Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial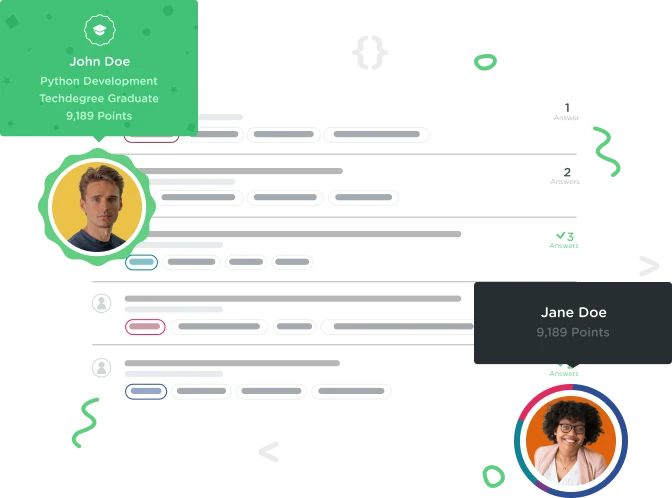

Simon Goldman
1,028 PointsString_interpolation
im stuck on method returns part 2, cant figure out how to write it in a sentance form.
def mod(a, b)
a % b = c
puts "The remainder of #{a} divided #{b} is #{c}."
end
1 Answer

andren
28,558 PointsThere are two ways to do it, your solution is pretty close to the first way, but there are a couple of mistakes.
In the first line of your function. "a % b = c" should be "c = a % b". Unlike in math equations it actually matters quite a bit what goes on the left and right side of the equals sign when you are doing assignments in programming languages.
The challenge wants you to return the string, not print it with puts. Those are very different things. Returning a value is done by adding the return keyword before the thing you want to return (there is a caveat to this that I'll detail below).
The code challenges are pretty picky about the fact that text that is printed or returned has to be exactly what the challenge expects it to be. Even the smallest of typos will often trip the challenge checker. In your string you forgot to write "by" before the "#{b}" part of the string.
If you fix those mistakes you will end up with this code:
def mod(a, b)
c = a % b
return "The remainder of #{a} divided by #{b} is #{c}."
end
Which will allow you to complete the challenge.
There is also a shorter solution where you don't create a c variable, instead you do the a % b equation directly in the string interpolation stage like this:
def mod(a, b)
"The remainder of #{a} divided by #{b} is #{a % b}."
end
You might also notice that I don't use the return keyword in the above example, that is because of the caveat I alluded to earlier. In most programming languages you have to explicitly return a value by using the return keyword. But in ruby there is a concept called "implicit return" which means that ruby will always return the value of the last statement it executed, even if you do not use the return keyword.
Because of that if the value you want to return is the last line of your function then you don't need to type return. Though I personally tend to explicitly type return anyway, mostly because I'm used to programming in other languages where that is necessary. Getting into the habit of typing it explicitly is probably not a bad idea if you ever intend to learn another programming language.