Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial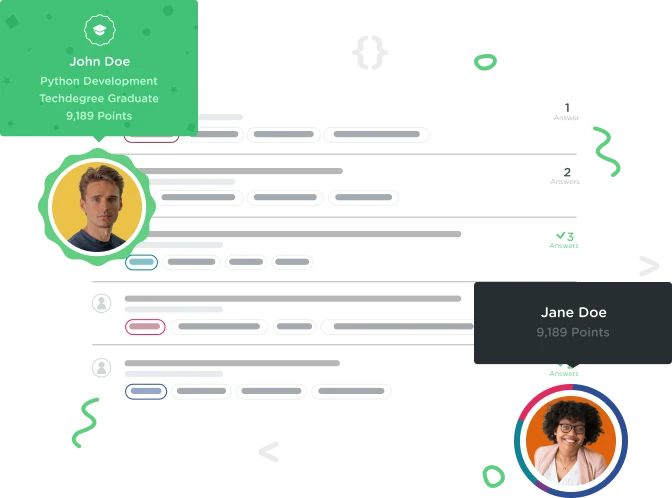
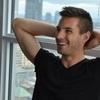
evanpavan
5,025 PointsStruggling with the code challenge.
I'm having a tough time in the first exercise of the code challenge stubbing out the actual classes from the given tests.
Right now I'm getting a compilation error that my constructor can't have the same name as it's enclosing class.
public class Calculator
{
public double intialValue;
//constructor related to the Initialization test method
public double Calculator(double x)
{
intialValue = x;
}
//adding method related to the BasicAdd method
public double Add(double y)
{
//adding adds the value passed to the add method to the value the calculator was initialized with
double total;
total = y + initialValue;
return total;
}
}
using Xunit;
public class CalculatorTests
{
[Fact]
public void Initialization()
{
var expected = 1.1;
var target = new Calculator(1.1);
Assert.Equal(expected, target.Result, 1);
}
[Fact]
public void BasicAdd()
{
var target = new Calculator(1.1);
target.Add(2.2);
var expected = 3.3;
Assert.Equal(expected, target.Result, 1);
}
}

Alan Brown
20,524 PointsRemove the return type on your constructor.
1 Answer
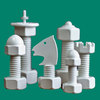
Steven Parker
230,688 PointsI see three issues:
- "initialValue" is spelled "intialValue" in two places
- constructors should not have a return type (not even "void")
- the class to be tested must have a "Result" property or field
evanpavan
5,025 Pointsevanpavan
5,025 PointsSteven Parker I've recreated the question with my code. Thanks for the tips of this.