Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial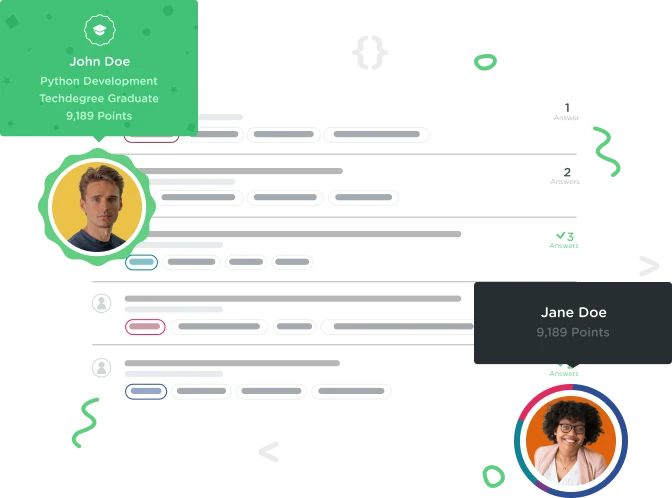
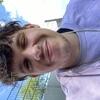
Marvin Deutz
8,349 PointsStream or enhanced for loop
Wouldn't it be more efficient to use a Stream and filter for the findByName method? Was just wondering!

okilydokily
12,105 PointsAgreed and thanks! I was hoping to find this code in the q and a.

cdlvr
14,448 PointsThe efficiency difference for something like this is negligible in the grand scheme of things. Make the decision based on style preference and readability. Worry about the efficiency of different methods of searching/looping only if you're profiled a performance bottle neck related to that code.
Here's a slightly more succinct (IMO) Stream version:
public Gif findByName(String name) {
return ALL_GIFS.stream()
.filter(gif -> gif.getName().equals(name))
.findFirst()
.orElse(null);
}
liamthornback2
9,618 Pointsliamthornback2
9,618 PointsI used a stream method like this:
I think my code looks a little cleaner and is easier to follow (if you understand streams and a little functional programming).
For most, I would guess his method was probably easier to follow.
Also Intelij is complaining that my conditional can be rewritten in functional style, even though it already is in functional style....