Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial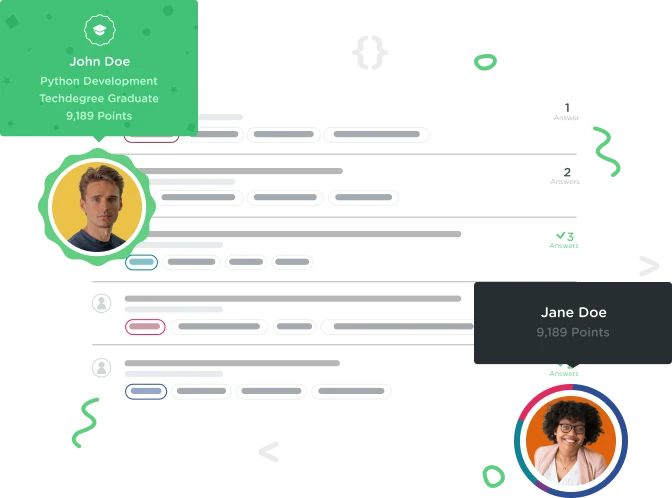
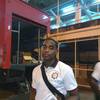
Isaac John
7,516 Pointsstring factory test
dicts = [
{'name': 'Michelangelo',
'food': 'PIZZA'},
{'name': 'Garfield',
'food': 'lasanga'},
{'name': 'Walter',
'food': 'pancakes'},
{'name': 'Galactus',
'food': 'worlds'}
]
string = "Hi, I'm {name} and I love to eat {food}!"
def string_factory(dicts, string):
dicts_copy = dicts[:]
final_list = ""
counter = 0
while True:
if counter == len(dicts):
return final_list.split()
else:
for key in dicts:
new_dict = key
new_string = "Hi, I'm {name} and I love to eat {food}!".format(**new_dict)
final_list = final_list + new_string
counter = counter +1
continue
When I run this code I get "Didn't get all results" or something like that...But when I run it in Workspaces it prints out every sentence:
['Hi,', "I'm", 'Michelangelo', 'and', 'I', 'love', 'to', 'eat', 'PIZZA!Hi,', "I'm", 'Garfield', 'and', 'I', 'love', 'to', 'eat', 'lasanga!Hi,', "I'm", 'Walter', 'and', 'I', 'love', 'to', 'eat', 'pancakes!Hi,', "I'm", 'Galactus', 'and', 'I', 'love', 'to', 'eat', 'worlds!']
I'm not exactly sure what it's supposes to look like
Update!
def string_factory(dicts, string):
final_list = []
for key in dicts:
temp_dict = key
del key
my_string = "Hi, I'm {name} and I love to eat {food}!".format(**temp_dict)
final_list = final_list + list(my_string)
return final_list
I tried the above code which gives me the same error in test but prints:
['H', 'i', ',', ' ', 'I', "'", 'm', ' ', 'M', 'i', 'c', 'h', 'e', 'l', 'a', 'n', 'g', 'e', 'l', 'o', ' ', 'a', 'n', 'd', ' ', 'I', ' ', 'l', 'o', 'v', 'e', ' ', 't', 'o', ' ', 'e', 'a', 't', ' ', 'P', 'I', 'Z', 'Z', 'A', '!', 'H', 'i', ',', ' ', 'I', "'", 'm', ' ', 'G', 'a', 'r', 'f', 'i', 'e', 'l', 'd', ' ', 'a', 'n', 'd', ' ', 'I', ' ', 'l', 'o', 'v', 'e', ' ', 't', 'o', ' ', 'e', 'a', 't', ' ', 'l', 'a', 's', 'a', 'n', 'g', 'a', '!', 'H', 'i', ',', ' ', 'I', "'", 'm', ' ', 'W', 'a', 'l', 't', 'e', 'r', ' ', 'a', 'n', 'd', ' ', 'I', ' ', 'l', 'o', 'v', 'e', ' ', 't', 'o', ' ', 'e', 'a', 't', ' ', 'p', 'a', 'n', 'c', 'a', 'k', 'e', 's', '!', 'H', 'i', ',', ' ', 'I', "'", 'm', ' ', 'G', 'a', 'l', 'a', 'c', 't', 'u', 's', ' ', 'a', 'n', 'd', ' ', 'I', ' ', 'l', 'o', 'v', 'e', ' ', 't', 'o', ' ', 'e', 'a', 't', ' ', 'w', 'o', 'r', 'l', 'd', 's', '!']
sorry for the long post
2 Answers
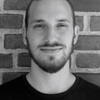
Neil Andruski
21,509 PointsI see whats happening here. What they are looking for is a result that looks like with the variables filled in.
["Hi, I'm {name} and I love to eat {food}",
"Hi, I'm {name} and I love to eat {food}",
"Hi, I'm {name} and I love to eat {food}",
etc]
But when you use .split() it makes a list with each word as its own item. You want to keep it as a full sentence. You have some good ideas but they are getting implemented in the wrong spots and some extra work. This was my code. There is still some other ways you could add the formatted string to the list but this was the what I came up with.
def string_factory(dicts, string):
#make a blank list I can add each sentence to
result = []
#loop through each pair of {name,food} in dicts and set them to key
for key in dicts:
#add the sentence once formatted using the variables from the string
result.append(string.format(**key))
#finally return the list with all the sentences
return result
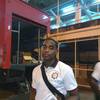
Isaac John
7,516 PointsWow thanks a lot! :D Look how simple it is...wow...I'm going to look over this and try to understand everything.
I actually tried using append but when I did it was saying something about you can't append a string to a list but i guess it was probably how i did it. Your comments in between code proved very useful
thanks again!!!
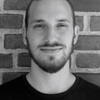
Neil Andruski
21,509 PointsYeah, I added in the comments to help you through my process and I feel like Kenneth covered a similar example in the video right before this problem. I always re-watch the videos to help me if I get stuck. Really your last fix was 2-3 lines edits away from working. Just remember the Key is already a duplicate so no need to delete it and last was adding to your final_list
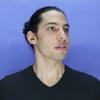
Alonso Serrano
15,341 PointsThanks! I knew I was on the right track! I tried a very similar solution but it didn't work.
def string_factory(dicts,string):
new_list=[]
for key in dicts:
string.format(**key)
new_list.append(string)
return new_list
Apparently, the format method doesn't actually modify the string, so you can't call it later and expect the changes to be there.

Tyson Pyles
1,398 PointsI have been using all of your examples as the foundation for trying to understand this section but have been failing miserable.
How would you then call this function?

Adam Fields
Full Stack JavaScript Techdegree Graduate 37,838 PointsTyson I was struggling with this one too, it's not the most well-written challenge question. If you're still stuck, this should get you un-stuck:
"""
1. only pass in the dicts argument (template is a global variable, you don't need to pass it in)
2. they want a list of strings for a result, so make an empty list
3. dicts is a list, each item is a dictionary that you want to pass to the format method and unpack
4. append the formatted string to the list each loop
5. return the list
"""
def string_factory(dicts):
array = []
for item in dicts:
array.append(template.format(**item))
return array

Anders Axelsen
3,471 PointsHi Adam, this clarification of your first statement, concering template as a global value, made the final understanding come clear for me. Thanks for chipping in.

Adam Fields
Full Stack JavaScript Techdegree Graduate 37,838 PointsMy pleasure Anders Axelsen . What's funny is that I haven't touched Python since March, and when I first saw this, I was like, "Hmmm, that's some strange looking comments and JavaScript."
Neil Andruski
21,509 PointsNeil Andruski
21,509 PointsWhile I was working on my answer I saw your update and you were getting there man. Keep up your hard work.