Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial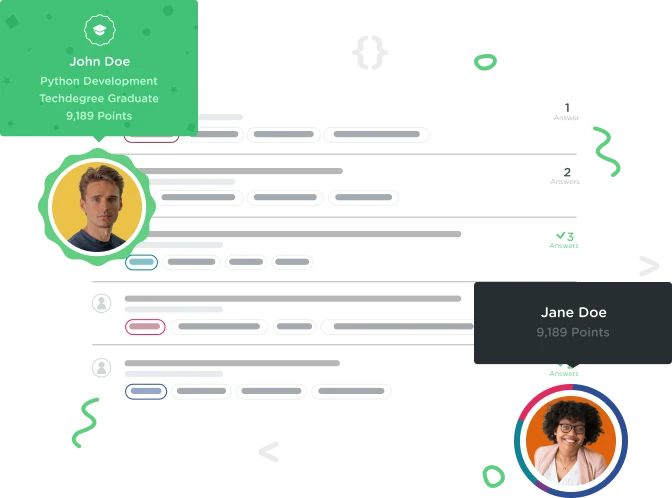
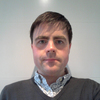
James Pask
13,600 PointsStuck on challenge 3 of the "Methods and Constants challenge" on Java Objects..
On this part:
Finally, let's add a method named charge. It should be public and return nothing. When called it should set mBarsCount to the value of the constant you declared in Task 1.
I've attempted to add the part about it returning nothing and I think that "void" should be used somewhere but I can't get it to work. I'm having problems converting the string to an integer. Considered using the Interger.parseInt thing but couldn't get that to do anything sensible either - maybe I was just making up code?
Can someone point me in the right direction please?
public class GoKart {
private String mColor;
public static final int MAX_CHARGE = 8;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
public String charge;
return mBarsCount;
}
}
2 Answers
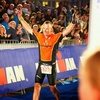
Steve Hunter
57,712 PointsOK - step by step:
Question: add a method named charge. It should be public and return nothing.
That looks like:
public void charge(){
}
Next up is the body of the method: When called it should set mBarsCount to the value of the constant you declared in Task 1.
public void charge(){
mBarsCount = MAX_CHARGE;
}
You have the method declaration returning a String and then the method body returning the mBarsCount
integer; it doesn't need to return anything. You also need to make sure that the method is declared with brackets after the name - they are empty as the method takes no parameters, but they are needed. And then you need to open up the body with a curly brace, and close that after the method.
Hope that makes sense!
Steve.
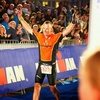
Steve Hunter
57,712 PointsHi James,
I'm pretty sure you've got that, yes. There's some further learning to be done as the course progresses but you're pretty much on track.
A couple of points: "Methods are applied to the variables of the class" - methods can do anything they like. Very often, they manipulate the instance variables as you have pointed out. You'll do more with "getters and setters" as the course progresses which will reinforce what you've understood so far. Next, " "constructors" apply to the class itself" - not quite. A constructor creates the instance of the class. It can take parameters to build the instance, in this case the colour of the Kart object. So, the constructor is the code that is applied to all instances of the class to create those instances and enable to user to specify any unique features, color in this case.
I've added some alternative comments in response to your request too.
Hope that helps!
Steve.
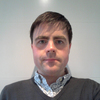
James Pask
13,600 PointsAppreciate your comments - thanks very much :)
James Pask
13,600 PointsJames Pask
13,600 PointsI think it's starting to make sense. So, this code:
is a method. And the code that we added in task 3 is also a method. Methods are applied to the variables of the class whereas "constructors" apply to the class itself, right?
Here is my finished code (which was accepted by the challenge (excluding my comments which I've added to help with my learning)).
If someone could also double check to ensure that my comments are correct, it'd be appreciated.