Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial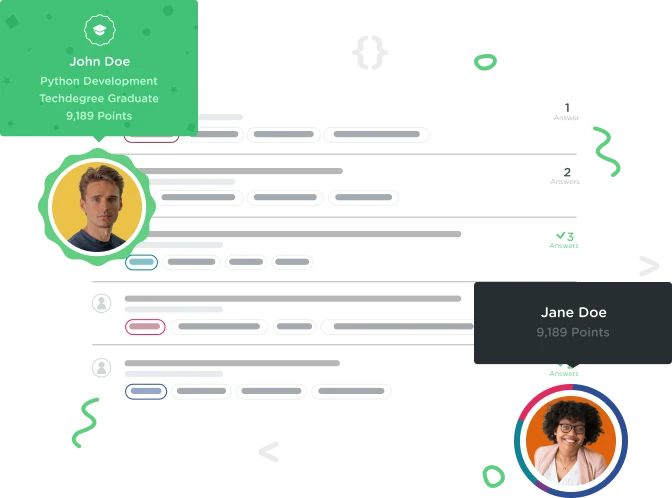

Shaun Williams
1,986 PointsStruggling With the getTileCount Challenge
Hey Guys,
I'm struggling to pass the ScrabblePlayer "getTileCount" Challenge.
I keep getting the error message: Bummer! Did you forget to create the method getTileCount that accepts a char?
Where am I going wrong? Any help would be great!
Here is my code (see getTileCount method at the bottom):
public class ScrabblePlayer {
private String mHand;
public ScrabblePlayer() {
mHand = "";
}
public String getHand() {
return mHand;
}
public void addTile(char tile) {
// Adds the tile to the hand of the player
mHand += tile;
}
public boolean hasTile(char tile) {
return mHand.indexOf(tile) > -1;
}
public String getTileCount() {
String counter = "";
for (char tile: mHand.toCharArray()) {
char display = tile++;
if (mHand.indexOf(tile) >= 0) {
display = tile;
}
counter += display;
}
return counter;
}
}
4 Answers
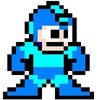
Robert Richey
Courses Plus Student 16,352 PointsAdding to Patrick's answer, here's another hint:
Did you forget to create the method getTileCount that accepts a char?
// this method signature is accepting a char
public boolean hasTile(char tile)
// your getTileCount method signature is not accepting a char
public String getTileCount()
I'll also point out that the return value for getTileCount should be a number (what data type can we use to represent a number?).
Here's some pseudo-code that may help
/*
getTileCount(char tile) {
initialize a counter variable to 0
for each letter in mHands
if letter and tile match
increment counter
return counter
}
*/
Please let me know if this helps or not.
Cheers

Michael C
3,230 PointsIf somebody says something about "accepting" and "methods" in one sentence I always think about "What kind of data type (char, integer, string etc.) does this method actually wants as an argument (or some say parameter)".
As you know printf() does need a String. So if the Bummer tells you if you created a method that "accepts a char" you might realize that the error message wants to point to the question if you actually created a method that awaits a parameter/argument.
As you can see in your method's parantheses you did not put any parameter of type char in there.

Shaun Williams
1,986 PointsThanks so much for the reply, Patrick.
I originally had:
public char getTileCount() { char counter = '-';
However, I received a ton of compile issues and that really threw me.
I'm still struggling to wrap my head around it. Any hints on what I can do from there?
Thanks

Shaun Williams
1,986 PointsHey Robert,
Got it! Thanks so much for the help, you're a life saver. I'm relying on Google a lot, and struggling to know when to apply certain concepts, but I'll get there with practice.
Thanks again :)
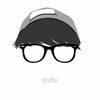
kevin nisay
1,159 Pointshi guys im just starting to learn how to program using java ,its been a year for this comment, guys can you give me some advice ? im struggling a lot , i would like to ask is it fine to look around some codes and answer ? can i learn by doing that ?
Shaun Williams
1,986 PointsShaun Williams
1,986 PointsHey Robert,
Really appreciate the reply! That's a big help, I understand it now.
I think I'm almost there, but getting the following error: "Bummer! The hand was "sreclhak" and 'e' was checked. Expected 1 but got 844."
How could it be getting 844 instead of 1?
Robert Richey
Courses Plus Student 16,352 PointsRobert Richey
Courses Plus Student 16,352 PointsYou're doing great! These challenges can be really tricky sometimes. Here are some more hints. Please let me know how you're doing after this.