Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial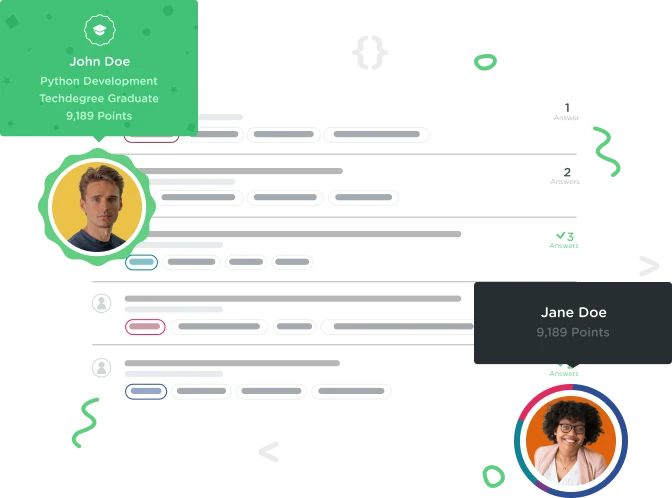

Daniel Alfaro
Courses Plus Student 11,938 PointsStrict equality "===" doesn't recognize right answer
In my [questions] array, one of the answers is a number.
At first I tried using a strict equality in my if statement "===" but the second question wouldn't be logged as correct.
I had to change to the if condition to "==" for it to work.
Is there a reason for this that I might have missed?
Thanks in advance! (:
// 1. Create a multidimensional array to hold quiz questions and answers
let questions = [
["This should be 'y'? y/n","y"],
["This should be 34? y/n",34],
["This should be 'y'? y/n","y"]
];
// 2. Store the number of questions answered correctly
let score = 0;
/*
3. Use a loop to cycle through each question
- Present each question to the user
- Compare the user's response to answer in the array
- If the response matches the answer, the number of correctly
answered questions increments by 1
*/
for (let i = 0; i < questions.length; i++) {
let answer = prompt(questions[i][0]);
if ( answer == questions[i][1]) {
score += 1;
console.log(score);
}
}
// 4. Display the number of correct answers to the user
let html = `
<h1>You scored <strong>${score}</strong>/3 points</h1>
`;
document.querySelector('main').innerHTML = html;
1 Answer
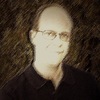
Jason Anders
Treehouse Moderator 145,860 PointsThe strict equality
check can and will often lead to problems like you are experiencing. With this operator, the check will be for BOTH value
and type
.
When you are using prompt
in JavaScript, the input will always be a string. So, when you put 34 as the answer to the question, the compiler reads "34"... which does not === 34, as one is a string and one a number.
Unless the coding requires you to check for both value and type, strict equals
is not a very popular operator.
Hope that helps. :)