Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial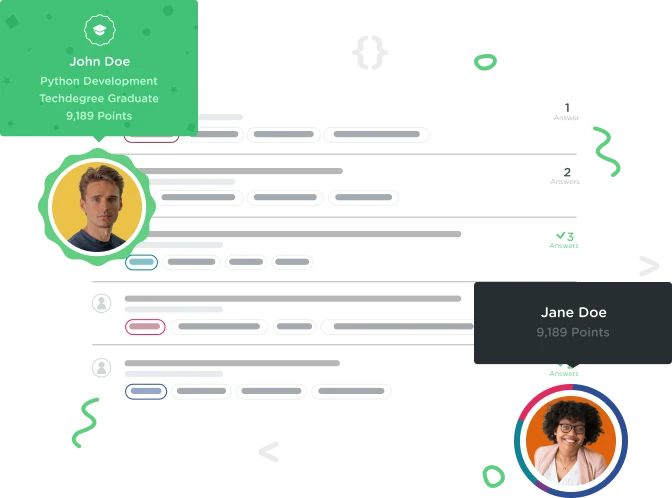

Alia Khan
3,733 PointsStructs challenge
Stuck on this challenge if anyone could help and explain it as well Thank you :) Alia
class Point {
var x: Int
var y: Int
init(x: Int, y: Int){
self.x = x
self.y = y
}
}
class Machine {
var location: Point
init() {
self.location = Point(x: 0, y: 0)
}
func move(direction: String) {
print("Do nothing! Im a machine!")
}
}
// Enter your code below
class Robot: Machine {
overide func move(direction: String) {
print("Do nothing! Im a machine!")
}
2 Answers

Jessica Hunter
Full Stack JavaScript Techdegree Student 13,097 PointsHey Alia!
Hope this helps:
This challenge wants you to override the "move" function so that instead of simply printing text, it will cause the x or y positions of Robot to change. (At the moment, your Robot's 'move' function is identical to the move code inside the Machine class.)
As a refresher, the challenge directions for the override function are:
"
If you enter the string "Up" the y coordinate (of the Robot's location) increases by 1.
"Down" decreases it by 1.
If you enter "Left", the x coordinate (of the location property) decreases by 1
while "Right" increases it by 1.
Note: If you use a switch statement you can use the break statement in the default clause to exit the current iteration.
"
Okay, now to get your code working!
First: The word 'override' in your function is misspelled. There should be two r's. That happens to me too =)
Next: Inside your Robot's move function, you need to create a switch statement. Note: The statement for the switch should be the parameter, 'direction', because it is the Robot's direction ("Up", "Down", "Left", "Right") that we will be checking. So far, the code would look something like this:
override func move(direction: String){
switch direction {
//your code here
}
}
Next, inside your switch: Each 'case' will represent one of the strings listed in the challenge directions ("Up", "Down", "Left", "Right"):
switch direction {
case "Up": //code to increase y by 1
case "Down": //code to decrease y by 1
case "Left": //code to decrease x by 1
case "Right": //code to increase x by 1
(As the challenge directions state, don't forget to add, default: break, here at the end)
}
A note on the cases above:
To increase x or y you can use the shorthand operator += 1
To access Robot's y location, you will need to use dot notion: self.location.y
(The video on switch statements is a good reference for syntax: https://teamtreehouse.com/library/swift-20-collections-and-control-flow/control-flow-with-conditional-statements/switch-statements )
I apologize for the formatting, it got a little jumbled together when I hit post, but I hope this walkthrough has helped!
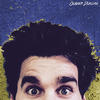
Oliver Duncan
16,642 PointsA robot is a machine that moves. To make the robot move, override move() by adjusting its location.
For example, if we call move("North") on an instance of the robot class, the robot's y position needs to increase by one. If we call move("West"), its X position decreases by one, and so on.
Alia Khan
3,733 PointsAlia Khan
3,733 PointsHi Jessica thank you for your help I've tried to do what uv said but its not working for some reason. could you please write out the whole code so i can compare it to mine and see where i've gone wrong. I appreciate your help
Thanks :)
Jessica Hunter
Full Stack JavaScript Techdegree Student 13,097 PointsJessica Hunter
Full Stack JavaScript Techdegree Student 13,097 Pointsclass Robot: Machine {
}