Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial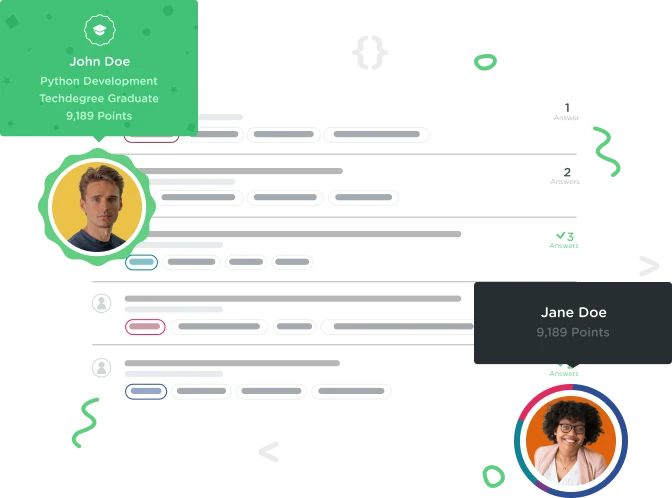
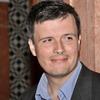
Brian Patterson
19,588 PointsStuck on Challenge
Here is my solution to the challenge- But it is giving me an error not returning tuples
func getTowerCoordinates(location: String) -> (lat: Double, long: Double){
switch location {
case "Eiffel Tower" : (48.8582,2.2945)
case "Great Pyramid": (29.9792,31.1344)
case "Sydney Opera House": (33.8587,151.2140)
default: (0,0)
}
return (lat:Double, long: Double)
}
3 Answers
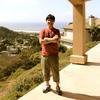
Richard Lu
20,185 PointsHey Brian,
It seems that your solution is missing one key component which is actually return the values. I've modified the code.
func getTowerCoordinates(location: String) -> (lat: Double, long: Double) {
switch location {
case "Eiffel Tower" : return (48.8582,2.2945) // return the value
case "Great Pyramid": return (29.9792,31.1344) // return the value
case "Sydney Opera House": return (33.8587,151.2140) // return the value
default: return (0,0) // return the value
}
}
The last line was also erased since you have a default value of (0,0)
good luck! :)

Alia Khan
3,733 PointsHi just a quick question How did you know to place the return keyword after naming the values.
Whilst I didn't name the tuple and did everything else right I like Brian put the return keyword at the end. Could anyone explain this?
Thank you
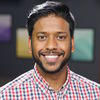
Pasan Premaratne
Treehouse TeacherHey Alia Khan
You can think of each case in a switch statement as a path of code through the function. If you hit a particular case, only the code in that case is executed. In this line of code:
case "Eiffel Tower" : (48.8582,2.2945)
You're not doing anything with the tuple that you create. It's created in that particular case, but you're not storing it in a variable or constant. So the moment you are done with the case, those values cease to exist.
Now if in each case you stored it in a variable that you declare at the top of the function and assign the tuple to that variable you can use it at the end to return out of the function.
Basically if you see curly braces in code, what is created within curly braces doesn't exist outside out of it unless you assign it to a constant or variable declared outside of it. The switch statement starts a new set of curly braces; anything created inside the switch statement exists within those braces only. This concept is called scope.
So yeah the reason you can't return at the end in your code is you're not returning any values.

victorripley
5,209 Pointsfunc coordinates (for location: String) -> (double double) { switch location { case "Eiffel Tower": return (48.8582, 2.2945) case " Great Pyramid": return(29.9792, 31.1344) case "Syndey Opera House" return (33.8587, 151.2140) default = return (0,0) } return (0,0, 0,0)
}
It keeps telling me that Your function needs to return a tuple containing two Double values"---what am I doing wrong
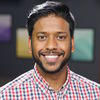
Pasan Premaratne
Treehouse TeacherHey victorripley
(1) This is the older Swift 2 version of the course. There's a newer, Swift 3 version. From your code it seems like you're using Swift 3 version but just want to double check because you're posting in Swift 2 course section.
(2) The reason you're getting this error is because your function is returning (double double)
. For staters, double, with lower case D is not an actual type. Second, you need to comma separate values in a tuple
Brian Patterson
19,588 PointsBrian Patterson
19,588 Points"Your function needs to return a tuple containing two Double values " Getting the above error with this code! How do you correct this?
Brian Patterson
19,588 PointsBrian Patterson
19,588 PointsYes I am getting the error with your code.
Richard Lu
20,185 PointsRichard Lu
20,185 PointsTry this out.
He mentions that you do not have to name the tuple return types.
Pasan Premaratne
Treehouse TeacherPasan Premaratne
Treehouse TeacherRichard Lu and Brian Patterson,
It's not a bug. The directions specify not to name the return values
(Note: You do not have to name the return values)
In Swift functions have signatures so the function Brian has above has a signature of
String -> (lat: Double, long: Double)
If you don't name the parameters , the signature is
String -> (Double, Double)
While this seems trivial, it means that I can't really validate answers that return named tuples because then I have to required everyone to pass in named tuples. It's an either or situation. If everyone returns named tuples then I have to restrict the names you can use because as you can see from the function, the names are hard coded into the resulting signature and I have to check you're passing in the correct names.
Long story short, since Swift is type safe, minor things like this make the underlying code quite different
Brian Patterson
19,588 PointsBrian Patterson
19,588 PointsThank you for the reply. I understand now. Really enjoying the course and I appreciate the speedy reply.