Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial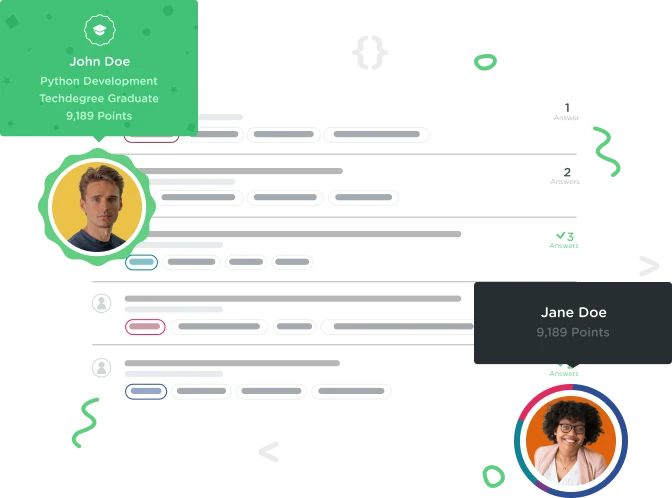

Ankush Jain
2,800 Pointsstring_factory
what am I doing wrong here ?
dicts = [
{'name': 'Michelangelo',
'food': 'PIZZA'},
{'name': 'Garfield',
'food': 'lasanga'},
{'name': 'Walter',
'food': 'pancakes'},
{'name': 'Galactus',
'food': 'worlds'}
]
string = "Hi, I'm {name} and I love to eat {food}!"
def string_factory(dicts, string):
strings = []
for k in dicts
strings.append(string.format(k['name'],k['food']))
return strings
5 Answers

Daniel Johnson
104,132 PointsJust answered this question for you, but if you want to do it a little differently you could also do it like this.
def string_factory(dicts, string):
string_list = []
for dict in dicts:
string_list.append(string.format(name=dict['name'], food=dict['food']))
return string_list
...but you could also do it this way.
def string_factory(dicts, string):
string_list = []
for dict in dicts:
string_list.append(string.format(**dict))
return string_list
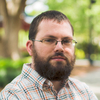
Kenneth Love
Treehouse Guest TeacherWhen placeholders have names (like {name}
), you have to give named arguments to .format()
, like .format(name="foo")
.

Mike Tribe
4,114 Pointsdef string_factory(dict_list, a_string):
my_list = []
while dict_list:
for k in dict_list:
my_list.append(string.format(**k))
return(my_list)
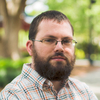
Kenneth Love
Treehouse Guest TeacherThat while
is going to run forever (because dict_list
isn't being changed so won't ever become false-y) and you don't need it anyway. The rest of your code is great.

Mike Tribe
4,114 PointsThanks Kenneth, feedback is great and enjoying the course. For some reason I find some of the code challenges run differently than expected in the given environment than in IDLE for example; I couldn't get this to work properly without an extra loop. Daniel's second example is the best i think; with 47k+ points, I guess he knows what he's doing :-)
Is the "dict" a keyword or something? "For dict in dicts" seems to work reliably. I just guessed that the Python interpreter would just "grab" the next nested dict until it reached the last one inside the container dict that was passed to the function; thanks for clarifying.
Sometimes I get more success writing the code challenge functions in IDLE and pasting them into the code challenge window.
Python does seem a great way to begin coding and doing interesting stuff quickly. If I can just understand using tuples properly......
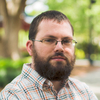
Kenneth Love
Treehouse Guest Teacherdict
is a built-in class (it's what's called when you do dict([('a', 1), ('b', 2)])
or {'a': 1, 'b': 2}
) so it's best to avoid it as a variable name.

Mike Tribe
4,114 PointsAh makes sense, I've just been thinking in terms of "format" of data, I guess: " ' ' ", [], {}, Strings, Lists and Dicts.... etc
It is ok to use "dict" in a for-loop is it, "For dict in dicts" ?
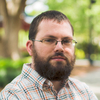
Kenneth Love
Treehouse Guest TeacherSure but you'll have the same problem as outside of the for loop.