Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial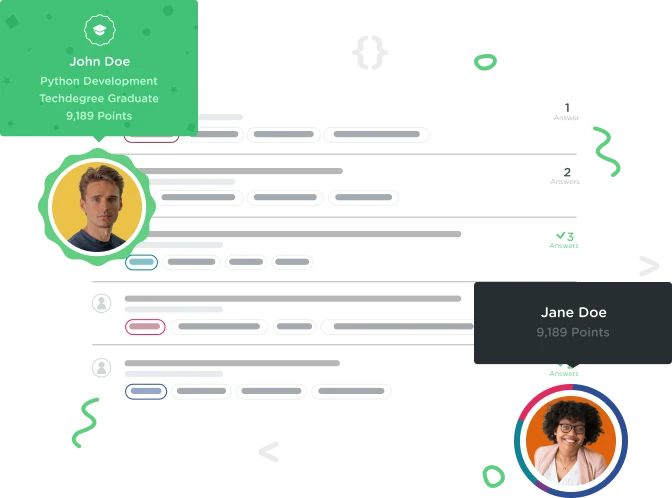

Halcyon Abraham Ramirez
846 Pointsstuck at combos challenge task 1 :/
how exactly do we do this?
been tearing my hair out solving it for 2 days now :/
# combo(['swallow', 'snake', 'parrot'], 'abc')
# Output:
# [('swallow', 'a'), ('snake', 'b'), ('parrot', 'c')]
# If you use list.append(), you'll want to pass it a tuple of new values.
# Using enumerate() here can save you a variable or two.
def combo(x,y):
x = list(x)
y = list(y)
wow = dict(zip(x,y))
for key, values in wow.items():
print("{},{}".format(key, values))
print(["hello","hi","sup"], "abc")
1 Answer
William Li
Courses Plus Student 26,868 PointsHello, Halcyon Abraham Ramirez , the answer to this problem doesn't have to be so complex. I see that your code made use of the zip
function in Python; if we're gonna approach this code challenge with zip, the solution is one-liner.
def combo(x, y):
return list(zip(x, y))
Halcyon Abraham Ramirez
846 PointsHalcyon Abraham Ramirez
846 Pointswithout using zip how exactly do we solve it?
I know im just really confused and my answer above spits out the wrong answer too :/
so without using zip what is the solution?
William Li
Courses Plus Student 26,868 PointsWilliam Li
Courses Plus Student 26,868 PointsPerhaps zip isn't how we should approach this code challenge, because I don't remember Kenneth ever mentioned about zip during the lecture; however, since you wrote your code using zip, I assumed you've done some research on the topic
Here's an alternate solution, without using zip. The problem description gave us 2 pieces of information.
So we are going to use enumerate() here.
That makes our jobs so much easier; we only need to iterate through one list, and using the index value to iterate through the other list as well.
Halcyon Abraham Ramirez
846 PointsHalcyon Abraham Ramirez
846 PointsWhoa so you basically enumerated through the x and use the index variable on x to y to get the the corresponding letter of y for that index? wow that genius
thank you very much just wow
William Li
Courses Plus Student 26,868 PointsWilliam Li
Courses Plus Student 26,868 Pointsno problem, I'm glad that you find it helpful.