Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial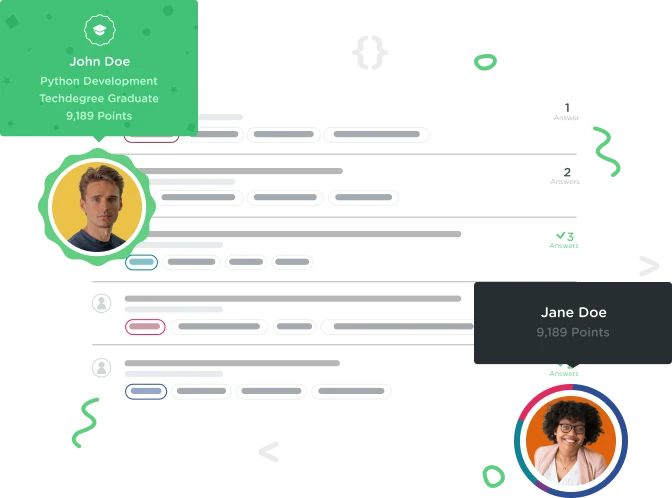

Mark Bradshaw
Courses Plus Student 7,658 PointsStruggling with functions in Objective - C. Need assistance.
Here's the question: Implement a function named "addTwo" that returns the sum of two floats. The function will accept two float numbers as arguments. It should add the two arguments together, and return the result. (No need to write the main function. Just write out the implementation for the addTwo function.)
My answer:
int addTwo(float a, float b){
float a = 1;
float b = 2;
return sum = float a + float b;
}
Not sure if needed to add the detail before the implementation wheere I indicated the value of a and b.
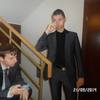
Aurelian Spodarec
7,369 PointsOr if you find hard to understand the video , what helped me , is when a moderator changed my code and i looked what he/she did .
You can also press edit and see what gloria did in your post :) hope this helps too .
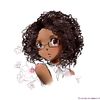
Gloria Dwomoh
13,116 PointsThat's a smart approach Aurelian ^^ Thanks for sharing, I never thought of that.
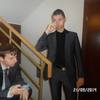
Aurelian Spodarec
7,369 PointsYes. On iMac , its a little bit different actually . The keyboard is slightly different than the windows one . The dash or whatever is that sign named , is on the bottom , not the top . I had trouble with that , so for new iMac users it would be helpful to tell them where about it is on the keyboard too.
I actually waited ages for a moderator to put my code like it should be, the video on the right , never worked , i mean there was some problem with it right ? i don't know .
Plus now we have amazon guide how to do different things such as and that makes BIG difference in writing in my opinion. But what i though . Lets face it , there are millions of people using this and every time someone is new , some people just never come on forum or once in a while and you know . Moderators can help to change the way they use bullet points , code etc..
But i think they should make something like for names where you use @ to tag people like gloria , see i tagged you :D
same as they could make something on forum like a command that would be easier for people to understand and help them put the code next time as it will take long time and even may not be able to get the code in place as its a ruby program e.g. all squished up . In Xcode , theres a good way to do it were it sort the text for you :)
So it would be nice if they would write like " Want to put the code on forum?" write e.g. @codeHTML and then this transforms to the ``` like it supposed . Or they could just add on the right small thing how to post your code on forum .
Also for me the title " Tip for asking questions " you know , a tip xd depends who what thinks.
What do you think ? xd i wrote like paragraphs again for uh this xd i like to write xd
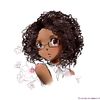
Gloria Dwomoh
13,116 PointsHi again Aurelian, we have some helpful posts on that as well. Initially it was hard for me to figure out where the backticks are but eventually I found it. These posts Posting Code to the Forum and How-to Guide: Markdown within Posts, show most if not all the cool tricks you can do to format your questions or replies in the forum. There is also a Markdown cheatsheet that appears below the reply box when writing a reply to someone. You can have a look at those as well. Those help a lot ^_^
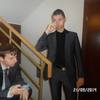
Aurelian Spodarec
7,369 PointsHi
Oo i never saw this https://teamtreehouse.com/forum/posting-code-to-the-forum but its helpful . I saw the other one with is really helpful . Thats a good knowledge sharing and all :D
bdw im strugling to find this sign # on my iMac keyboard. I have no idea where it is .
I got it from Xcode as for import so i just copied it and paste as i use it once in a while there but now as i want to make a webpage layout , i don't want to copy and paste all the time # . Do you know where could it be?
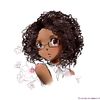
Gloria Dwomoh
13,116 PointsHold down option and press 3. I hope this helps.
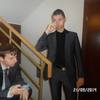
Aurelian Spodarec
7,369 Pointsuh , xd thank you :D pretty much the same as on windows : p only that here there is like cmd , alt , ctrl , fn , etc.. just messed up : p . Thank you very much :) Your doing a great work helping others :)
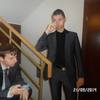
Aurelian Spodarec
7,369 PointsHi gloria ,
I have a request about begin a moderator but I'm not sure who to accept it. I read all they said on the pdf moderator file .
Should i just reply to them ?
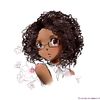
Gloria Dwomoh
13,116 PointsAurelian Spodarec , yes reply to that message with your response.
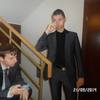
Aurelian Spodarec
7,369 PointsThank you :)
Hi again : p
gloria , I'm just wondering did you wait a bit for their reply ? or they don't read or do anything on weekend? I'm just curious because its like i don't like of having my state of mind saying something and then something else happens . Im not into rush or anything , but every time i wrote to teamtreehouse , when i started , i never had any reply at all , so I'm just wondering .
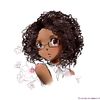
Gloria Dwomoh
13,116 PointsAurelian Spodarec , I see you got a reply :) that happens because the working days are Monday though Thursday, that's why there was a delay.
1 Answer
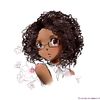
Gloria Dwomoh
13,116 PointsSince you are taking float arguments in your function, you will probably get a float after the addition so it will be good to set your return type to float. You have written the code below...I will write some comments on it for some errors
int addTwo(float a, float b){
/*the numbers below are not actual "floats", those are integers (but the compiler accepts them, and recognizes them as floats because it assumes their floating point is followed with zeros. Floats should have a floating point ie. 1.3, 2.456, 5.1
, the compiler considers the number 2 for example as the floating values 2.0, 2.0000 and so on as, if you see the output the instructor gets you'll see the floating values of those numbers appearing, but it is good to know that floats have a floating part*/
float a = 1;
float b = 2;
return sum = float a + float b;
}
the problem with this code is... it does not return the given argument...as in the function you initialize new float arguments. So if I call your code with...
addTwo(1.3, 5.6);
that will be passed on to your function ...so what will happen by the compiler will look somehow like this..
int addTwo(1.3, 5.6){
float a = 1; //Here you create a new variable
float b = 2;
return sum = float a + float b; // it will return 1 + 2 and not 1.3 + 5.6 as we want.
}
so the code has to change and be able to accept any given float number. A function that is able to do that is one like this
//This is the correct answer for the question
float addTwo(float num1, float num2);
return num1 + num2;
}
so now if I try to call
addTwo(1.3, 5.6);
the compiler will do something like this ...
float addTwo(1.3, 5.6){
return 1.3 + 5.6 ; //which is 6.9
}
which is what we want :) I hope this explains things better.

