Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial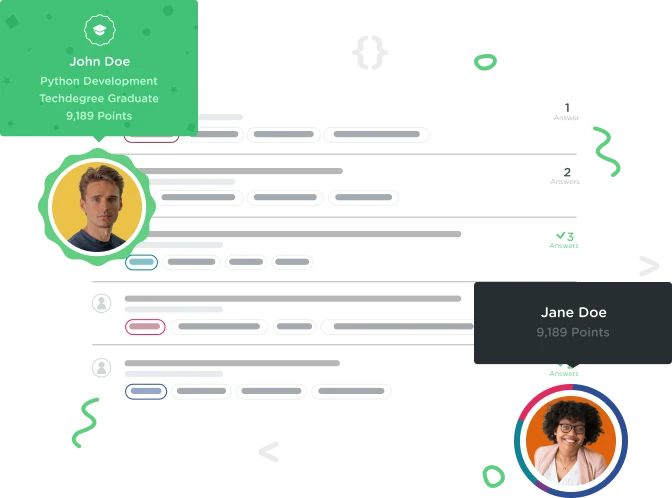
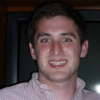
Richard Luick
10,955 PointsString Everywhere! Extra Credit - Rebuilding from Scratch
Hello, I decided to tackle the extra credit called Strings Everywhere! after the Rebuilding from Scratch lesson for the Blog Reader which asks to redo the Crystal Ball app using String resources. In my strings.xml file I created the appropriate string array and then called it in my CrystalBall.java file as shown in the lessons. However, I am getting an error "The method getResources() is undefined for the type CrystalBall". If anyone could help me understand the reasoning for this error and how I can fix it that would be great. My code is below.
<?xml version="1.0" encoding="utf-8"?>
<resources>
<string name="app_name">Crystal Ball</string>
<string name="hello_world">Hello world!</string>
<string name="action_settings">Settings</string>
<string-array name="ball_answers">
<item>It is certain</item>
<item>It is decidedly so</item>
<item>All signs say YES</item>
<item>The stars are not aligned</item>
<item>My reply is no</item>
<item>It is doubtful</item>
<item>Better not tell you now</item>
<item>Concentrate and ask again</item>
<item>Unable to answer now</item>
</string-array>
</resources>
package com.richluick.crystalball;
import java.util.Random;
import android.content.res.Resources;
public class CrystalBall {
Resources resources = getResources();
public String[] mAnswers = resources.getStringArray(R.array.ball_answers);
public String getAnAnswer() {
String answer = "";
Random randomGenerator = new Random();
int randomNumber = randomGenerator.nextInt(mAnswers.length);
answer = mAnswers[randomNumber];
return answer;
}
}
Thanks!
1 Answer

John McDonald
13,958 PointsTry
public class CrystalBall extends Activity {
Richard Luick
10,955 PointsRichard Luick
10,955 PointsSo that clears up the error in the code but when I launch the app on the emulator it crashes immediately. The LogCat is posted below.
John McDonald
13,958 PointsJohn McDonald
13,958 PointsSorry, try addng the new activity to the manifest and wrap this code:
In an onCreate() method
Richard Luick
10,955 PointsRichard Luick
10,955 PointsOkay so in addition to adding the activity to the manifest, here is my CrystalBall.java code
The app now launches on my phone but crashes as soon as I shake it to get an answer. I am not sure what the issue is. Does it have something to do with me having two onCreate methods? I already had one in my MainActivity. Would it be better practice to put this code there?
John McDonald
13,958 PointsJohn McDonald
13,958 PointsCan you add the manifest code as well?
Richard Luick
10,955 PointsRichard Luick
10,955 PointsJohn McDonald
13,958 PointsJohn McDonald
13,958 PointsI'm sorry, I thought that might have fixed your problem but apparently not :(
Richard Luick
10,955 PointsRichard Luick
10,955 PointsIts okay I appreciate the help! After thinking about it, It seems to make sense to me that this code would get the job done. Maybe someone else has some insight on why it crashes when I shake the phone.