Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial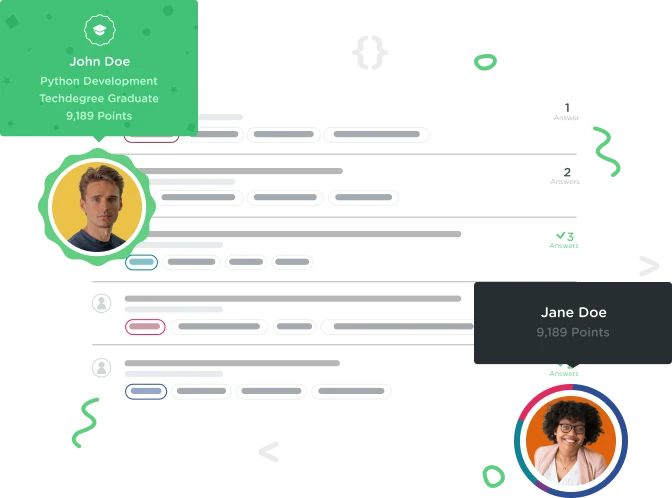

Dawson Young
512 PointsStuck
Keep getting stuck, I think it's because I don't really understand all parts of the task at hand. Maybe because of the way it is written lol idk. Any tips on this challenge?
let numbers = [2,8,1,16,4,3,9]
var sum = 0
var counter = 0
// Enter your code below
while sum <= 7{
print(numbers)
sum++
}
sum += numbers[counter]
3 Answers
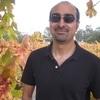
Kourosh Raeen
23,733 PointsThe loop condition should use the variable counter. Also, use the count property to get the size of the array, but use the less than symbol since arrays are zero indexed, meaning the last item in the array is at index 6 not 7. You also need to have the code that does the summation inside the loop. Try the following code:
while counter < numbers.count {
print(numbers)
sum += numbers[counter]
counter++
}

Dawson Young
512 PointsThe top half of my code works, but the bottom part starting at "sum += numbers[counter]" does not
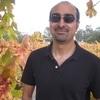
Kourosh Raeen
23,733 PointsIf you look at the instructions again it reads that "The while loop should continue as long as the value of counter is less than the number of items in the array", so instead of
while sum <= 7
you need to either use
counter < 7
or
counter < numbers.count
As for your last line of code, you need to have that inside the loop so that in each iteration of the loop you add one of the numbers in the array to the sum and by the time the loop is done sum contains the sum of all the numbers in the array.
Also, there is no need for that print statement. I forgot to delete it.

Dawson Young
512 PointsI understand that part, but the second task of this code challenge is " Now that we have the while loop set up, it's time to compute the sum! Using the value of counter as an index value, retrieve each value from the array and add it to the value of sum.
For example: sum = sum + newValue. Or you could use the compound addition operator sum += newValue where newValue is the value retrieved from the array."... That is the part I am stuck on
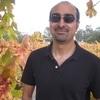
Kourosh Raeen
23,733 PointsBut we are already doing the second task. The lines:
sum += numbers[counter]
counter++
are computing the sum. When the loop first starts, the value of counter is zero so numbers[counter] is numbers[0] which is the first entry in the array and that is 2. So adding that to sum, which is zero at this point, we end up with sum holding the value 2. Then counter ++ increments the value of counter by one so it is now 1, which means that numbers[counter] is now numbers[1], which is the second entry in the array and that is 8. Now the line:
sum += numbers[counter]
adds 8 to sum, which at this point is 2 so the new value of sum is 10 now. Continuing in the same fashion, in the last iteration of the loop the value of counter is 6 so numbers[counter] is numbers[6] which is the last entry in the array and that is 9. Again the line:
sum += numbers[counter]
adds 9 to the value of sum at this stage, which is 34, so now sum hold the value 43. Then the line counter++ changes the value of counter to 7 which make the condition in the while loop false, because 7 is not less than 7, so the loop terminates and we have computed the sum.
Also, remember that the line:
sum += numbers[counter]
is exactly the same as:
sum = sum + numbers[counter]