Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial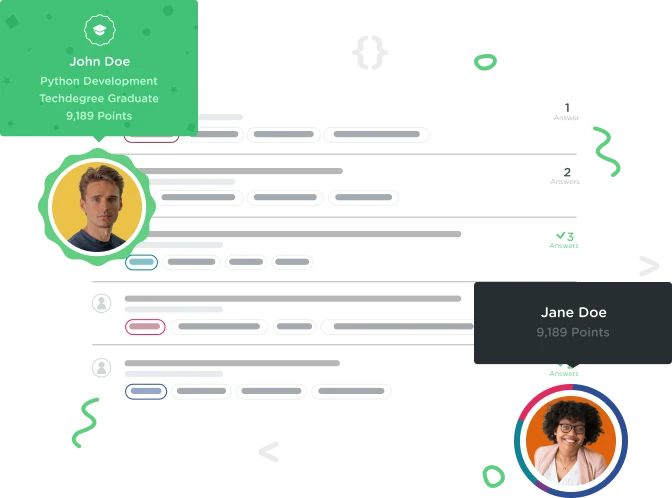

YANG CHEN
1,744 PointsStuck in the last Challenge..
Im stuck in this challenge..Can someone give me a hint of how to "take the first two elements passed args" & "Add the author, title and description". Thanks!
public class ForumPost {
private User mAuthor;
private String mTitle;
private String mDescription;
public ForumPost(User author, String title, String description){
mAuthor = author;
mTitle = title;
mDescription = description;
}
public User getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getDescription(){
return mDescription;
}
// TODO: We need to expose the description
}
public class User {
private String mFirstName;
private String mLastName;
public User(String firstName, String lastName) {
// TODO: Set the private fields here
mFirstName = firstName;
mLastName = lastName;
}
public String getFirstName(){
return mFirstName;
}
public String getLastName(){
return mLastName;
}
}
public class Forum {
private String mTopic;
public Forum(String topic){
mTopic = topic;
}
public String getTopic() {
return mTopic;
}
public void addPost(ForumPost post) {
System.out.printf("New post from %s %s about %s.\n",
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
post.getTitle());
}
}
public class Example {
public static void main(String[] args) {
System.out.println("Starting forum example...");
if (args.length < 2) {
System.out.println("first and last name are required. eg: java Example Craig Dennis");
}
else{
Forum forum = new Forum("Java");
// Take the first two elements passed args
User author = new User();
// Add the author, title and description
ForumPost post = new ForumPost();
forum.addPost(post);
}
}
}
3 Answers
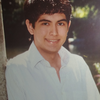
Jon Kussmann
Courses Plus Student 7,254 PointsFor the first part you'll need to look at Example.java
If you remember from the video, you can add parameters when you run the program from the console. These will be added to a string array (String[]) called args.
Arrays are zero indexed, so to get the first item from this array would be args[0].
Is that enough to get you started?

James Simshaw
28,738 PointsHello,
Args are passed into the main function as the String array args, which works just as any other array for calling them, the first arg would be args[0] and so on.

YANG CHEN
1,744 PointsWould this work? (Take the first two elements passed args) Forum forum = new Forum(args[0], args[1]); Also I'm confused about how to "Add the author, title and description". Does it mean to create a function that would enable us to add the author, title and description in the post? What method should I use?
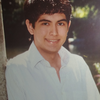
Jon Kussmann
Courses Plus Student 7,254 PointsThe first two arguments that will be passed in will be a user's first name and last name, respectively. So instead of creating a Forum object with those, you should create a User object.
For example:
User author = new User(args[0], args[1]);
The next step involves creating a ForumPost object, and that takes three parameters... an author, title and description. The author parameter would just be the User object that you have created. You don't need a new method to add the author, etc.... do so when you construct the ForumPost object like above with creating the User object.
YANG CHEN
1,744 PointsYANG CHEN
1,744 PointsThank you Jon! You hints are very helpful and I finally figure out how to do the task.
Jon Kussmann
Courses Plus Student 7,254 PointsJon Kussmann
Courses Plus Student 7,254 PointsGlad to hear!