Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial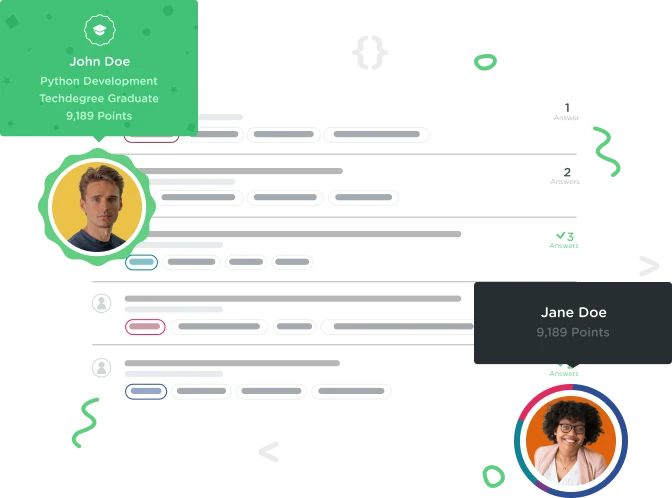

Mikkel Storch
1,955 PointsStructs into an array
Hello. I was trying to make a program to search through some products i made with a struct. I was wondering if its possible to program, something that every time i create an instance of a struct, the instance is automatically put into an array? So i can search through all the instances of the struct via the array. This is my code so far:
struct Products {
let title: String
let price: Double
}
let quadcopter = Products(title: "Quadcopter", price: 499.99)
let iPhone6 = Products(title: "iPhone6", price: 999.99)
let keyboard = Products(title: "Keyboard", price: 49.99)
let horse = Products(title: "Horse", price: 7999.99)
let dog = Products(title: "Dog", price: 399.99)
4 Answers

miguelcastro2
Courses Plus Student 6,573 PointsHere is an example of using a factory class to create the Product struct and using an inout variable to pass an array to append the product to:
struct Product {
let title: String
let price: Double
init (title: String, price: Double) {
self.title = title
self.price = price
}
}
class ProductFactory {
class func createProduct(title: String, price: Double, inout productsArr: [Product]) -> Product {
let product = Product(title: title, price: price)
productsArr.append(product)
return product
}
}
var productsArr = [Product]()
var quadCopter = ProductFactory.createProduct("Quadcopter", price: 499.99, productsArr: &productsArr)
var iPhone = ProductFactory.createProduct("iPhone", price: 699.99, productsArr: &productsArr)
for product in productsArr {
println(product.title)
}

Bradley Weston
Courses Plus Student 360 PointsThis is called lazy and no language supports this functionality.
You're not going to get what you asked for unless you made some sort of factory for creating Products
instances via a method on the factory and then in the factories logic put it onto a collection you can retrieve at a later date.

miguelcastro2
Courses Plus Student 6,573 PointsI completely agree with your assessment.

Mikkel Storch
1,955 PointsCompletely disagree, whether it's possible or not. It's much smarter and time efficient if this method was possible, it has nothing to do with laziness. Its just like saying that you are lazy because you drive your car to work instead of walking. Its TIME EFFICIENT...

Bradley Weston
Courses Plus Student 360 PointsWell magic is never good, being verbose is. Out of all the language I've used I've come across such behavior.
However I can drive, but I do bike to work ;)

miguelcastro2
Courses Plus Student 6,573 PointsInitialize an empty array of Products and then add your individual instances:
let productsArray = [Products]()
let quadcopter = Products(title: "Quadcopter", price: 499.99)
// Append to array
productsArray.append(quadcopter)

Mikkel Storch
1,955 PointsYea, this i know. But as i wrote, i need it to be done automatically, so I don't need to write the .append statement for every new product I make. You know a solution for this?
But thanks anyway! ;-)
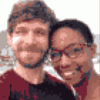
Chris Nash
Courses Plus Student 1,402 PointsHey man, cool problem! I could see many uses for this. Here's how I got it to work in playground using a designated initializer:
struct Products {
let title: String
let price: Double
init (title: String, age: Double) {
self.title = title
self.age = age
arrayProducts.append(self)
}
}
let arrayProducts = [Products]()
let quadcopter = Products(title: "Quadcopter", price: 499.99)
let iPhone6 = Products(title: "iPhone6", price: 999.99)
let keyboard = Products(title: "Keyboard", price: 49.99)
let horse = Products(title: "Horse", price: 7999.99)
let dog = Products(title: "Dog", price: 399.99)

Bradley Weston
Courses Plus Student 360 PointsCould you elaborate on the usages? I'm interested. Also that arrayProducts
is now global? Global variables are bad practice.
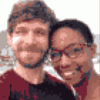
Chris Nash
Courses Plus Student 1,402 PointsThanks for the article, it inspired a morning of learning about local vs global variables in Swift. As far as I can tell, if you declare a variable outside of a method, function, etc. then it is global, which allows any part of the program to mess with it, including 3rd party plugins and users that are clever enough to find a crack in your system.
Is fixing this global problem as easy as this:
//fixed "age" vs "price" typo -- whoops!
//added listProducts struct to house arrayProducts.
struct Products {
let title: String
let price: Double
init (title: String, price: Double) {
self.title = title
self.price= price
listProducts.arrayProducts.append(self)
}
}
struct listProducts {
let arrayProducts = [Products]()
}
let quadcopter = Products(title: "Quadcopter", price: 499.99)
let iPhone6 = Products(title: "iPhone6", price: 999.99)
let keyboard = Products(title: "Keyboard", price: 49.99)
let horse = Products(title: "Horse", price: 7999.99)
let dog = Products(title: "Dog", price: 399.99)
What are some uses you see for this? As someone who is pretty much brand new to programming, I'd like to hear an experienced programmer's thoughts first.

Bradley Weston
Courses Plus Student 360 PointsFirstly global variables are bad in all programming languages.
Well, programming has been my full-time job for the past 5 years. I've never expected this behavior at all. However the suggestion I used above it about the only nice way of doing it. Doing it your way means ALL instances are appended to the the variable arrayProducts
. Where as having a factory that you'd specifically called would contain the logic for this as it is a separate concern (Single Responsibility Pattern). The Products
should not be concerned about how it is stored this is up to the user/factory initializing the instance.