Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial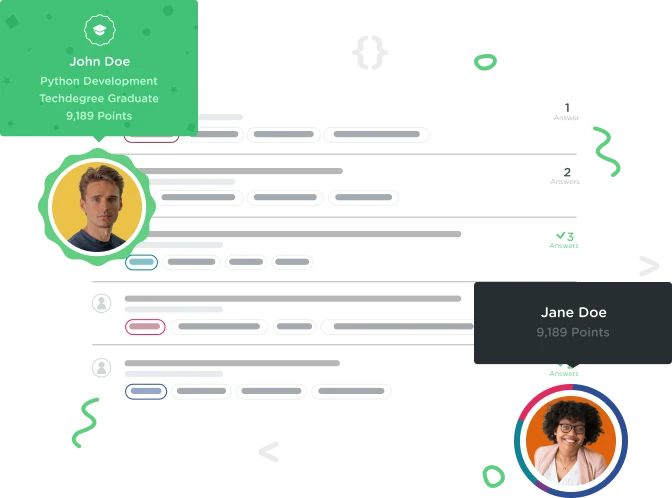

Greg Stone
2,705 PointsStuck. Getting error 'Cannot convert return of type "String" to return type "(Double, Double). How do I convert?
Stuck. Getting error 'Cannot convert return of type "String" to return type "(Double, Double). How do I convert?
// Enter your code below
func getTowerCoordinates (location: String) -> (Double, Double) {
switch location {
case "Eiffel Tower": 48.8582; 2.2945
case "Great Pyramid": 29.9792; 31.1344
case "Sydney Opera House": 33.8587; 151.2140
default: 0; 0
}
return location
}
1 Answer

Anjali Pasupathy
28,883 PointsWith the way you've structured your solution, you're returning the String location. What you need to return are the coordinates (which should be structured as (Double, Double), not Double; Double).
One way you could do this is by writing all the case blocks and the default blocks as return statements. Another way to do this would be to create a coordinates variable of type (Double, Double) before the switch statement, assign the coordinate values to coordinates inside each case block and the default block, and return coordinates.
func getTowerCoordinates (location: String) -> (Double, Double) {
var coordinates: (Double, Double) // DECLARE coordinates VARIABLE OF TYPE (Double, Double)
switch location { // ASSIGN LONGITUDE AND LATITUDE TO coordinates IN (Double, Double) FORMAT
case "Eiffel Tower": coordinates = (48.8582, 2.2945)
case "Great Pyramid": coordinates = (29.9792, 31.1344)
case "Sydney Opera House": coordinates = (33.8587, 151.2140)
default: coordinates = (0, 0)
}
return coordinates // RETURN coordinates RATHER THAN location
}
I hope this helps!
Greg Stone
2,705 PointsGreg Stone
2,705 PointsInteresting. What you're having me do isn't quite something I've learned yet in this course -and some of your steps are opposed to the teachers instructions. Though I'm sure your code works in the real world, there must be another way to solve this as your code is not passing my code challenge.
Anjali Pasupathy
28,883 PointsAnjali Pasupathy
28,883 PointsThat is strange. I tested my code in the quiz challenge, and it passed.
I'm pretty sure what I'm having you do is well within the bounds of what you should have learned if you're on the Swift 2 track. I apologize if anything in my explanation confused you.
The relevant instructions in the challenge are as follows:
"Declare a function named getTowerCoordinates that takes a single parameter of type String, named location, and returns a tuple containing two Double values (Note: You do not have to name the return values)
Use a switch statement to switch on the string passed in to return the right set of coordinate values for the location."
The only difference between the code I wrote and the specifications in the instructions is, rather than return the coordinates ("a tuple containing two Double values", which is structured as (Double, Double)), I created a variable named coordinates of type (Double, Double), set coordinates to the correct value using the switch statement, and returned coordinates.
If you like, I could restructure the function and point out which parts of the code conform to which parts of the instructions.
I hope this clarifies the issue.