Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial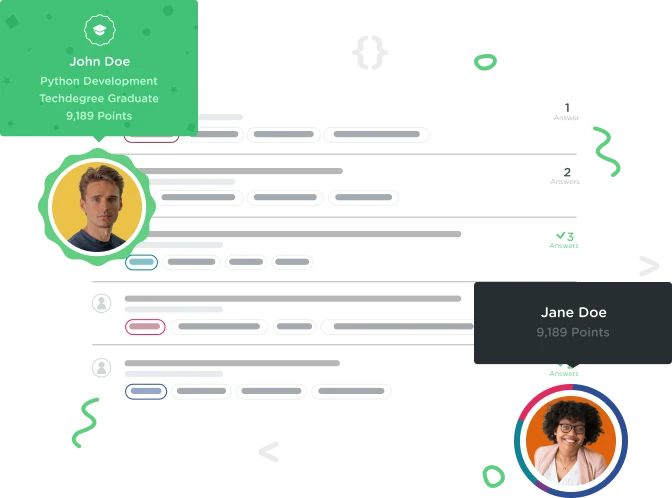
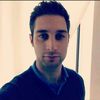
Giancarlo Fusiello
9,795 PointsStuck on final Django Basics Challenge
Hi all,
keep getting the following error when testing my code. Can someone enlighten me as to what I'm missing?
AssertionError: False is not true : Couldn't find 'I Wanna Be Sedated by The Ramones' in response
It appears the performer is not being associated with the assigned songs.
The instructions do state that the performer_detail view should:
- performer view, a particular performer
- list all of their songs
I believe I'm not listing the songs but cannot figure out how to add them in the response. Any help would be appreciated.
Thanks,
from django.db import models
# Write your models here
class Performer(models.Model):
name = models.CharField(max_length=255)
def __str__(self):
return self.name
class Song(models.Model):
title = models.CharField(max_length=255)
artist = models.CharField(max_length=255)
performer = models.ForeignKey(Performer)
length = models.IntegerField(default=0)
def __str__(self):
return '{} by {}'.format(self.title, self.artist)
from django.shortcuts import get_object_or_404, get_list_or_404, render
from .models import Song, Performer
def song_list(request):
songs = Song.objects.all()
return render(request, 'songs/song_list.html', {'songs': songs})
def song_detail(request, pk):
song = get_object_or_404(Song, pk=pk)
return render(request, 'songs/song_detail.html', {'song': song})
def performer_detail(request, pk):
performer = get_object_or_404(Performer, pk=pk)
return render(request, 'songs/performer_detail.html', {'performer': performer})
{% extends 'base.html' %}
{% block title %}{{ performer }}{% endblock %}
{% block content %}
<h2>{{ performer }}</h2>
{% endblock %}
4 Answers
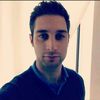
Giancarlo Fusiello
9,795 PointsI eventually figured it out.
My performer_detail function was missing this:
performer.song_set.all()
more information can be found here:
If a model has a ForeignKey, instances of the foreign-key model will have access to a Manager that returns all instances of the first model. By default, this Manager is named FOO_set, where FOO is the source model name, lowercased. This Manager returns QuerySets, which can be filtered and manipulated as described in the “Retrieving objects” section above.
In context it looks like this:
def performer_detail(request, pk):
performer = get_object_or_404(Performer, pk=pk)
performer.song_set.all()
return render(request, 'songs/performer_detail.html', {'performer': performer})
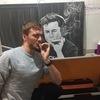
Tobias Mahnert
89,414 Pointsbesides the changes what giancarlo mentioned, update the template code like that and i passes
{% extends 'base.html' %}
{% block title %}{{ performer }}{% endblock %}
{% block content %} <h2>{{ self.performer }}</h2> <ul> {% for song in performer.song_set.all %} <li>{{ song }}</li> {% endfor %} </ul> {% endblock %}
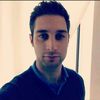
Giancarlo Fusiello
9,795 PointsDid you replace the performer detail function with that provided in the answer?
What is the assertion error you're seeing?

Ira Bradley
12,976 PointsThe performer_detail view should: * return a 200 * have self.performer in the context * use the songs/performer_detail.html template * show the string version of self.song in the template
You need to modify the template so that self.song is in the template.
Chase Marchione
155,055 PointsChase Marchione
155,055 PointsInterestingly, I tried testing your code on my end and not all of the views's tests seem to pass. Your solution looks good, though.