Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial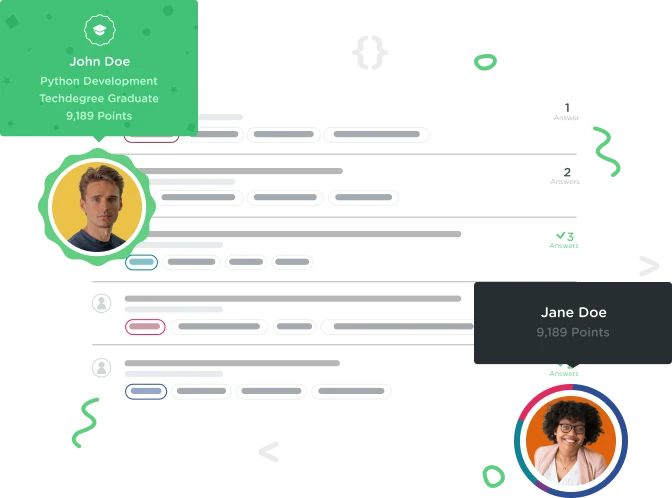

Shannon McKinney
5,079 PointsStruggling with Errors in Random Number Challenge
I am trying to complete the Random Number Challenge without looking at the solution video, and I can't figure out why my code isn't working correctly. I've played around with it by placing the min/max definitions & parseInt outside of the function and inside the function. Outside the function, I get a NaN, but when coded according to the following, the random number I get is not actually inside the range I want it to be.
Any help is much appreciated. I want to understand what I'm doing wrong just as much as I want to understand the right way to do it. Thanks!
function randomNumber(min, max) {
min = prompt("Please input an integer.");
parseInt(min);
max = prompt("Please input another integer higher than the first.");
parseInt(max);
var randomNumber = Math.floor(Math.random() * (max - min + 1)) + min;
document.write(randomNumber);
}
randomNumber();
4 Answers
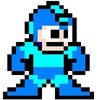
Robert Richey
Courses Plus Student 16,352 PointsAwesome job!
Here is some feedback, if you like. Since your function contains all of the code, you could remove the parameters. (you're not calling the function with any arguments). Here are two ways you could re-write it, depending if you want function parameters or not.
Note: I changed the name of the function to rng()
, because it feels very strange (if not potentially buggy) to have both a function and variable with the same name.
// with parameters
function rng(min, max) {
return Math.floor(Math.random() * (max - min + 1)) + min;
}
var min = parseInt(prompt("Please input an integer.");
var max = parseInt(prompt("Please input another integer higher than the first.");
var randomNumber = rng(min, max);
document.write(randomNumber);
// without parameters
function rng() {
var min = parseInt(prompt("Please input an integer.");
var max = parseInt(prompt("Please input another integer higher than the first.");
var randomNumber = Math.floor(Math.random() * (max - min + 1)) + min;
document.write(randomNumber);
}
rng();
Cheers
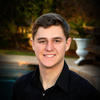
Colton Ehrman
Courses Plus Student 5,859 PointsSo parseInt() takes two paremters the first is the thing you want to parse, and the second is the number format you want to parse it into, in this case you want base 10 so just have the second parameter be 10.
And recheck your random number calculations

Shannon McKinney
5,079 Points(ETA: I think the parsing is not happening at the right place. Going to try playing around with variables for a minute. Below is what I initially replied with)
Thank you. I'm not sure if I've learned about the number format bit yet. Do you know of how I might fix this without using the number format aspect to parsing? I did try adding 10 as the second parameter, and I'm still experiencing an issue of, say, entering numbers 1 (min) and 5 (max) and returning a value of 21 or even 01.
Regarding random number calculations, I've checked it against the code used in a previous video, and I can't figure out what might be wrong with mine. This was the code he used in a previous lesson:
var input1 = prompt("Please type a starting number.");
var bottomNumber = parseInt(input1);
var input = prompt ("Please type a number");
var topNumber = parseInt(input);
var randomNumber = Math.floor( Math.random() * (topNumber - bottomNumber + 1) ) + bottomNumber;
var message = "<p>" + randomNumber + " is a random number between 1 and " + topNumber + ".</p>";
document.write(message);

Iain Simmons
Treehouse Moderator 32,305 PointsThe second parameter/argument for parseInt
is optional (though recommended). If you don't pass the second (radix) argument, then it defaults to 10.
The actual issue was that Shannon McKinney wasn't assigning the results of the parseInt
function to a usable variable, since it returns a new integer, it doesn't change the type or value of the variable passed to it as the first argument.

Shannon McKinney
5,079 PointsOK, I think I fixed it! Seems to be working now. Here's what I did:
function randomNumber(min, max) {
input1 = prompt("Please input an integer.");
min = parseInt(input1);
input2 = prompt("Please input another integer higher than the first.");
max = parseInt(input2);
var randomNumber = Math.floor(Math.random() * (max - min + 1)) + min;
document.write(randomNumber);
}
randomNumber();
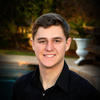
Colton Ehrman
Courses Plus Student 5,859 PointsSo use this for calculationing your random number
Math.floor(Math.random() * max) + 1;
And do parseInt like this
parseInt(num, 10);

Iain Simmons
Treehouse Moderator 32,305 PointsThat calculation is only good for a range of 1 to max
, not min
to max
.
Iain Simmons
Treehouse Moderator 32,305 PointsIain Simmons
Treehouse Moderator 32,305 PointsNo issues with a variable within a function sharing the same name as the function, aside from being confusing. It only becomes an issue if you try and define both a function and a variable with the same name in the same scope. In that case, the one coming second will overwrite the first.