Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial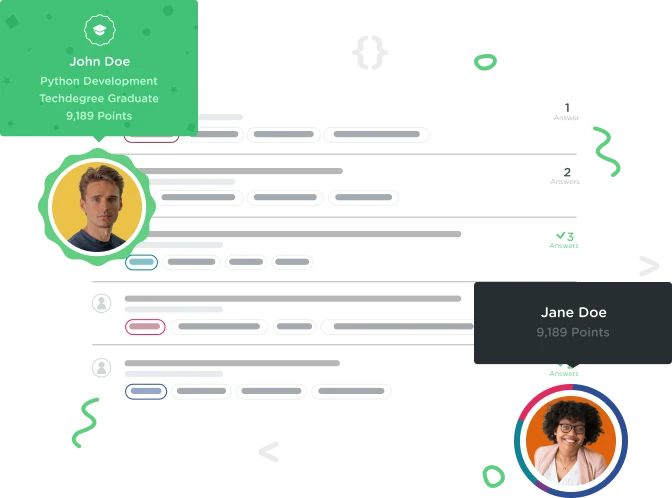
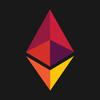
Richard Verbraak
7,739 PointsStruggling with OOP and this Challenge
So I did the OOP course and it went okay, took me a long time for me to understand these things and sometimes I still get confused. Especially when it comes to using the this keyword.
But man this challenge is really hard. I feel like it doesn't really complement the pretty small course about OOP in JavaScript since there are a lot of new things shown here. It gets quite complex (for me atleast) and I'm beginning to think I missed a whole course on OOP.
For example: Setting a due date of 14 days with the date object. That wasn't really touched on last course. When comparing my code to the solution in the tutorial there are glaring mistakes so can anyone help me clarify these things a bit?
I feel like OOP isn't a thing that's learned in 2 days either, or maybe I'm just not focusing hard enough.
My code:
class Patron {
constructor(name, email){
this.name = name;
this.email = email;
this.currentBook = null;
}
checkOut(book) {
this.currentBook = book;
this.out = true;
this.patron = new Patron();
this.dueDate = new Date().setDate(14);
}
returnBook(book) {
this.currentBook = null;
this.out = false;
this.patron = null;
this.dueDate = null;
}
}
The solution:
class Patron {
constructor(name, email){
this.name = name;
this.email = email;
this.currentBook = null;
}
checkOut(book) {
this.currentBook = book;
book.out = true;
book.patron = this;
const newDueDate = new Date();
newDueDate.setDate(newDueDate.getDate() + 14);
book.dueDate = newDueDate;
}
returnBook(book) {
this.currentBook = null;
book.out = false;
book.patron = null;
book.dueDate = null;
}
}
I would greatly appreciate any help.
2 Answers
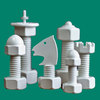
Steven Parker
231,275 PointsI think the concept of "this" often takes a while to fully grasp, you're quite normal in this regard! Have you seen this workshop: Understanding "this" in JavaScript?
It looks like most of the differences in the two examples above have to do with where the properties are located. If you think about what each class represents it helps to make sense of it.
These are all methods of the Patron. If a patron checks out or returns a book, it makes sense to change the "out" status of the book (book.out
), but the patron doesn't need an "out" status (this.out
) since we don't track whether they are in the library or not.
Similarly, it makes sense to update the book record with the patron checking it out (book.patron = this
). But checking out a book wouldn't cause the patron to be associated with another (and brand new) patron (this.patron = new Patron()
).
Does thinking of it that way help to make it clear?
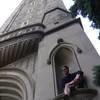
Michael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsHey Richard, I know it's been some time now since you posted this. How are things going now? Do you feel as though you understand OOP better? I did the JS OOP course and this course after spending a lot of time learning OOP in PHP and learning to build backend applications. I realized that when I first started learning OOP it was crazy confusing, but now this course seems incredibly simple to me. I am sure you feel similarly now. I actually think that part of the problem that students have is that instructors try to simplify OOP too much with concrete examples that really don't do much to illuminate the concepts. OOP isn't really about modeling real-world objects so much as it is just organizing related functionality together into containers. Thinking about OOP as modeling the real world confuses me, because there is a lot of ambiguity and places where the metaphors break down. But when I think about object-oriented programming as just an approach where variables and functions that depend on each other get grouped together, suddenly it doesn't seem so hard. I think to understand it you just have to embrace the abstraction of it. Anyway I hope things are going better for you now.