Mark Bradshaw
Courses Plus Student 7,658 PointsThis makes sense. I realize that I wasn't using the correct version of a float number, and that I didn't need to add the additional variables, but I still can't get through the question. I've just submitted the implementation code.
float addTwo(car1.1, car2.2){
return 1.1 + 2.2;
}
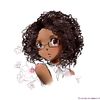
Gloria Dwomoh
13,116 PointsHi Mark, the numbers I added in the function are for you to understand how it works but you don't have to use numbers. That happens by the compliler. It replaces the place holders you have put with numbers. In this case what you need is.
float addTwo(float num1, float num2){
return num1 + num2;
}
If you call that with
addTwo(1.1, 1.2)
the compiler will then replace num1 with 1.1 and num2 with 1.2 and return to you their sum. :) That will work for any type of float numbers you use as num1 and num2 are placeholders that get replaced with the values you pass to them.
Ps. addTwo(car1.1, car2.2)
car1.1 and car2.2 is considered as an invalid variable and not a number
EDIT: I also edited my code a bit because I forgot to note that num1/num2 is a float

Mark Bradshaw
Courses Plus Student 7,658 PointsYour help is much appreciated Gloria. Your explanation is implementation portion of the function right?
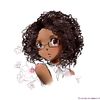
Gloria Dwomoh
13,116 PointsYes Mark, basically what I did with the number...is do what a compiler will do with the function call, replace the placeholders with the numbers I gave in the function call. I have now specified it is somehow the way a compiler will act on those parts of the code where I am explaining the implementation, to make things clear. I hope this was helpful :)

Mark Bradshaw
Courses Plus Student 7,658 PointsThanks for all of the assistance Gloria. Your explanation finally clicked for me. Cheers.
Gloria Dwomoh
13,116 PointsGloria Dwomoh
13,116 PointsI fixed your code so it appears better. If you'll like to know how I did it, you can check the video on the right.