Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial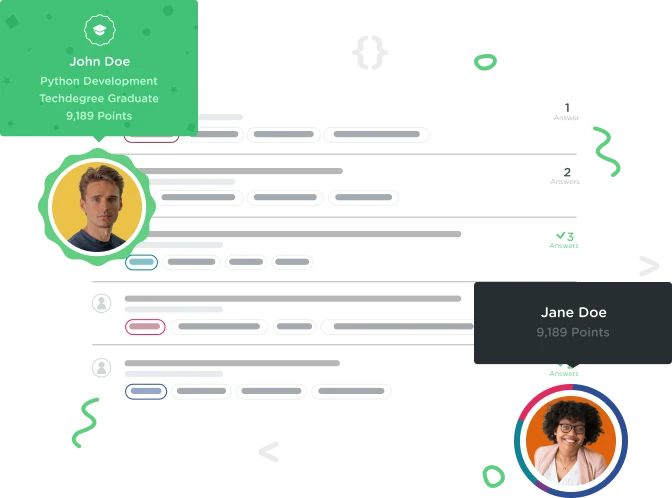

Nicholas Simpson
8,341 Pointsstrftime
I'm having some trouble understanding the format for strftime and strptime. Could someone assist me by explaining where the issue is in my code?
## Examples
# to_string(datetime_object) => "24 September 2012"
# from_string("09/24/12 18:30", "%m/%d/%y %H:%M") => datetime
def to_string(datetime_object):
return datetime_object.strftime("%d %B %Y")
def from_string(datetime_object, strftime_object):
return datetime_object.strftime_object("09/24/12 18:30", "%m/%d/%y %H:%M")
2 Answers
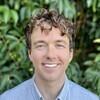
Asher Orr
Python Development Techdegree Graduate 9,409 PointsHi Nicholas!
You should check out the Python documentation for strptime and strftime: https://docs.python.org/3/library/datetime.html?highlight=datetime#strftime-and-strptime-behavior
strptime returns a datetime object (from a string)
strftime returns a string, formatted to look like a datetime object.
In task two, you are converting a string into a datetime object.
First: your function arguments are not labeled correctly. The task says "Create a new function named from_string that takes two arguments: a date as a string and an strftime-compatible format string."
Your arguments are currently this:
def from_string(datetime_object, strftime_object):
When they should look like this:
def from_string(date_as_string, compatible_format_string):
Now, look at this bit of instructions: "Create a new function named from_string that takes two arguments: a date as a string and an strftime-compatible format string, and returns a datetime created from them."
Since you need a datetime object, and your arguments are strings, you'll be using strptime (string parsed into time.)
Just like you used the strftime method on task 1, you need to use the strptime method on task 2.
In this video, Kenneth details how to use strptime: https://teamtreehouse.com/library/dates-and-times-in-python/dating-methods
I suggest re-watching that, and then try to re-write your function.
Also, as Steven said, the the examples in the comments are for reference only. You'll use the parameters in your method.
For example:
def from_string(date_as_string, compatible_format_string):
return answer(date_as_string, compatible_format_string)
I hope this helps. Let me know if you have more questions!
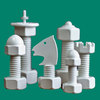
Steven Parker
231,269 PointsCheck the instructions again for what the function arguments should be: "a date as a string and an strftime-compatible format string,".
So here's a few hints:
- both arguments will be strings, neither one is a datetime object
- while strftime is useful in converting to a string, converting from one will likely require strptime
- since there's no object (until you make one) you'll need to access the method directly through the class
- the examples in the comments are for reference only, use the parameters instead of string literals
Nicholas Simpson
8,341 PointsNicholas Simpson
8,341 PointsThanks for the help! Definitely cleared that up for me!