Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial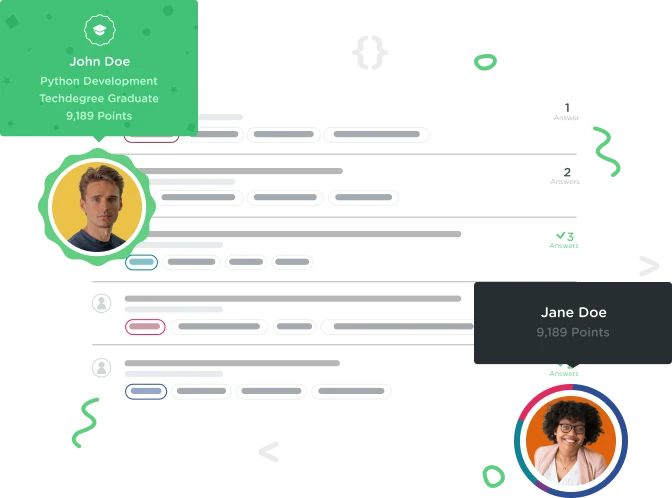

walterb
972 PointsStruggling to get While loop to work on this one
I'm struggling to get the while loop to work on this code challenge. When I run the code without the while loop, it work fine and tells me if the random number is even or odd. When I add my while loop structure, the program does nothing. Can anyone point me the right direction here?
import random
start = 5
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
while start is True:
num = random.randint(1, 99)
if even_odd(num) is True:
print("{} is even".format(num))
else:
print("{} is odd".format(num))
start -= 1
1 Answer
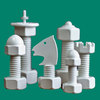
Steven Parker
241,808 PointsIn Python, "is" is an identity comparison. It only matches if both sides refer to the same thing (not just have the same value).
An equality comparison is the double equal sign operator ("=="). But you don't need that here, since "start" is a number it will never be equal to "True" (a boolean).
You can test a value for "truthiness" just by naming it:
while start:
Similarly, you can test the result of calling "even_odd" directly also.
walterb
972 Pointswalterb
972 PointsThat helps, thanks!