Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial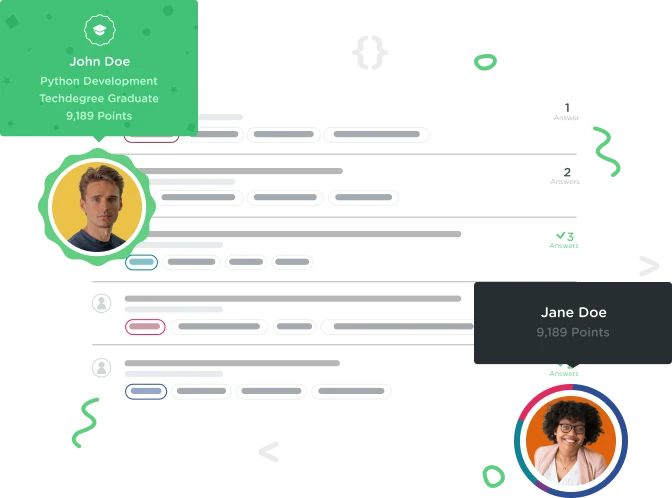

barryhatfield
3,001 PointsStruggling to set up discount permissions in form_valid method
I've gone through the docs, but am not sure how to implement has_perm()
from django.core.urlresolvers import reverse
from django.db import models
class Product(models.Model):
name = models.CharField(max_length=255)
description = models.TextField()
price = models.DecimalField()
discount = models.DecimalField(blank=True, null=True)
class Meta:
permissions = (
('can_give_discount','gives discount'),
)
def __str__(self):
return self.name
def get_absolute_url(self):
return reverse("products:detail", kwargs={"pk": self.pk})
from django.contrib.auth.mixins import LoginRequiredMixin
from django.views import generic
from djando.contrib.auth import has_perm
from . import models
class List(generic.ListView):
model = models.Product
class Detail(generic.DetailView):
model = models.Product
class Create(LoginRequiredMixin, generic.CreateView):
fields = ("name", "description", "discount", "price")
model = models.Product
def form_valid(self, form):
user = self.request.user
if not user.has_perm("products.can_give_discount"):
self.object.discount = 0
self.object.save()
return super(form_valid, self).form_valid(form)
2 Answers
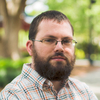
Kenneth Love
Treehouse Guest TeacherYour code looks like it should pass once you fix the indentation.
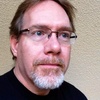
Chris Freeman
Treehouse Moderator 68,457 PointsHey Barry Hatfield, there are two errors in your code:
Before the
if
statement, define the self.object
:
self.object = form.save(commit=False) # <-- create self.object to modify
Call the parent class from
super()
not the method:
return super(Create, self).form_valid(form) # <-- refer to the parent class
Otherwise, your code will pass. Post back if you need more help. Good Luck!!
barryhatfield
3,001 Pointsbarryhatfield
3,001 PointsStill no Joy: Manually added every space as I didn't want to trust the tab button. Am I indenting something incorrectly?
[MOD: added ```python formatting -cf]