Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial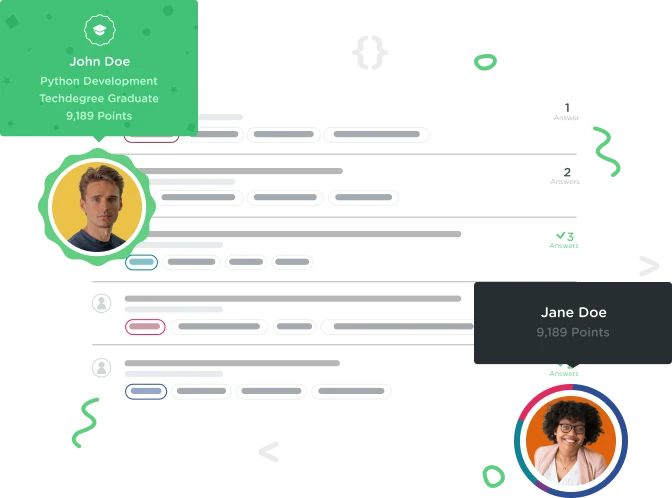

Cody Adkins
7,260 Pointsstruct not conforming to type protocol
I keep getting an error message in Xcode when I try making my struct type a protocol i made earlier in the video. The error is at about 1:30 minutes into the video. Can someone explain what I should do to fix this?
2 Answers
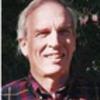
jcorum
71,830 PointsYou are making price and quantity Double arrays, not Doubles. Try this:
protocol ItemType {
var price: Double {get} //not [Double]
var quantity: Double {get set} //not [Double]
}
struct VendingItem: ItemType {
let price: Double
var quantity: Double
}
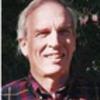
jcorum
71,830 PointsSwift protocols are like interfaces in Java. If you say a class or struct will conform to a protocol you are saying it will implement whatever the protocol requires. In the video the ItemType protocol has two variables: price and quantity. So any struct that conforms to this protocol must have those two properties, as Pasan's VendingItem struct does (1:26).

Cody Adkins
7,260 PointsHere is the protocol I have written, with the struct that is not conforming...
protocol ItemType { var price: [Double] {get} var quantity: [Double] {get set} }
struct VendingItem: ItemType { let price: Double var quantity: Double }
I am still getting error...
John Perry
Full Stack JavaScript Techdegree Graduate 41,012 PointsJohn Perry
Full Stack JavaScript Techdegree Graduate 41,012 PointsIn the previous video, Pasan switched from adding code to the
ItemType
to adding code to theVendingMachineType
in a way that was easy to miss, especially if you were typing as he was talking. So, if you did what I did, you added code to the wrong area. Make sure thatVendingMachineType
andItemType
look like this: