Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial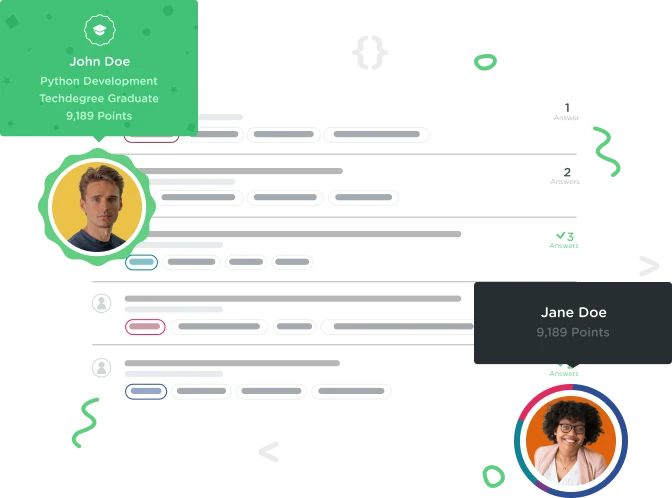

Michael Kolibaba
915 Pointsstuck on challenge to create new function named “max” (to compare 2 numbers)
I have become stuck with this challenge, even after reviewing posts from others seeking help.
My guess is that my conditional statement is incorrectly formatted (and possibly other errors as well). Could someone please look at my code and help me find my mistakes?
Here is my code:
function max (numberA, numberB){
if (numberA < numberB){
return numberA
else
return numberB
}
return max (5,8);
function max (numberA, numberB){
if (numberA < numberB){
return numberA
else
return numberB
}
return max (5,8);
3 Answers

Alexander Solberg
14,350 Points1) You are using "return" to call the function, which ufortunately is incorrect usage of "return". "return" is used to "send back" a value from the function. If you want to use a function, you need to call it with parenthesis. so:
max(5, 8)
2) your "else" statement is missing its braces, {}
Other than that, it looks fine :)
function max(numberA, numberB) {
if (numberA < numberB) {
return numberA;
} else {
return numberB;
}
}
max(5,8)

Antonio De Rose
20,885 Points/*2 issues
issue number 1 -> if and else, where are the proper curly construction
there are two opening curly to one closing curly, in your code
there should be three opening curly to three closing curly, altogether considering the function
issue number 2 -> check, are you returning the smaller or the larger number*/
function max (numberA, numberB){
if (numberA < numberB){
return numberA
else
return numberB
}
return max (5,8); // why this statement, take it off, for the first task

Michael Kolibaba
915 PointsHi Antonio,
Thanks for your notes and suggestions for fixing the errors in my code. They are very helpful!

Michael Kolibaba
915 PointsHi Alexander,
Thanks for reviewing my code and for your suggestions, which are great. When I tried submitting this code, I got an error message telling me the smaller number was being returned (sorry, but I didn't think to copy the error message).
I changed the code to read as follows:
function max(numberA, numberB) {
if (numberA === numberB) {
return numberA;
} else {
return numberB;
}
}
max(5,8)
Fortunately, this change did the trick :). Thanks for your help!

Alexander Solberg
14,350 PointsAh I see where it went wrong. I am using the wrong comparison operator, "<", instead of ">".
I suggest sticking to ">" rather than "===". Because with the triple equals, you are checking if numberA is equal to numberB, which for some reason passes the challenge :P but it's not actually doing what was asked in the challenge.