Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial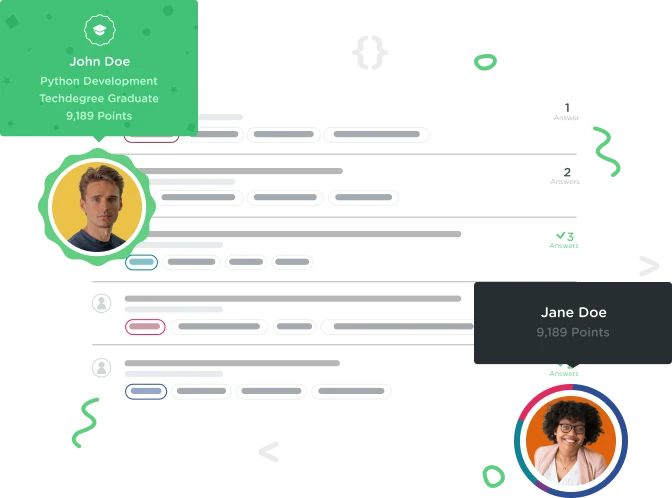

Steven Ossorio
1,706 PointsStruct, Func, Class and Init. The difference between the 3
I've watched the videos and I've read up on it in apple developer library but I'm still confused on when each should be used. Can someone help explain the difference or when it'll be appropriate to use them.
Does Class have a similar role to what is found in RoR. Thank you everyone who helps me understand how to use them correctly when coding.
1 Answer
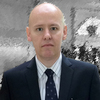
Nathan Tallack
22,159 PointsFirstly, value types and refrence types.
An int and a struct are value types. That means when you creating them you are refrencing the acutal values in memory. And when you copy them around (that is reassign them to another value type) you are creating new copies of them in memory. If you make changes to the original the new one does not change.
A class is a refrence type. This means when you are creating them you are refrencing an address in memory where the object is stored. When you copy it around you are copying that refrence to that address which means you are not creating new copies of that object you are just creating mutliple "copies" of them you are just copying the refrence to the same object. If you make changes to the original you make changes to the new one.
Additionally, an Int stores just one value. A struct and a class can store multiple values (called properties) and can store code (functions inside of these objects are called methods) that let you interact with that object and its properties.
Finally, a class can be subclassed, meaning you can create a subclass that has all of the properties and methods of a parent class inside of it. You cannot do that with a struct.
Many many other things, but that will do for now. That's the most important stuff. :)
Steven Ossorio
1,706 PointsSteven Ossorio
1,706 Points//Just to repeat what you've told me. Please fix me if I'm wrong (hoping I got it though).
//Int and Struct are able to create copies. In that sense, an example would be:
/*Though not wise. We can add it to other methods such as let worldBestPizza = newPizzaRecipe etc This isn't a smart idea since it'll get too long to reference the recipe you're trying to change. The original also stays the same without it being affected */
print(\(Recipe.firstStep)
Make sure the flower is spread across the tray.
//Please let me know if I made a mistake somewhere. Also when we use Int, it would be conventional when we are //using a single value. An example would be:
// Against please let me know if I'm off in my understanding. I'm sorry also for the long reply to my question. // Last is class, much like I've shown thus far. This one will change the original method if I were to modify it
// Thank you again for your explanation and final check up to my understanding of my question.