Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial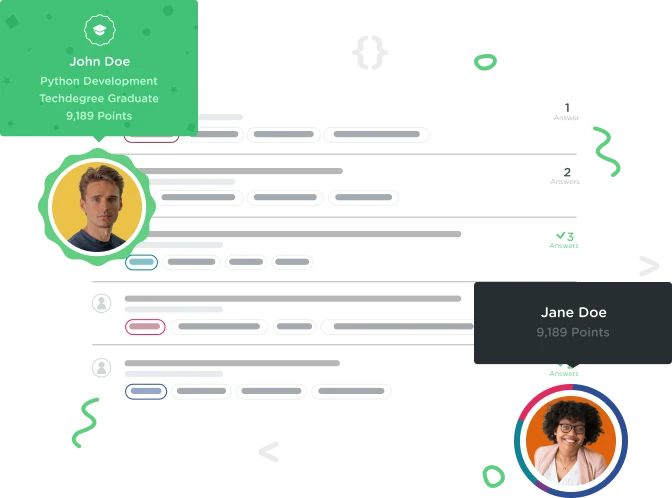

Matt Otis
1,402 Points__str__ morse.py Challenge
Def str works perfectly as an independent function in my IDE; unsure why it’s failing the challenge task check.
class Letter:
def __init__(self, pattern=None):
self.pattern = pattern
def __str__(pattern)
morse = []
for piece in pattern:
if piece == ".":
morse.append("dot")
elif piece == "_":
morse.append("dash")
return '-'.join(morse)
class S(Letter):
def __init__(self):
pattern = ['.', '.', '.']
super().__init__(pattern)
4 Answers
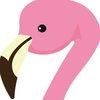
Dave StSomeWhere
19,870 PointsA couple of key items:
- pattern is a class attribute, so you shouldn't be passing it to your str() method
- What is needed in your str() method is self (should be first parameter - most of the time)
- So then you'll have access to your pattern variable with self.pattern
Does that make sense or do you need to see the actual code?

Matt Otis
1,402 PointsWhile I thought I was following you, I tried following your steps and still Bummer
def __str__(self) #Step 1: lose ‘pattern’ as it’s an attribute, not a value. / Step 2: Pass in ‘self’ to the str() method
morse = []
for piece in self.pattern: #Step 3: Access to the pattern’s value with self.pattern
if piece == ".":
morse.append("dot")
elif piece == "_":
morse.append("dash")
return '-'.join(morse)
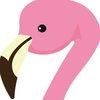
Dave StSomeWhere
19,870 PointsOne other important item I forgot - you need to terminate your def
statement with a colon ":" - I added that in with your code and the other changes and it passed.
So it looks good - just add the colon

KRIS NIKOLAISEN
54,971 PointsYou are close. You just need a colon at the end here:
def __str__(self):

Matt Otis
1,402 PointsGah, always with the little things!
: added, and all works great, thanks y’all.
def __str__(self):
morse = []
for piece in self.pattern:
if piece == ".":
morse.append("dot")
elif piece == "_":
morse.append("dash")
return '-'.join(morse)