Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial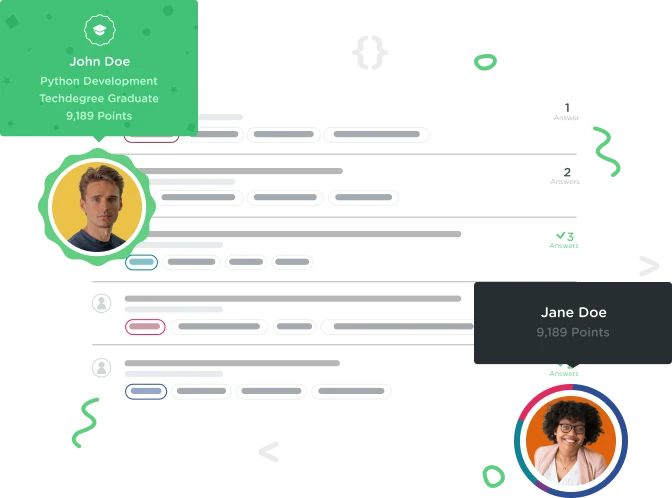

James Rodney
2,005 PointsSTORMY CRASHED AND I'm getting the error Error:(5) No resource identifier found for attribute 'showAsAction' in package
Help me
Code ... Error:Execution failed for task ':app:processDebugResources'.
com.android.ide.common.process.ProcessException: org.gradle.process.internal.ExecException: Process 'command 'C:\Users\James\AppData\Local\Android\sdk\build-tools\21.1.2\aapt.exe'' finished with non-zero exit value 1
package com.jamesrodney2020gmail.stormy.ui;
import android.app.Activity; import android.content.Intent; import android.os.Bundle; import android.os.Parcelable; import android.support.v7.widget.LinearLayoutManager; import android.support.v7.widget.RecyclerView;
import com.jamesrodney2020gmail.stormy.R; import com.jamesrodney2020gmail.stormy.adapters.HourAdapter; import com.jamesrodney2020gmail.stormy.weather.Hour;
import java.util.Arrays;
import butterknife.ButterKnife; import butterknife.InjectView;
public class HourlyForecastActivity extends Activity {
private Hour[] mHours;
@InjectView(R.id.reyclerView) RecyclerView mRecyclerView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_hourly_forecast);
ButterKnife.inject(this);
Intent intent = getIntent();
Parcelable[] parcelables = intent.getParcelableArrayExtra(MainActivity.HOURLY_FORECAST);
mHours = Arrays.copyOf(parcelables, parcelables.length, Hour[].class);
HourAdapter adapter = new HourAdapter(mHours);
mRecyclerView.setAdapter(adapter);
RecyclerView.LayoutManager layoutManager = new LinearLayoutManager(this);
mRecyclerView.setLayoutManager(layoutManager);
mRecyclerView.setHasFixedSize(true);
}
}
package com.jamesrodney2020gmail.stormy.adapters;
import android.support.v7.widget.RecyclerView; import android.view.LayoutInflater; import android.view.View; import android.view.ViewGroup; import android.widget.ImageView; import android.widget.TextView;
import com.jamesrodney2020gmail.stormy.R; import com.jamesrodney2020gmail.stormy.weather.Hour;
/**
-
Created by James on 4/13/2015. */ public class HourAdapter extends RecyclerView.Adapter<HourAdapter.HourViewHolder> {
private Hour[] mHours;
public HourAdapter(Hour[] hours) { mHours = hours;
}
@Override public HourViewHolder onCreateViewHolder(ViewGroup parent, int viewType) { View view = LayoutInflater.from(parent.getContext()) .inflate(R.layout.hourly_list_item, parent, false); HourViewHolder viewHolder = new HourViewHolder(view); return viewHolder; }
@Override public void onBindViewHolder(HourViewHolder holder, int position) { holder.bindHour(mHours[position]);
}
@Override public int getItemCount() { return mHours.length; }
public class HourViewHolder extends RecyclerView.ViewHolder {
public TextView mTimeLabel; public TextView mSummaryLabel; public TextView mTemperatureLabel; public ImageView mIconImageView; public HourViewHolder(View itemView) { super(itemView); mTimeLabel =(TextView) itemView.findViewById(R.id.timeLabel); mSummaryLabel = (TextView) itemView.findViewById(R.id.summaryLabel); mTemperatureLabel = (TextView) itemView.findViewById(R.id.temperatureLabel); mIconImageView = (ImageView) itemView.findViewById(R.id.iconImageView); } public void bindHour(Hour hour) { mTimeLabel.setText(hour.getHour()); mSummaryLabel.setText(hour.getSummary()); mTemperatureLabel.setText(hour.getTemperature() + ""); mIconImageView.setImageResource(hour.getIconId()); }
}
}
2 Answers

James Rodney
2,005 Pointsit also brings me to this
<menu xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" tools:context="com.jamesrodney2020gmail.stormy.ui.HourlyForecastActivity"> <item android:id="@+id/action_settings" android:title="@string/action_settings" android:orderInCategory="100" app:showAsAction="never"/> </menu>

James Simshaw
28,738 PointsHello,
From what I'm reading here, there are a couple of ways to solve this. The easiest is to verify that you are including
dependencies {
compile 'com.android.support:appcompat-v7:+'
}
in your build.gradle file. Let me know if that helps. If not, we'd need to see your build.gradle files and possibly your AndroidManifest.xml file.