Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial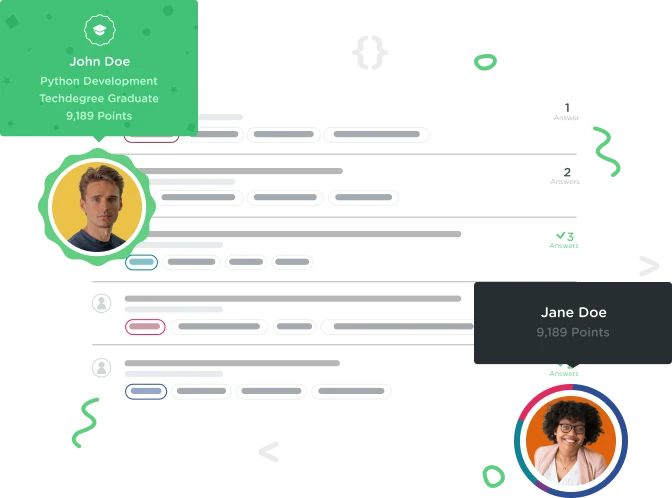

Liam Dawson
13,530 PointsStoring Values in SASS Maps - Using Breakpoints
Could someone please give me a nudge in the right direction if I'm understanding this incorrectly? I get that it works but I've been struggling to understand how it works or what's happening in the code. I've written just below my understanding of it so far but it just hasn't been 'clicking'.
From what (I think) I understand, $value: map-get($breakpoints, $break); tells the mixin to look at the $breakpoints map and use that as a reference for ($break). So when you @include the mixin in your stylesheet, you can use any of the keys in the map as the value referred to in the (parentheses) and the browser will check the map to see if it matches up with what's listed.
$sm: map-get($breakpoints, 'sm'); tells the mixin to use 'sm' value held in the $breakpoints map (600px) as the value whenever it sees $sm.
@if $value < $sm tells the mixin to look for any of the values held in the $breakpoints map that are less than the value held by $sm (which is 'sm': 600px) - so everything up to 599px (or 'xs: 599px). It then applies its nested @media query to anything in the $breakpoints map as long as it's less than 600px.
Then there's the @else rule.
Here, the @media query tells the mixin that whenever the minimum width is the same as $value, then it should apply its nested rule. We tell the browser what $value is when we insert an entry to the @include's (parentheses) which matches up with a key held in the map - so in this case: 'xs', 'sm', 'm', 'l'.
So if I were to add
@include mq(xl) {
background-color: yellow;
}
then the browser would look at for @mixin mq. Then would look for the meaning of 'xl' within the $breakpoints map, the browser would then know to apply a yellow background color if ever the width reaches 1500px or more. If in the (parentheses) I wrote 'xxl' then nothing would happen as this does not correspond to anything in the map being referred to.
$breakpoints: (
'xs': 599px,
'sm': 600px,
'm': 800px,
'l': 1200px,
'xl': 1500px,
);
@mixin mq($break) {
$value: map-get($breakpoints, $break);
$sm: map-get($breakpoints, 'sm');
@if $value < $sm {
@media (max-width: $value) {
@content;
}
}
@else {
@media (min-width: $value) {
@content;
}
}
}
body {
@include mq(xs) {
background-color: red;
}
@include mq(m) {
background-color: green;
}
@include mq(l) {
background-color: blue;
}
}
Apologies for the long question/theory - Any help appreciated!
2 Answers
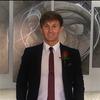
Liam Clarke
19,938 PointsHI Liam
Yes, you've got it. the mixin is just a way of following DRY coding standards and you've pretty much understood what it does.
if we take it step by step:
- include the mixin
@include mq(xl) {
background-color: yellow;
}
- scss will go to the mixin with the parameter of "xl" and store it as a variable named "$value" which will get value from the "$breakpoints" map
@mixin mq($break) {
$value: map-get($breakpoints, $break); // This now holds the value of 'xl', 1500px
}
- scss will set a variable named "$sm" and store the value of 'sm' from the $breakpoints map
@mixin mq($break) {
$sm: map-get($breakpoints, 'sm'); // This now holds the value of 'sm', 600px
}
- Then declare a condition, if $value (1500px) is less than $sm (600px), then set @media (max-width: $value) and the content
@mixin mq($break) {
@if $value < $sm {
@media (max-width: $value) {
@content;
}
}
}
- else set @media (min-width: $value) and the content
@mixin mq($break) {
@else {
@media (min-width: $value) {
@content;
}
}
}
All together with variable values
@include mq(xl) {
background-color: yellow;
}
$breakpoints: (
'xs': 599px,
'sm': 600px,
'm': 800px,
'l': 1200px,
'xl': 1500px,
);
@mixin mq(xl) {
$value: map-get($breakpoints, xl); // 1500px
$sm: map-get($breakpoints, sm); //600px
@if 1500px < 600px {
@media (max-width: 1500px) {
@content;
}
}
@else {
@media (min-width: 1500px) {
@content;
}
}
}
So from that example, you will get
@media (min-width: 1500px) {
background-color: yellow;
}
Hope this helps!

Chapman Cheng
PHP Development Techdegree Graduate 46,936 Pointsthanks for the answer Liam, so thorough
Liam Dawson
13,530 PointsLiam Dawson
13,530 PointsWow, that's really helpful - thanks, Liam!