Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial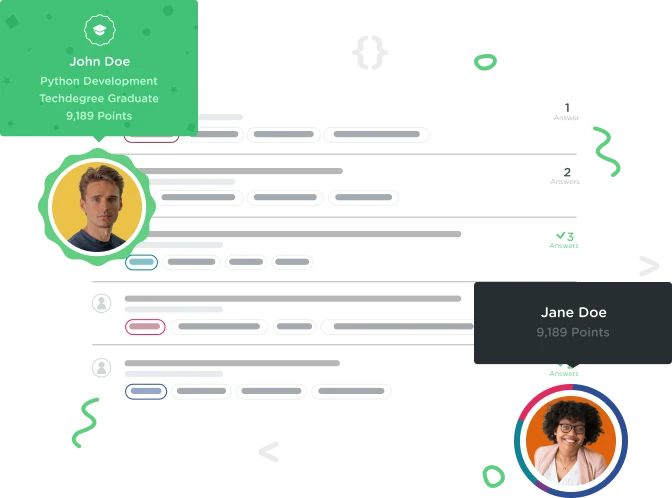
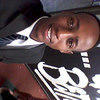
Kudakwashe Clinton Nyamhuka
3,461 PointsStuck here dont know whats wrong with my code seems alright to me. Can someone help?
In the ParseWeatherForecast method in the Program.cs file, use int.TryParse to parse the Temperature property from the value of values[3] and add the parsed value to the weatherForecast object. Don't forget to declare the variable to hold the out parameter passed to the TryParse method.
using System;
using System.IO;
namespace Treehouse.CodeChallenges
{
public class Program
{
public static void Main(string[] arg)
{
}
public static WeatherForecast ParseWeatherForecast(string[] values)
{
var weatherForecast = new WeatherForecast();
weatherForecast.WeatherStationId = values[0];
DateTime timeOfDay;
if (DateTime.TryParse(values[1], out timeOfDay))
{
weatherForecast.TimeOfDay = timeOfDay;
}
Condition condition;
if (Enum.TryParse(values[2], out condition))
{
weatherForecast.Condition = condition;
}
return weatherForecast;
int temperature;
if(int.TryParse(values[3], out temperature) )
if(int.TryParse(values[3], out temperature) )
{
weatherForecast.Temperature = temperature;
}
}
}
}
using System;
/* Sample CSV Data
weather_station_id,time_of_day,condition,temperature,precipitation_chance,precipitation_amount
HGKL8Q,06/11/2016 0:00,Rain,53,0.3,0.03
HGKL8Q,06/11/2016 6:00,Cloudy,56,0.08,0.01
HGKL8Q,06/11/2016 12:00,PartlyCloudy,70,0,0
HGKL8Q,06/11/2016 18:00,Sunny,76,0,0
HGKL8Q,06/11/2016 19:00,Clear,74,0,0
*/
namespace Treehouse.CodeChallenges
{
public class WeatherForecast
{
public string WeatherStationId { get; set; }
public DateTime TimeOfDay { get; set; }
public Condition Condition { get; set; }
public int Temperature {get; set; }
}
public enum Condition
{
Rain,
Cloudy,
PartlyCloudy,
PartlySunny,
Sunny,
Clear
}
}
4 Answers
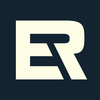
Eric Ridolfi
19,417 PointsRemove the IF condition right under int temperature and move the entire if block above the return. Once return runs, nothing will get run below that.
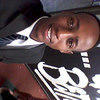
Kudakwashe Clinton Nyamhuka
3,461 PointsThanks Eric it worked. using System; using System.IO;
namespace Treehouse.CodeChallenges { public class Program { public static void Main(string[] arg) { }
public static WeatherForecast ParseWeatherForecast(string[] values)
{
var weatherForecast = new WeatherForecast();
weatherForecast.WeatherStationId = values[0];
DateTime timeOfDay;
if (DateTime.TryParse(values[1], out timeOfDay))
{
weatherForecast.TimeOfDay = timeOfDay;
}
int temperature;
if (int.TryParse(values[3], out temperature))
{
weatherForecast.Temperature = temperature;
}
return weatherForecast;
double precipitationChance;
if (double.TryParse(values[4], out precipitationChance))
{
weatherForecast.PrecipitationChance = precipitationChance;
}
return weatherForecast;
double precipitationAmount;
if (double.TryParse(values[5], out precipitationAmount))
{
weatherForecast.PrecipitationAmount = precipitationAmount;
}
return weatherForecast;
}
}
}
Now on this one its saying property precipitationChance not assigned. Can you help?
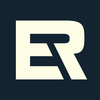
Eric Ridolfi
19,417 PointsYou want return weatherForecast last. It's in there several times so it is stopping at the first one.
So you would need to remove all of the return weatherForecast except for the one that comes after the precitptationAmount IF statement.
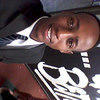
Kudakwashe Clinton Nyamhuka
3,461 Pointswow you are such a lifesaver. Have to read and practise on the return keyword. Thanks Again
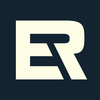
Eric Ridolfi
19,417 PointsNo problem, I'm glad I could help!