Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial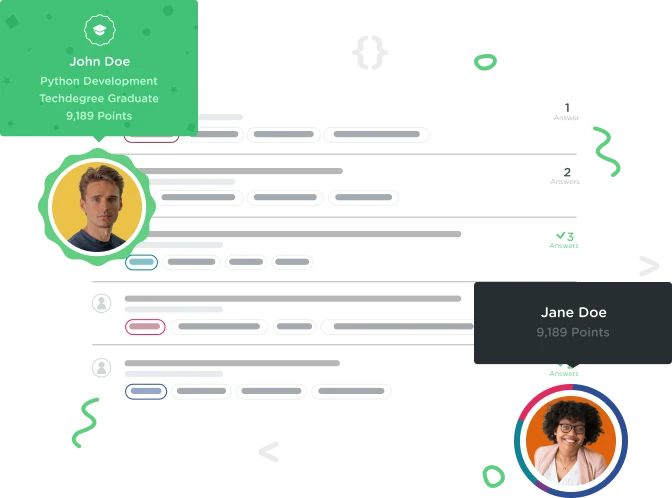
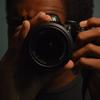
Jordan Welch
1,771 PointsStrange Error in Java
I am working on the blog reader app, and everything is going smooth when I try to make a new class for GetBlogPostsTask I get a error that says "Illegal Modifier" Ben Jakuben Did the same exact code and did not get that error here is my code for reference.
package com.jwelchapps.blogreader;
import java.io.IOException;
import java.net.HttpURLConnection;
import java.net.MalformedURLException;
import java.net.URL;
import android.app.ListActivity;
import android.os.AsyncTask;
import android.os.Bundle;
import android.util.Log;
import android.view.Menu;
import android.view.MenuItem;
public class MainListActivity extends ListActivity {
protected String[] mBlogPostTitles;
public static final int NUMBER_OF_POSTS = 20;
public static final String TAG = MainListActivity.class.getSimpleName();
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main_list);
//Toast.makeText(this, getString(R.string.no_items), Toast.LENGTH_LONG).show();
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main_list, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
if (id == R.id.action_settings) {
return true;
}
private class GetBlogPostsTask extends AsyncTask<Object, Void, String> {
@Override
protected String doInBackground(Object... params) {
int responseCode = -1;
try {
URL blogFeedUrl = new URL("http://blog.teamtreehouse.com/api/get_recent_summary/?count=" + NUMBER_OF_POSTS);
HttpURLConnection connection = (HttpURLConnection) blogFeedUrl.openConnection();
connection.connect();
responseCode = connection.getResponseCode();
Log.i(TAG, "Code: " + responseCode);
}
catch (MalformedURLException e) {
Log.e(TAG, "Exception caught: ", e);
}
catch (IOException e) {
Log.e(TAG, "Exception caught: ", e);
}
catch (Exception e) {
Log.e(TAG, "Exception caught: ", e);
}
return "Code: " + responseCode;
}
}
return super.onOptionsItemSelected(item);
}
}
The error is on line 47 thanks in advance to anyone who can help
6 Answers
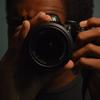
Jordan Welch
1,771 PointsSomeone help me please :)
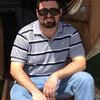
Zack Yovel
8,966 PointsI believe the illegal modifier is the 'class' keyword, you can't define an inner class inside a function. You must either define it outside the function and instantiate it in the function or make it an anonymous class.
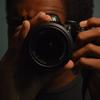
Jordan Welch
1,771 PointsI know that's what it means but Ben Jakuben did it and got no errors
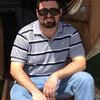
Zack Yovel
8,966 PointsWell, I didn't see Ben's videos yet, tell me in what video he does this and in what time in the video or if this is in the project files tell be in what file and I will check it out.
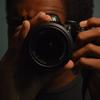
Jordan Welch
1,771 Points3:25
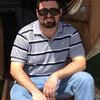
Zack Yovel
8,966 PointsBen's AsyncTask subclass is nested in the activity class, not within a function. Notice how it shows under the closing brace of the onCreateOptionsMenu(Menu menu){... } method. Your AsyncTask subclass is nested withing the curly braces of a method, that's illegal.
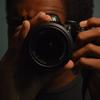
Jordan Welch
1,771 PointsI moved it an my main error went away now I have a error in onOptionsItemSelected
package com.jwelchapps.blogreader;
import java.io.IOException;
import java.net.HttpURLConnection;
import java.net.MalformedURLException;
import java.net.URL;
import android.app.ListActivity;
import android.os.AsyncTask;
import android.os.Bundle;
import android.util.Log;
import android.view.Menu;
import android.view.MenuItem;
public class MainListActivity extends ListActivity {
protected String[] mBlogPostTitles;
public static final int NUMBER_OF_POSTS = 20;
public static final String TAG = MainListActivity.class.getSimpleName();
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main_list);
try {
URL blogFeedUrl = new URL("http://blog.teamtreehouse.com/api/get_recent_summary/?count=" + NUMBER_OF_POSTS);
HttpURLConnection connection = (HttpURLConnection) blogFeedUrl.openConnection();
connection.connect();
int responseCode = connection.getResponseCode();
Log.i(TAG, "Code: " + responseCode);
}
catch (MalformedURLException e) {
Log.e(TAG, "Exception caught: ", e);
}
catch (IOException e) {
Log.e(TAG, "Exception caught: ", e);
}
catch (Exception e) {
Log.e(TAG, "Exception caught: ", e);
}
//Toast.makeText(this, getString(R.string.no_items), Toast.LENGTH_LONG).show();
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main_list, menu);
return true;
}
private class GetBlogPostsTask extends AsyncTask<Object, Void, String> {
@Override
protected String doInBackground(Object... params) {
// TODO Auto-generated method stub
return null;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
if (id == R.id.action_settings) {
return true;
}
return super.onOptionsItemSelected(item);
}
}
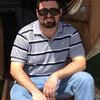
Zack Yovel
8,966 PointsIt's because you never close your AsyncTask subclass (you are missing one "}" before the "@Override"). It's just a guess, you forgot to say what the error message was...
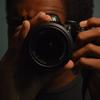
Jordan Welch
1,771 PointsThanks zack!
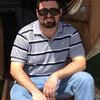
Zack Yovel
8,966 PointsNo problem Jordan.