Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial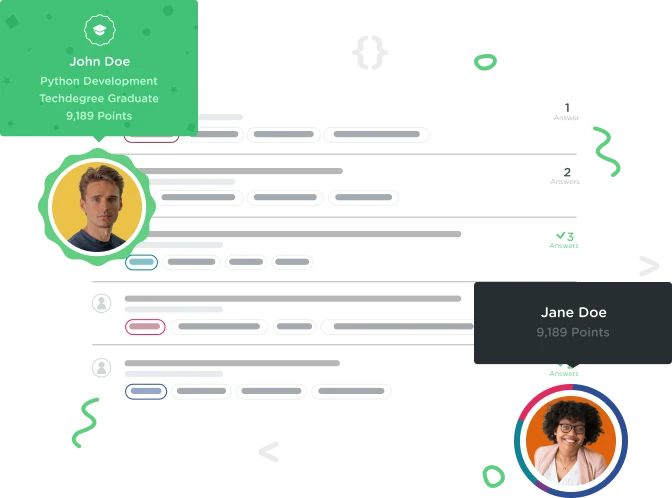

vryiziilos
Full Stack JavaScript Techdegree Student 23,052 Pointsstoring data in array vs database
I am currently on PHP dev track and I haven't yet fully understand databases and how to store and retrieve info from them. But now I have a good grasp of arrays and specifically associative arrays.
I am building a website for a construction company that has over 30 completed projects to display on a page and I am not sure how to tackle this. Before I waste my time storing data inefficiently I wanted to consult with you. Should I just create a big associative array or wait and go through database courses and then store in a db and retrieve that data via PHP?
Also, I know a bit about JSON. Do you think I should maybe store all the project info in one JSON file and then retrieve it using AJAX?
P.S. Project data is not sensitive it's just a project description and a bunch of images associated with it.
Thanks!
3 Answers
jordans
1,609 PointsThe biggest reason you would want to use a database over an array is if users enter information one day, and retrieve it another day. Databases are good for holding data for long periods of time.
Another reason someone may use a database is if you are storing large amounts of data.
An advantage with arrays over databases is the speed. A database call is going to be much slower than accessing an element in an array. It sounds to me you would be best using an array.
jordans
1,609 PointsThe guide below is for a OS X computer. If you decide not to use a database, you're gonna have some trouble in the future. But if so, I suggest encoding your array into JSON format with this PHP code:
$projects["id"] = array(
"title" => "Project Title #1",
"begin_date" => "8/1/2005",
"end_date" => "1/1/2006",
"investor" => "Berkshire Hathaway, Inc.",
"client" => "Microsoft",
"work_as" => "Contractor",
"photos" => array(
"http://lorempicsum.com/nemo/400/200/1",
"http://lorempicsum.com/nemo/400/200/2",
"http://lorempicsum.com/nemo/400/200/3",
"http://lorempicsum.com/nemo/400/200/4"
)
);
echo json_encode($projects);
Once you've done that, set up a PostreSQL database server and create a new database and then connect to it by typing:
createdb json_test
psql json_test
Now you need to create the table inside the database. You can do this by typing:
CREATE TABLE json_table ( id INTEGER, doc JSON);
Next, copy the JSON data from your PHP page and make some minor tweaks so it looks something similar to this:
INSERT INTO json_table VALUES ( 1, '{
"title": "Project Title #1",
"begin_date": "8/1/2005",
"end_date": "1/1/2006",
"investor": "Berkshire Hathaway, Inc.",
"client": "Microsoft",
"work_as": "Contractor",
"photos":{
"1":"http://lorempicsum.com/nemo/400/200/1",
"2":"http://lorempicsum.com/nemo/400/200/2",
"3":"http://lorempicsum.com/nemo/400/200/3",
"4:"http://lorempicsum.com/nemo/400/200/4"
}
}');
When you are done with that, just copy and paste it into your terminal and hit enter. You should get a "INSERT" message. To check your table type "SELECT * FROM first;".
Here's what your database should look like:

vryiziilos
Full Stack JavaScript Techdegree Student 23,052 Pointsjordan, thanks for your answer. I was initially thinking of adding an array to the project as well. I just wasn't quite sure if it's the better way of storing data. I guess my concern was that if the data grows I will need to migrate the array to a database. Is there a way to convert an array to a database using PHP?
jordans
1,609 PointsIt depends on how on how your array is made. To be honest, if you're worrying on whether you may need to switch to a database in the future, you mine as well just use a database. Databases aren't very difficult to learn and they will save you a lot of trouble in the future if you do decide to expand.
If you can show me an example of what your array may look like, I can let you know how difficult it'd be to convert it.

vryiziilos
Full Stack JavaScript Techdegree Student 23,052 Points// array sample
// data is fictional.
$projects[1] = array(
"title" => "Project Title #1",
"begin_date" => "8/1/2005",
"end_date" => "1/1/2006",
"investor" => "Berkshire Hathaway, Inc.",
"client" => "Microsoft",
"work_as" => "Contractor",
"photos" => array(
"img/featured-gallery/featured1.jpg",
"img/featured-gallery/featured2.jpg",
"img/featured-gallery/featured3.jpg",
"img/featured-gallery/featured4.jpg"
)
);
// ... and there are about 30 similar arrays