Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial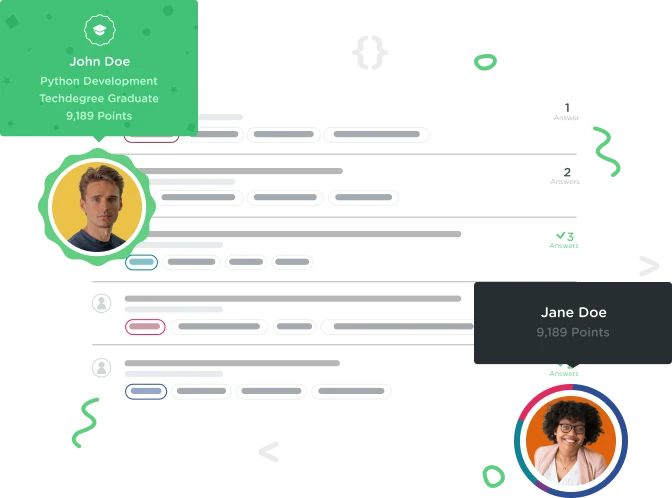

Nayoon kim
3,098 PointsString.contains ();
import java.io.Console;
public class TreeStory {
public static void main(String[] args) {
Console console = System.console();
String age = console.readLine("Enter your age: ");
int age_int = Integer.parseInt (age);
if (age_int<13) {
console.printf("you must be over 13 to use this program\br");
System.exit(0);}
String name= console.readLine ("Enter a name: " );
String adjective;
String [] notallowed;
boolean sensorship;
do {
adjective= console.readLine("Enter an adjective: " );
sensorship = adjective.contains (notallowed);
notallowed = {"dorky", "jerk" , "dumb", "bitchy"};
if (notallowed.contains(1,2,3);) {
console.printf ("that is not very nice word to use , try nicer adjective. try agin \n\n" );}
} while (notallowed.contains(1,2,3););
String noun= console.readLine ("Enter a noun: " );
String adverb= console.readLine ("Enter an adverb: " );
String verb= console.readLine ("Enter a verb: " );
}
}
this didn't work. as well .. I feel frustrated a bit
1 Answer

Yanuar Prakoso
15,196 PointsHi Nayoon
The main problem on your code is you set notallowed as array of strings. However, Java documentation denotes that inside contains can only read Char Sequences. The main point here String is also a sequence of chars which means you should set notallowed variable as String. Then you need to change the {"dorky", "jerk" , "dumb", "bitchy"} which denotes array of Strings to just Strings : String notallowed = "dorky jerk dumb bitchy";
Next the problem in your do while loop. First one is you use adjective variable as reference to examine if its contains notallowed, however it should be the other way around: you should use sensorship = (notallowed.contains(adjective.toLowerCase()));
Please note that I use toLowerCase method to change all characters in adjective String since contains method is case sensitive.
The next problem is the IF statement. I failed to understand what do you mean by ** if (notallowed.contains(1,2,3);)** since this will get you nowhere. You should test if sensorship is true or false.
This is how I code:
import java.io.Console;
public class TreeStory {
public static void main(String[] args) {
Console console = System.console();
String age = console.readLine("Enter your age: ");
int age_int = Integer.parseInt (age);
if (age_int<13) {
console.printf("you must be over 13 to use this program\br");
System.exit(0);}
//this is where I modify the code
String name= console.readLine ("Enter a name: " );
String adjective;
String notallowed = "dorky jerk dumb bitchy";
boolean sensorship;
do {
adjective= console.readLine("Enter an adjective: " );
sensorship = (notallowed.contains(adjective.toLowerCase()));
if (sensorship) {
console.printf ("that is not very nice word to use , try nicer adjective. try agin \n\n" );}
} while (sensorship);
//this is the end of my modification of your code
String noun= console.readLine ("Enter a noun: " );
String adverb= console.readLine ("Enter an adverb: " );
String verb= console.readLine ("Enter a verb: " );
}
}
I hope this can help a little. Since I am modifying your code not in the work space console I have not test it yet. Please test it and give me feedback if the code is still does not work. Thank you
Nayoon kim
3,098 PointsNayoon kim
3,098 Pointsomg thank you so much , it is just that I have little knowledge in Java. So I did not know how to make string array.. I've only just started. thank you lots ! it really helped me
kevin hudson
Courses Plus Student 11,987 Pointskevin hudson
Courses Plus Student 11,987 PointsYeah, I think Nayoon was trying to reference array indexes (1,2,3) which would have left out dorky since that was array[0]. But yes it should be from a String object
https://docs.oracle.com/javase/7/docs/api/java/lang/String.html ---> search "contains"