Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial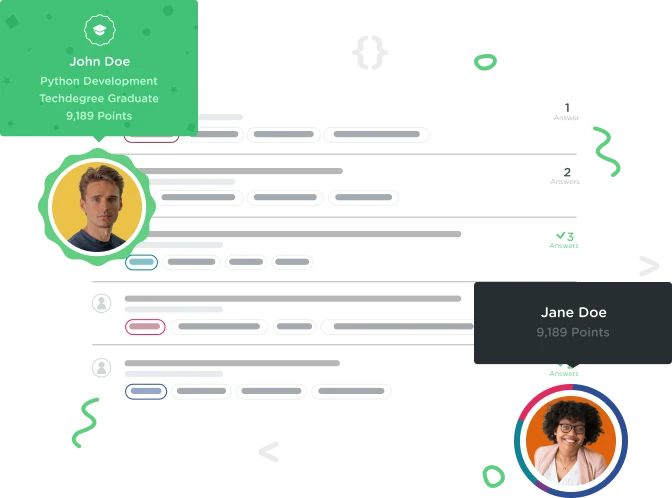

Mohammed Ali
10,625 PointsStruggling with Javascript Unit Testing 'Code Challenge: Catching an Error'
Not sure what to do here. I get the following error:
Bummer! We tried your spec with a version of subtraction.js that works correctly, but the specs didn't pass!
Here is the solution I came to:
var expect = require('chai').expect
describe('subtraction', function () {
var subtraction = require('../WHEREVER')
it('only works with numbers', function () {
var result = subtraction('string', false);
expect(result).to.not.be.a('number');
});
})
var expect = require('chai').expect
describe('subtraction', function () {
var subtraction = require('../WHEREVER')
it('only works with numbers', function () {
var result = subtraction('string', false);
expect(result).to.not.be.a('number');
});
})
function subtraction (number1, number2) {
if (typeof number1 !== 'number' || typeof number2 !== 'number') {
throw Error('subtraction only works with numbers!')
}
return number1 - number2
}
3 Answers

Neil McPartlin
14,662 PointsHi Mohammed. I found this quite tough too. I had only done this course a few months back, I must have passed the challenge back then but this time, I struggled so much, I copied the challenge to my computer so that I could experiment freely without suffering that 'Bummer' message. It was good that I did.
The first thing to mention is a key sentence in their Challenge...
Make sure to check for subtraction's specific error message!
So we need to be checking for the specific text ''subtraction only works with numbers!', and we should witness this by specifically ensuring one of the 2 arguments provided to the subtraction function is not a number.
So testing 'offline', I proved to myself that the subtraction worked correctly when provided with 2 numbers.
describe('subtraction', function () {
var subtraction = require('../WHEREVER')
it('can correctly subtract 1 from 3', function () {
expect (subtraction(3, 1)).to.equal(2);
})
})
The above resulted with...
subtraction
√ can correctly subtract 1 from 3
So after a great deal of reading, I replaced one number with a letter and expected the following to work...
describe('subtraction', function () {
it('it should state "subtraction only works with numbers!"', function () {
expect (subtraction('a', 1)).to.throw('subtraction only works with numbers!');
})
})
But not so... :(
1) subtraction
it should state "subtraction only works with numbers!":
Error: subtraction only works with numbers!
at Error (native)
at subtraction (subtraction.js:3:13)
at Context.<anonymous> (subtraction_spec.js:27:17)
I watched Guil's previous video and sure enough, at around 6:42 he states...
So it looks like the throw method requires me to wrap my function in something that Chai can handle internally. Now, if I didn't do this, then my test spec would always fail, because placeShip will throw an error. Mocha will think that error counts as a test failure, even though the error is really what I expect.
So I just had to wrap the subtraction function inside another function, so as not to confuse Chai like so...
describe('subtraction', function () {
it('it will state "subtraction only works with numbers!"'', function () {
var handler = function () {
subtraction('a', 1);
}
expect (handler).to.throw('subtraction only works with numbers!');
})
})
So back online, to solve the Treehouse challenge, we just need to replace // YOUR CODE HERE with...
var handler = function () {
subtraction('a', 1);
}
expect (handler).to.throw('subtraction only works with numbers!');

Julianna Kahn
20,702 PointsThank you for being so helpful and so thorough. I guess I overlooked the handler function. A great lesson.

Harald N
15,843 PointsThanks Neil!
I tried and tried, first try was with a handler function and all, but never got it to work and without a console to read messages it's really hard to understand what you are doing wrong.
Turnes out teamtreehouse parses or maybe Chai doesn't like arrow functions, that was my mistake. Used the handler function with a normal function declaration and it passed. In case someone has the same problem with using an arrow function.
Mohammed Ali
10,625 PointsMohammed Ali
10,625 PointsAmazing. What a great and thorough answer. Thank you.
Neil McPartlin
14,662 PointsNeil McPartlin
14,662 PointsYou're welcome Mohammed. Having taken the 'scenic route' trying to get to the answer, I felt I might as well 'take some photos' along the way, and share with others. :)