags(Intent.FLAG_ACTIVITY_CLEAR_TASK);
startActivity(intent);
}
else {
AlertDialog.Builder builder = new AlertDialog.Builder(ProfileCreation.this);
builder.setMessage(e.getMessage())
.setTitle(R.string.signup_error_title)
.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
}
}
});
}
}
});
save = (Button) findViewById(R.id.button2);
String picturePath = PreferenceManager.getDefaultSharedPreferences(this).getString("picturePath", "");
if (!picturePath.equals("")) {
ImageView imageView = (ImageView) findViewById(R.id.profilePicturePreview);
imageView.setImageBitmap(BitmapFactory.decodeFile(picturePath));
}
SeekBar seekBar = (SeekBar) findViewById(R.id.seekBarDistance);
final TextView seekBarValue = (TextView) findViewById(R.id.seekBarDistanceValue);
seekBar.setOnSeekBarChangeListener(new SeekBar.OnSeekBarChangeListener() {
@Override
public void onProgressChanged(SeekBar seekBar, int progress,
boolean fromUser) {
// TODO Auto-generated method stub
seekBarValue.setText(String.valueOf(progress));
}
@Override
public void onStartTrackingTouch(SeekBar seekBar) {
// TODO Auto-generated method stub
}
@Override
public void onStopTrackingTouch(SeekBar seekBar) {
// TODO Auto-generated method stub
}
}); // Add this
SeekBar seekBarMinimum = (SeekBar) findViewById(R.id.seekBarMinimumAge);
final TextView txtMinimum = (TextView) findViewById(R.id.tMinAge);
seekBarMinimum.setOnSeekBarChangeListener(new SeekBar.OnSeekBarChangeListener() {
@Override
public void onProgressChanged(SeekBar seekBar, int progress,
boolean fromUser) {
// TODO Auto-generated method stub
txtMinimum.setText(String.valueOf(progress));
}
@Override
public void onStartTrackingTouch(SeekBar seekBar) {
// TODO Auto-generated method stub
}
@Override
public void onStopTrackingTouch(SeekBar seekBar) {
// TODO Auto-generated method stub
}
}); // Add this
SeekBar seekBarMaximum = (SeekBar) findViewById(R.id.seekBarMaximumAge);
final TextView txtMaximum = (TextView) findViewById(R.id.tMaxAge);
seekBarMaximum.setOnSeekBarChangeListener(new SeekBar.OnSeekBarChangeListener() {
@Override
public void onProgressChanged(SeekBar seekBar, int progress,
boolean fromUser) {
// TODO Auto-generated method stub
txtMaximum.setText(String.valueOf(progress));
}
@Override
public void onStartTrackingTouch(SeekBar seekBar) {
// TODO Auto-generated method stub
}
@Override
public void onStopTrackingTouch(SeekBar seekBar) {
// TODO Auto-generated method stub
}
}); // Add this
Button buttonLoadImage = (Button) findViewById(R.id.btnPictureSelect);
buttonLoadImage.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View arg0) {
Intent i = new Intent(
Intent.ACTION_PICK,
android.provider.MediaStore.Images.Media.EXTERNAL_CONTENT_URI);
startActivityForResult(i, RESULT_LOAD_IMAGE);
}
});
save.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View arg0) {
// Locate the image in res >
Bitmap bitmap = BitmapFactory.decodeFile("picturePath");
// Convert it to byte
ByteArrayOutputStream stream = new ByteArrayOutputStream();
// Compress image to lower quality scale 1 - 100
bitmap.compress(Bitmap.CompressFormat.PNG, 100, stream);
Object image = null;
try {
String path = null;
image = readInFile(path);
} catch (Exception e) {
e.printStackTrace();
}
// Create the ParseFile
ParseFile file = new ParseFile("picturePath", (byte[]) image);
// Upload the image into Parse Cloud
file.saveInBackground();
// Create a New Class called "ImageUpload" in Parse
ParseObject imgupload = new ParseObject("Image");
// Create a column named "ImageName" and set the string
imgupload.put("Image", "picturePath");
// Create a column named "ImageFile" and insert the image
imgupload.put("ImageFile", file);
// Create the class and the columns
imgupload.saveInBackground();
// Show a simple toast message
}
});
}
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (requestCode == RESULT_LOAD_IMAGE && resultCode == RESULT_OK
&& null != data) {
Uri selectedImage = data.getData();
String[] filePathColumn = { MediaStore.Images.Media.DATA };
Cursor cursor = getContentResolver().query(selectedImage,
filePathColumn, null, null, null);
cursor.moveToFirst();
int columnIndex = cursor.getColumnIndex(filePathColumn[0]);
String picturePath = cursor.getString(columnIndex);
cursor.close();
ImageView imageView = (ImageView) findViewById(R.id.profilePicturePreview);
imageView.setImageBitmap(BitmapFactory.decodeFile(picturePath));
}
}
private byte[] readInFile(String path) throws IOException {
// TODO Auto-generated method stub
byte[] data = null;
File file = new File(path);
InputStream input_stream = new BufferedInputStream(new FileInputStream(
file));
ByteArrayOutputStream buffer = new ByteArrayOutputStream();
data = new byte[16384]; // 16K
int bytes_read;
while ((bytes_read = input_stream.read(data, 0, data.length)) != -1) {
buffer.write(data, 0, bytes_read);
}
input_stream.close();
return buffer.toByteArray();
}
}
Again I greatly appreciate your support in advance.