Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial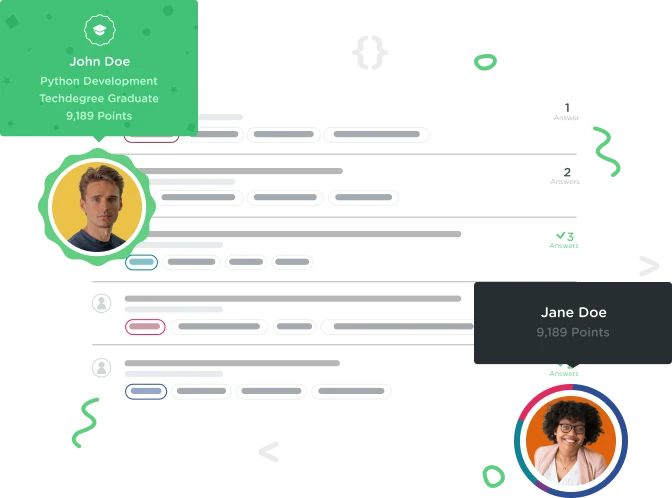
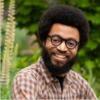
Nicolas Hampton
44,638 PointsStrange error in compareTo if both dates are equal
So I ran the compareTo method in the repl to test it out. Seems the date sort is working great, but when I run the method when both dates are the same and the descriptions are "body" and "Zody" respectively, the return value is 24, indicating that for strings the compareTo method gave us the total distance between letters instead of a simple sort. Are we going to deal with that?
1 Answer
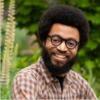
Nicolas Hampton
44,638 PointsI changed the method a little to eliminate most of the problem (would have a problem if both of the statements are exactly the same, but we check for that earlier, so it should be that much of a problem). Is this any better?
@Override
public int compareTo(Object obj) {
Treet other = (Treet) obj;
if(equals(other)) {
return 0;
}
int dateCmp = mCreationDate.compareTo(other.mCreationDate);
if (dateCmp == 0) {
int descriptionCmp = mDescription.compareTo(other.mDescription);
if (descriptionCmp > 0) {
return 1;
} else {
return -1;
}
}
return dateCmp;
}
Nicolas Hampton
44,638 PointsNicolas Hampton
44,638 PointsYeah, I looked up compareTo on the oracle documentation, and it explained the problem:
Compares two strings lexicographically. The comparison is based on the Unicode value of each character in the strings. The character sequence represented by this String object is compared lexicographically to the character sequence represented by the argument string. The result is a negative integer if this String object lexicographically precedes the argument string. The result is a positive integer if this String object lexicographically follows the argument string. The result is zero if the strings are equal; compareTo returns 0 exactly when the equals(Object) method would return true. This is the definition of lexicographic ordering. If two strings are different, then either they have different characters at some index that is a valid index for both strings, or their lengths are different, or both. If they have different characters at one or more index positions, let k be the smallest such index; then the string whose character at position k has the smaller value, as determined by using the < operator, lexicographically precedes the other string. In this case, compareTo returns the difference of the two character values at position k in the two string -- that is, the value:
this.charAt(k)-anotherString.charAt(k)
If there is no index position at which they differ, then the shorter string lexicographically precedes the longer string. In this case, compareTo returns the difference of the lengths of the strings -- that is, the value: this.length()-anotherString.length()