Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial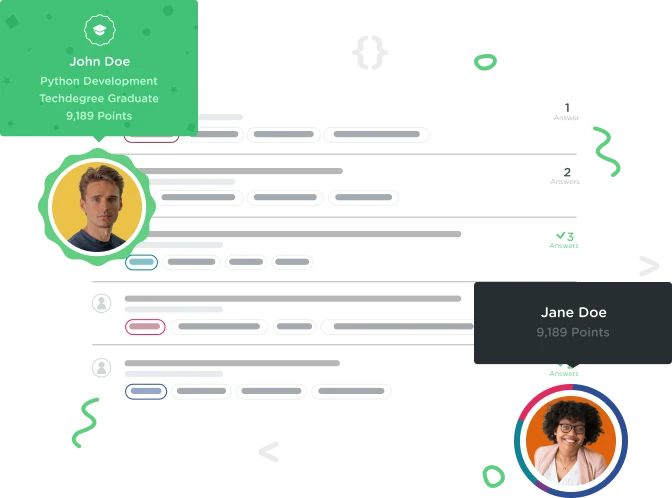
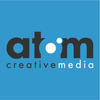
Alex Connor
2,809 PointsStruct broken down... I think. Please correct and collaborate!
Like many people who've reached this stage, I've been having some difficulty getting my head around what's happening in this video, even after watching a number of times.
I've added comments to each line of the struct and the method within, in the hopes that it might a) help others, and b) encourage corrections and collaborations from others as well.
So here's my understanding of what's happening:
struct Point {
let x: Int // these *stored properties* have no value assigned to them - that comes later
let y: Int // their job is to name the constant, and define its type.
/// Returns the surrounding points in range of
/// the current one
func points(inRange range: Int = 1) -> [Point] { // Here we are creating a new function within the struct, with a default value of 1
// We have then specified the return type as being an array of Point instances
var results = [Point]() // Here we declare a new variable of type Point, and assign an empty array. We'll eventually assign
// coordinates to this array. It's a variable because we need to mutate it throughout this process.
let lowerBoundOfXRange = x - range // get the lower bound numbers of the origin x point and assign them to a constant
let upperBoundOfXRange = x + range // get the upper bound numbers of the origin x point and assign them to a constant
let lowerBoundOfYRange = y - range // get the lower bound numbers of the origin y point and assign them to a constant
let upperBoundOfYRange = y + range // get the upper bound numbers of the origin y point and assign them to a constant
/*
now we start a loop which will go through all of the x values and y values and create a new constant containing the values based on the custom type Point. this constant is then added (appended) to the above "results" variable, and the loop continues until all points have been collected and added to the results array.
*/
for xCoordinate in lowerBoundOfXRange...upperBoundOfXRange {
for yCoordinate in lowerBoundOfYRange...upperBoundOfYRange {
let coordinatePoint = Point(x: xCoordinate, y: yCoordinate)
results.append(coordinatePoint)
}
}
return results // finally, the function returns the results array ready for calling back later
}
}
let playerPosition = Point(x: 1, y: 2) // so we have to create a new instance of the Point type, declarun the object's position
let enemyPosition = Point(x: 4, y: 4) // each time we want to use this struct and method within
playerPosition.points() // now we can simply call the points method each time we want to get the surrounding points
enemyPosition.points() // for that instance
enemyPosition.points(inRange: 3) // We can also define a custom range, instead of using the default
Hopefully this helps somebody out! Like I said, I'd welcome corrections and invite others to collaborate on this in order to improve it and help people understand what's happening in this video.
:)
SivaKumar Kataru
2,386 PointsSivaKumar Kataru
2,386 PointsWell Written!