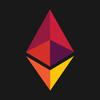
Richard Verbraak
7,739 PointsHey Michael,
Yes it's been awhile haha. I've been doing a LOT better now, especially when I look back on this post I made a few months ago. Shows you that nothing is impossible and you'll understand it eventually, it just takes time. I found that reading/watching different sources on the same subject makes it so much easier. Every instructor has his own examples or way of explaining things, some click, some don't.
I semi-agree on the real-world objects metaphor not being a great example of OOP but at the same time it does make a little bit of sense. Specifically the car/dog example is better than most examples, it has functions like bark or drive and properties like it's race or brand. The only problem is they just put too much emphasis on those examples instead of just saying that it's a group of key/value pairs (the properties) with methods that modify the data that is attributed to said class instance. Or even simpler, a class has and modifies data that is related to it.
Anyhow, thank you for your comment, it's great to look back every once in awhile. I hope you're doing good as well! I'll follow your progress on GitHub! If you have any question regarding JavaScript by the way, you can ask me if you like.
Richard Verbraak
7,739 PointsRichard Verbraak
7,739 PointsAh, I haven't seen that workshop before so I will definitely check that out. Now, I understand where I went wrong with my thinking with:
this.out vs book.out
The this keyword I used in my solution was referring to the Patron object and not the book parameter in checkOut? That's what I thought this was referring to in my solution.
I don't get the
book.patron = this
part at all though, could you maybe clarify what's going on here? I will check out the course in an hour or so and will probably get back to this thread with hopefully a better understanding of what you explained. Thanks for clearing some parts up, it's kicking my ass atm.Steven Parker
231,275 PointsSteven Parker
231,275 PointsThroughout the patron class methods, "this" refers to the patron instance which the method was (will be) called on. So "
book.patron = this
" assigns the current patron instance to the "patron" property inside the book that is being checked out.So later, if the book object is examined, you can tell that is checked out (
out = true
) and who has it (patron
).Richard Verbraak
7,739 PointsRichard Verbraak
7,739 PointsSo I got around to watching the Workshop and I get it now (I think), thanks for notifying me. I think I should've learned more about the this keyword first before continueing. It confused me a lot and wasn't able to focus on much of the OOP course as a result.
Just to be clear of my understanding. This is solely based on context and when this is used in a method, that's inside of an object, it refers to the class you made. Then you can further access it's properties with dot notation.
So why can't I use the class's name instead of using this? Like book.patron = Patron; I know it's probably invalid syntax but why not use that instead of the confusing keyword?
Steven Parker
231,275 PointsSteven Parker
231,275 Points"Patron" is the class, you might think of a class as a "blueprint" for making an object. But "this" is a reference to a specific object, which happens to be an instance of that class.
Richard Verbraak
7,739 PointsRichard Verbraak
7,739 PointsAhh, that took me a lot longer to learn then I initially wanted to but atleast I get it now. Thanks a lot!
Steven Parker
231,275 PointsSteven Parker
231,275 PointsAs I said, this concept often takes a bit to "sink in". It sounds like you're doing great!
Happy coding!
i1276
7,626 Pointsi1276
7,626 PointsUnfortunately that "Understanding "this" in JavaScript" workshop is gone :(
Steven Parker
231,275 PointsSteven Parker
231,275 PointsOdd for that to be retired but not updated, since it's a concept that can often need some reinforcement. You might try making an appeal for bringing it back to the Support folks.
In the meantime, you might take a look at what some of the many free learning resources online have to say about "this", for examples:
Joe Elliot
5,330 PointsJoe Elliot
5,330 PointsHey guys, is the "this" workshop still available? The link is broken and I can't find it when searching on the treehouse website,
Darrel Valdiviezo
Full Stack JavaScript Techdegree Student 13,473 PointsDarrel Valdiviezo
Full Stack JavaScript Techdegree Student 13,473 PointsHey Steven Parker , how about the utilizing this.currentBook for the out and patron property. It' s confusing because it is reference a book obj and specifically that same one "book" is?
Steven Parker
231,275 PointsSteven Parker
231,275 PointsYou're right that it represents the currently checked-out book. But why is this confusing?